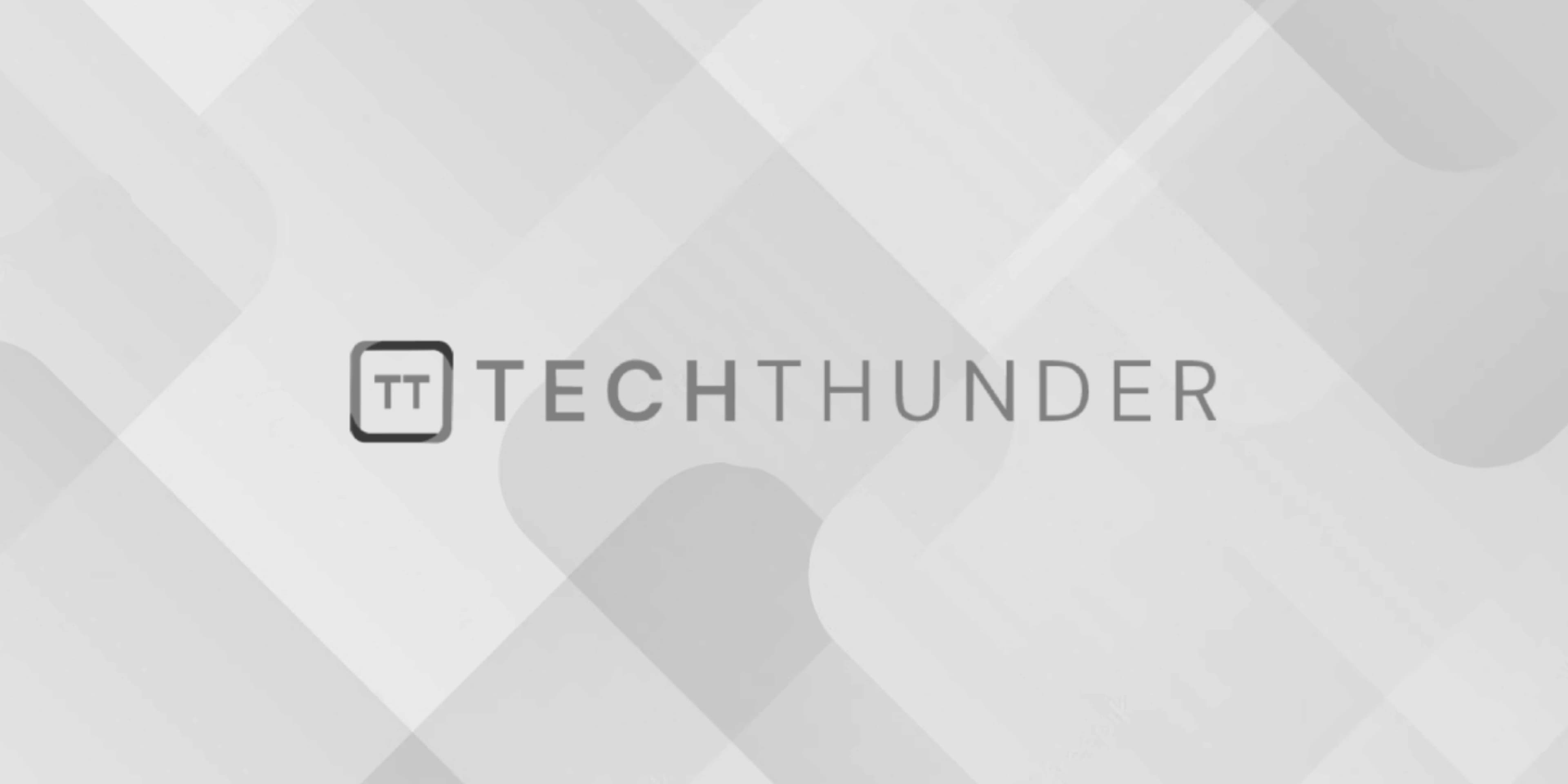
STRCMP() IN C++
The strcmp()
function is not a part of standard C++, but it is a standard C library function used for comparing two null-terminated C-style strings. It is commonly used when working with C-style strings (character arrays) in C++.
Here’s how to use strcmp()
:
#include <iostream>
#include <cstring> // Include the C string library for strcmp
int main() {
const char* str1 = "hello";
const char* str2 = "world";
int result = strcmp(str1, str2);
if (result < 0) {
std::cout << "str1 comes before str2" << std::endl;
} else if (result > 0) {
std::cout << "str2 comes before str1" << std::endl;
} else {
std::cout << "str1 and str2 are equal" << std::endl;
}
return 0;
}
In this example:
- We include the
<cstring>
header to use thestrcmp()
function. - We define two C-style strings
str1
andstr2
. - We call
strcmp(str1, str2)
to compare the two strings. - The function returns an integer value:
- If
result
is less than0
, it meansstr1
comes beforestr2
. - If
result
is greater than0
, it meansstr2
comes beforestr1
. - If
result
is0
, it means the two strings are equal.
Keep in mind that strcmp()
compares strings lexicographically, character by character, and stops when it finds a difference or reaches the end of one of the strings. It does not compare the lengths of the strings, so if you have strings of different lengths, you should be careful to avoid buffer overflows. In modern C++, it’s often recommended to use std::string
for string manipulation instead of C-style strings and functions like strcmp()
for improved safety and ease of use.