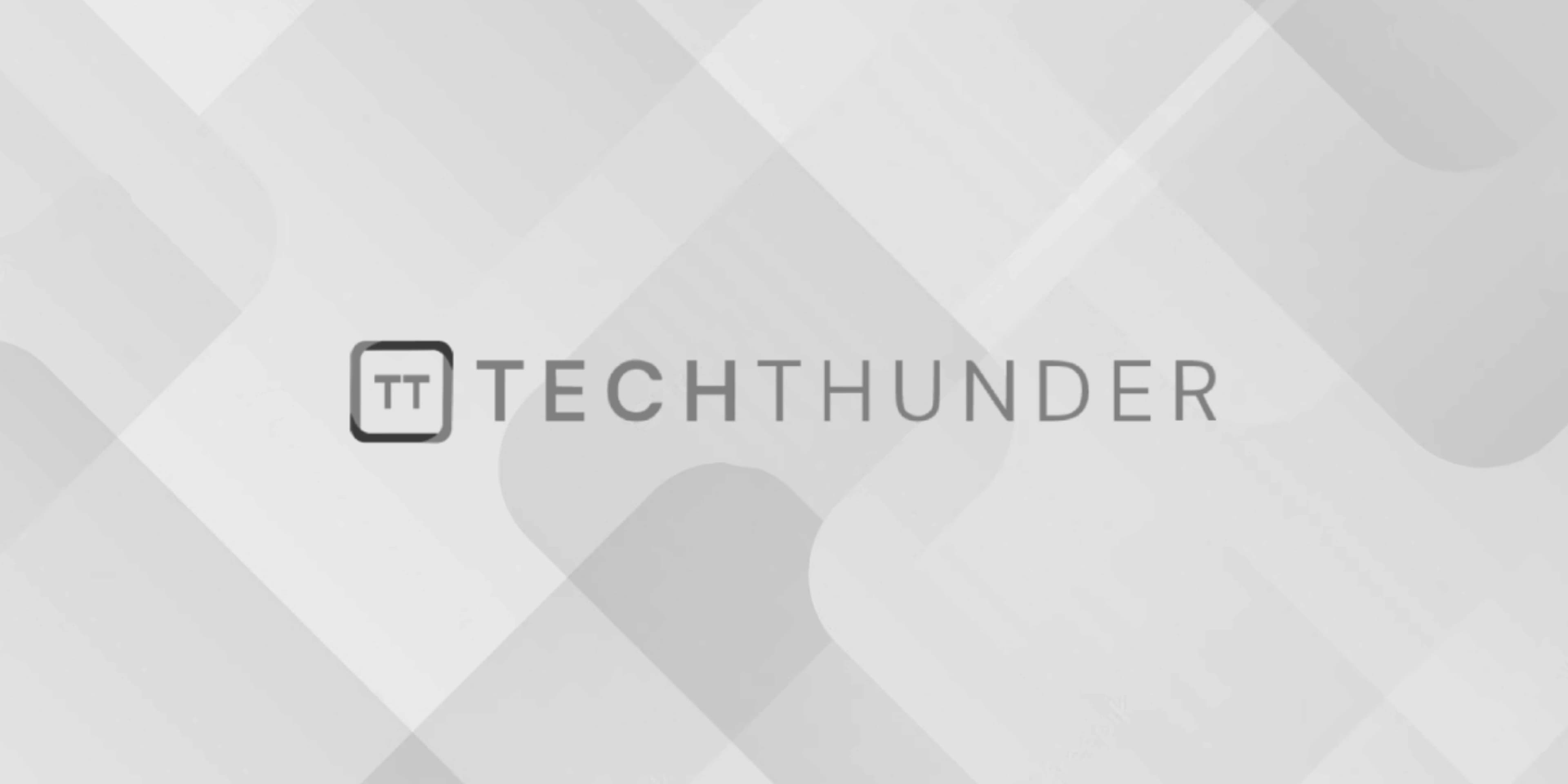
Range-based for loop in C++
A range-based for loop in C++ is a convenient way to iterate over the elements of a container or a sequence without having to deal with indices or iterators explicitly. It was introduced in C++11 and is commonly used for arrays, standard containers (like vectors and lists), and other iterable objects. Here’s the basic syntax:
for (const auto &element : iterable) {
// Do something with 'element'
}
Here’s a breakdown of the components:
const auto &element
: This is a declaration of a loop variable that will take on each element’s value in the iterable. Theauto
keyword is used to automatically deduce the type of each element, andconst
is used to indicate that you won’t modify the elements if you only intend to read them. You can omitconst
if you intend to modify the elements.: iterable
: This is the range-based for loop’s range specification. It represents the container or sequence you want to iterate over.
Here are a few examples of how to use a range-based for loop with different types of containers:
- Array:
int arr[] = {1, 2, 3, 4, 5};
for (const auto &element : arr) {
// Do something with 'element'
std::cout << element << " ";
}
- Vector (from the C++ Standard Library):
#include <iostream>
#include <vector>
std::vector<int> vec = {10, 20, 30, 40, 50};
for (const auto &element : vec) {
// Do something with 'element'
std::cout << element << " ";
}
- String:
#include <iostream>
#include <string>
std::string str = "Hello, World!";
for (const auto &ch : str) {
// Do something with 'ch'
std::cout << ch << " ";
}
- Custom Iterable Object:
#include <iostream>
#include <vector>
class MyIterable {
public:
MyIterable() : data_{1, 2, 3, 4, 5} {}
auto begin() { return data_.begin(); }
auto end() { return data_.end(); }
private:
std::vector<int> data_;
};
int main() {
MyIterable myIterable;
for (const auto &element : myIterable) {
// Do something with 'element'
std::cout << element << " ";
}
return 0;
}
In the last example, we create a custom iterable object MyIterable
that allows us to use a range-based for loop to iterate over its elements.
Remember that you can use the range-based for loop when you want to iterate through a container without modifying its elements. If you need to modify the elements or access their indices, you may still need to use a traditional for
loop.