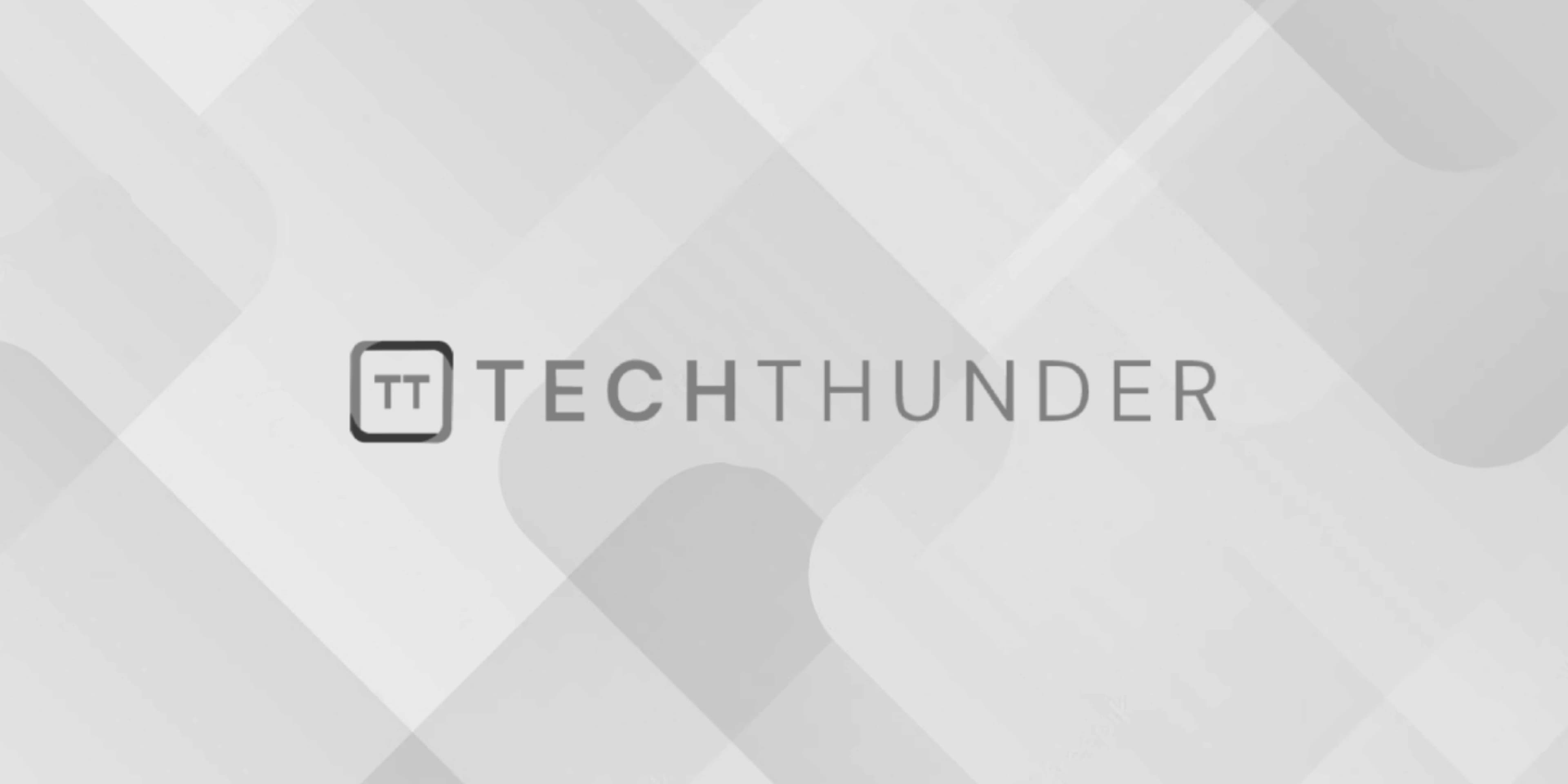
116 views
Virtual functions and runtime polymorphism in C++
Virtual functions and runtime polymorphism are powerful features of object-oriented programming (OOP) in C++. They allow you to achieve dynamic behavior based on the actual type of objects at runtime.
Virtual Functions:
- A virtual function is a member function of a base class that is declared with the
virtual
keyword. It is intended to be overridden by derived classes. - Virtual functions allow a derived class to provide a specific implementation for a function defined in a base class, even if the derived class is used through a pointer or reference to the base class.
- When you call a virtual function through a pointer or reference to the base class, the actual function that gets executed is determined by the type of the object being pointed to or referenced, not the type of the pointer or reference.
Example:
C++
class Shape {
public:
virtual void draw() {
// Base class implementation
std::cout << "Drawing a shape" << std::endl;
}
};
class Circle : public Shape {
public:
void draw() override {
// Derived class implementation
std::cout << "Drawing a circle" << std::endl;
}
};
Runtime Polymorphism:
- Runtime polymorphism is the ability of a program to determine the appropriate function implementation to execute at runtime, based on the actual type of an object.
- This is achieved through the use of virtual functions and inheritance. When a base class pointer or reference is used to access a derived class object, the appropriate derived class function is called.
Example:
C++
int main() {
Shape* shapePtr = new Circle();
shapePtr->draw(); // Calls draw() of Circle
delete shapePtr;
return 0;
}
In this example, shapePtr
is a pointer to a base class (Shape
). However, it is pointing to an object of the derived class (Circle
). When draw()
is called through shapePtr
, it calls the overridden function in Circle
, demonstrating runtime polymorphism.
Important Considerations:
- Virtual functions should be used in base classes when you expect derived classes to provide their own implementation.
- Always use the
override
keyword when overriding a virtual function to ensure that you’re actually overriding a function from a base class. - It’s good practice to have a virtual destructor in the base class if you plan to delete objects through pointers to the base class.
- Virtual functions can incur a slight runtime overhead due to the virtual function table (vtable) lookup, but the benefits in terms of flexibility and code organization usually outweigh this cost.
Runtime polymorphism is a powerful mechanism that enables more flexible and extensible code. It’s particularly useful in scenarios where you have a family of related classes and want to work with them generically through a common interface.