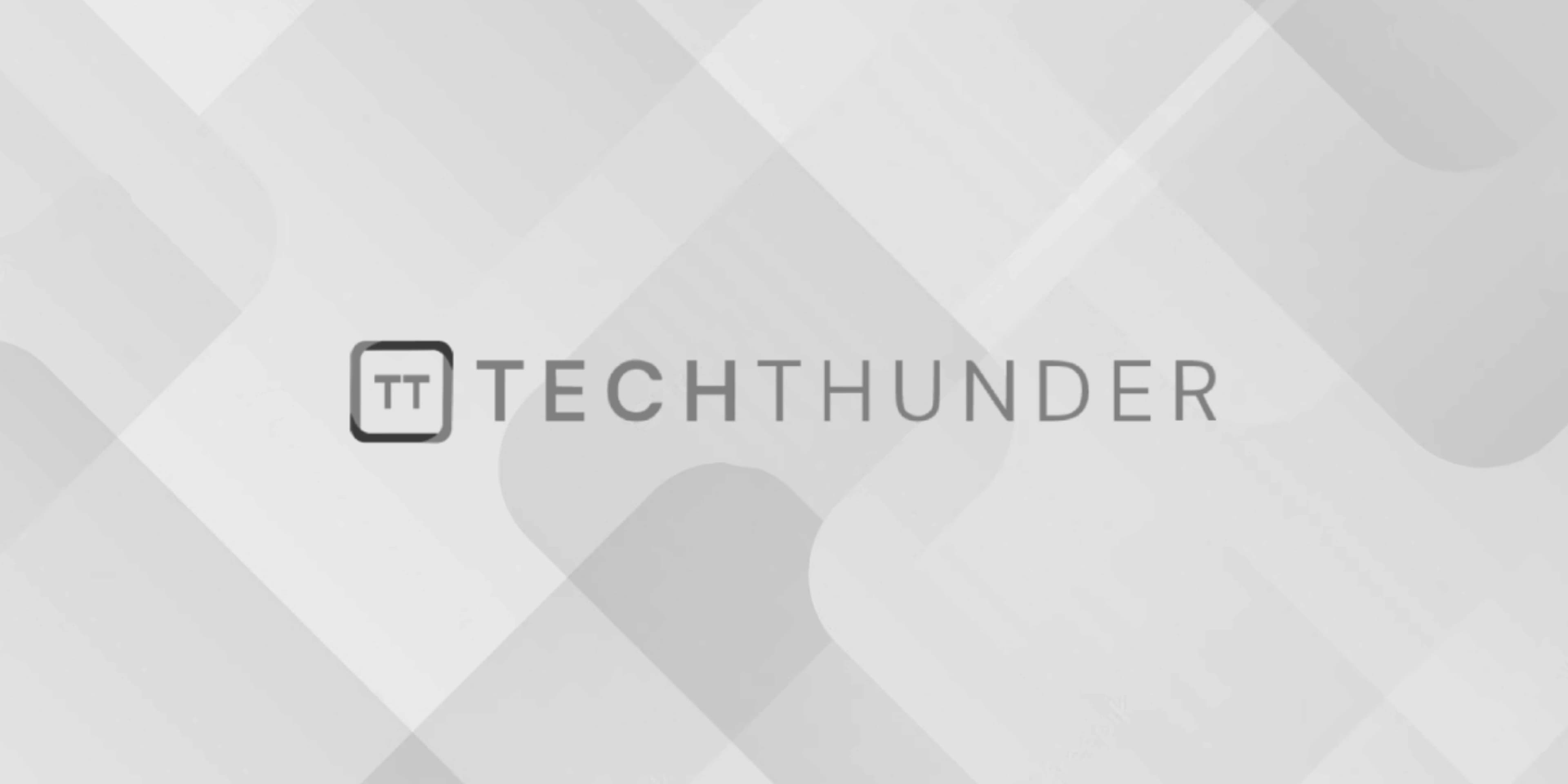
Memset in C++
The C++ memset
function is used to set a block of memory to a specific value. It is part of the C standard library and is commonly used for initializing arrays or buffers to a particular value, often zero. Here’s how you can use memset
in C++:
#include <iostream>
#include <cstring>
int main() {
char buffer[10];
// Using memset to initialize the buffer to zero
std::memset(buffer, 0, sizeof(buffer));
// Printing the contents of the buffer
for (int i = 0; i < sizeof(buffer); i++) {
std::cout << static_cast<int>(buffer[i]) << " ";
}
return 0;
}
In this example:
- We include the
<cstring>
header to access thememset
function. - We create a character array
buffer
of size 10. - We use
memset
to set the entirebuffer
to zero. Thememset
function takes three arguments: - The first argument is a pointer to the memory location to be set.
- The second argument is the value (in this case, zero) that will be written to the memory.
- The third argument is the number of bytes to set to the specified value. In this case, we use
sizeof(buffer)
to specify the size of thebuffer
. - Finally, we print the contents of the
buffer
, which will display ten zeros.
memset
is a low-level memory manipulation function, and it works with bytes. It’s often used for quickly initializing memory to a known state, like zeroing out an array or setting specific bit patterns in a memory block. Be cautious when using memset
with non-char types, as it may not produce the expected results when setting values other than zero. For complex objects with constructors and destructors, it’s typically better to use C++ constructs like constructors and assignment operators for initialization.