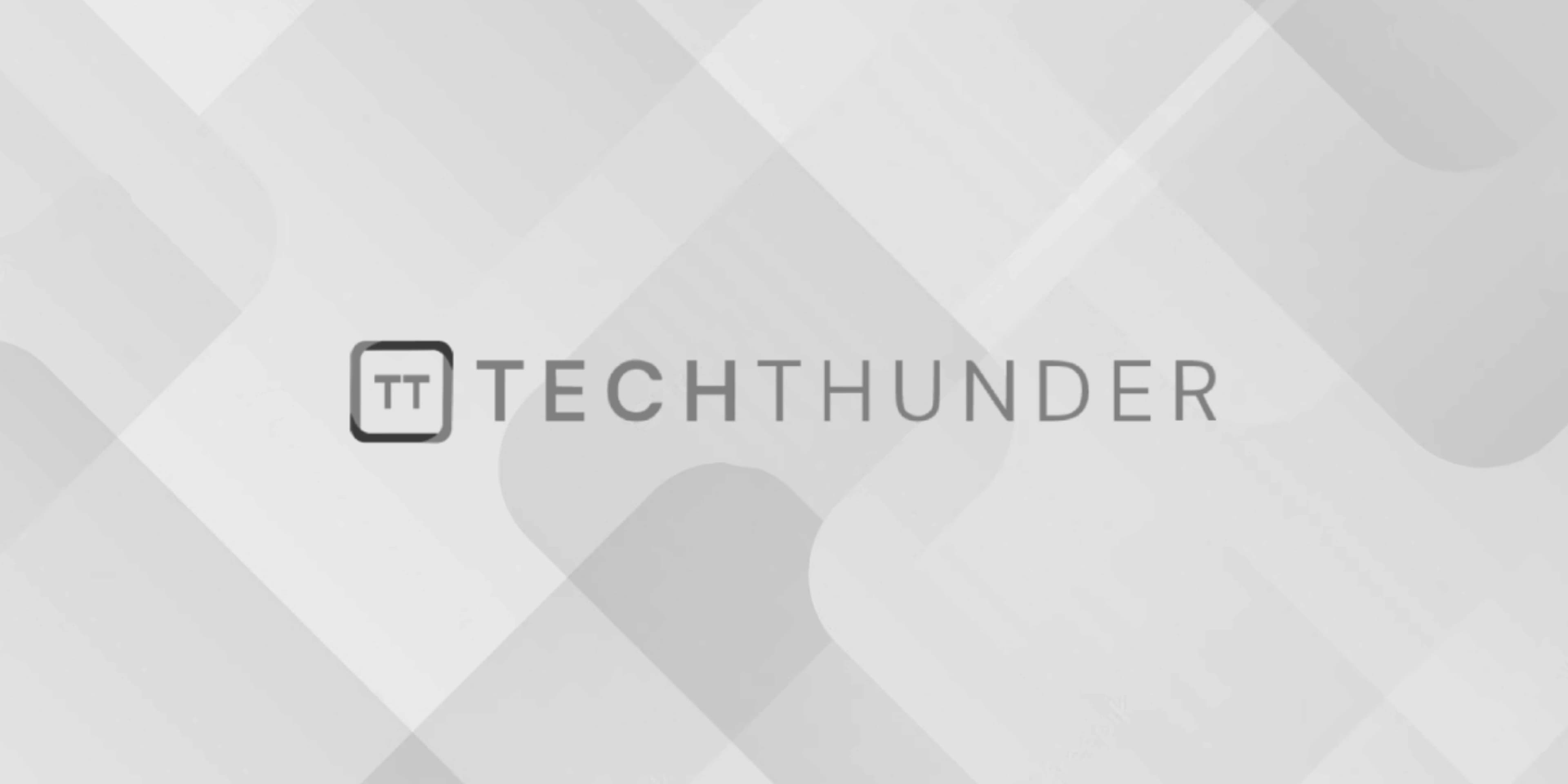
239 views
Isprint() in C++
The C++ isprint()
function is used to determine if a given character is a printable character. A printable character is any character that is not a control character (such as newline, tab, or carriage return) and can be displayed or printed to the screen.
Here’s how you can use the isprint()
function in C++:
C++
#include <iostream>
#include <cctype> // Include the <cctype> header for isprint() function
int main() {
char ch = 'A';
if (isprint(ch)) {
std::cout << ch << " is a printable character." << std::endl;
} else {
std::cout << ch << " is not a printable character." << std::endl;
}
ch = '\n';
if (isprint(ch)) {
std::cout << ch << " is a printable character." << std::endl;
} else {
std::cout << ch << " is not a printable character." << std::endl;
}
return 0;
}
In this example, we include the <cctype>
header to access the isprint()
function. We then use it to check if a character is printable. The isprint()
function returns a non-zero value (typically 1) if the character is printable, and 0 if it’s not.
In the example, we check if the characters ‘A’ and ‘\n’ (newline) are printable. The output will be:
A is a printable character.
[Newline] is not a printable character.
As you can see, ‘A’ is a printable character, while the newline character ‘\n’ is not.