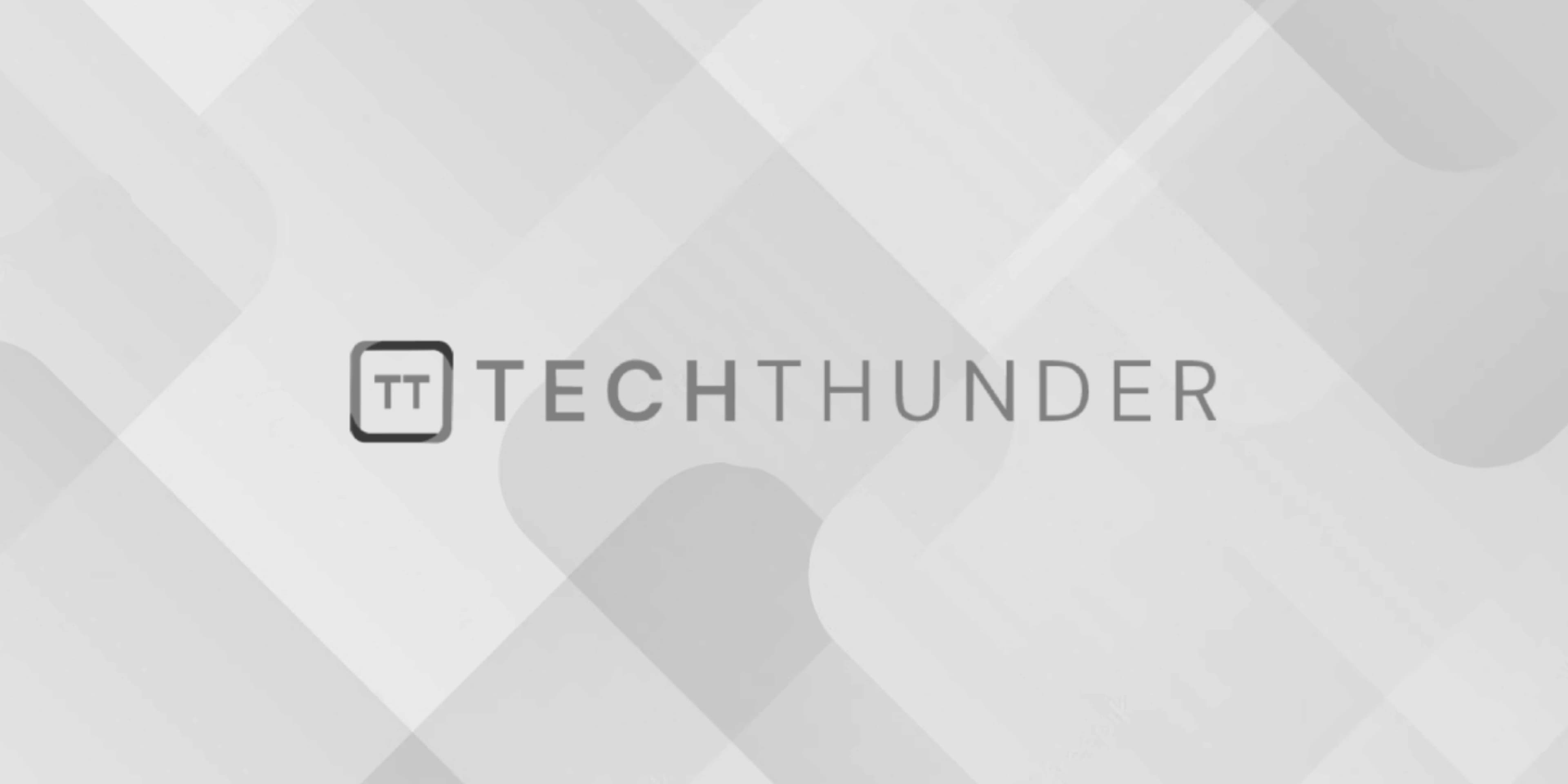
337 views
Add two Numbers in C++
You can add two numbers in C++ using the +
operator. Here’s a simple C++ program that takes two numbers as input from the user and then adds them together:
C++
#include <iostream>
int main() {
double num1, num2, sum;
// Input the first number
std::cout << "Enter the first number: ";
std::cin >> num1;
// Input the second number
std::cout << "Enter the second number: ";
std::cin >> num2;
// Add the two numbers
sum = num1 + num2;
// Display the result
std::cout << "The sum of " << num1 << " and " << num2 << " is: " << sum << std::endl;
return 0;
}
In this program:
- We include the
<iostream>
header to use input and output streams. - We declare three variables:
num1
,num2
, andsum
to store the two input numbers and their sum. - We use
std::cin
to get input from the user for bothnum1
andnum2
. - We add
num1
andnum2
together and store the result in thesum
variable. - Finally, we use
std::cout
to display the result to the user.
Compile and run this program, and it will add the two numbers you provide as input and display the sum.