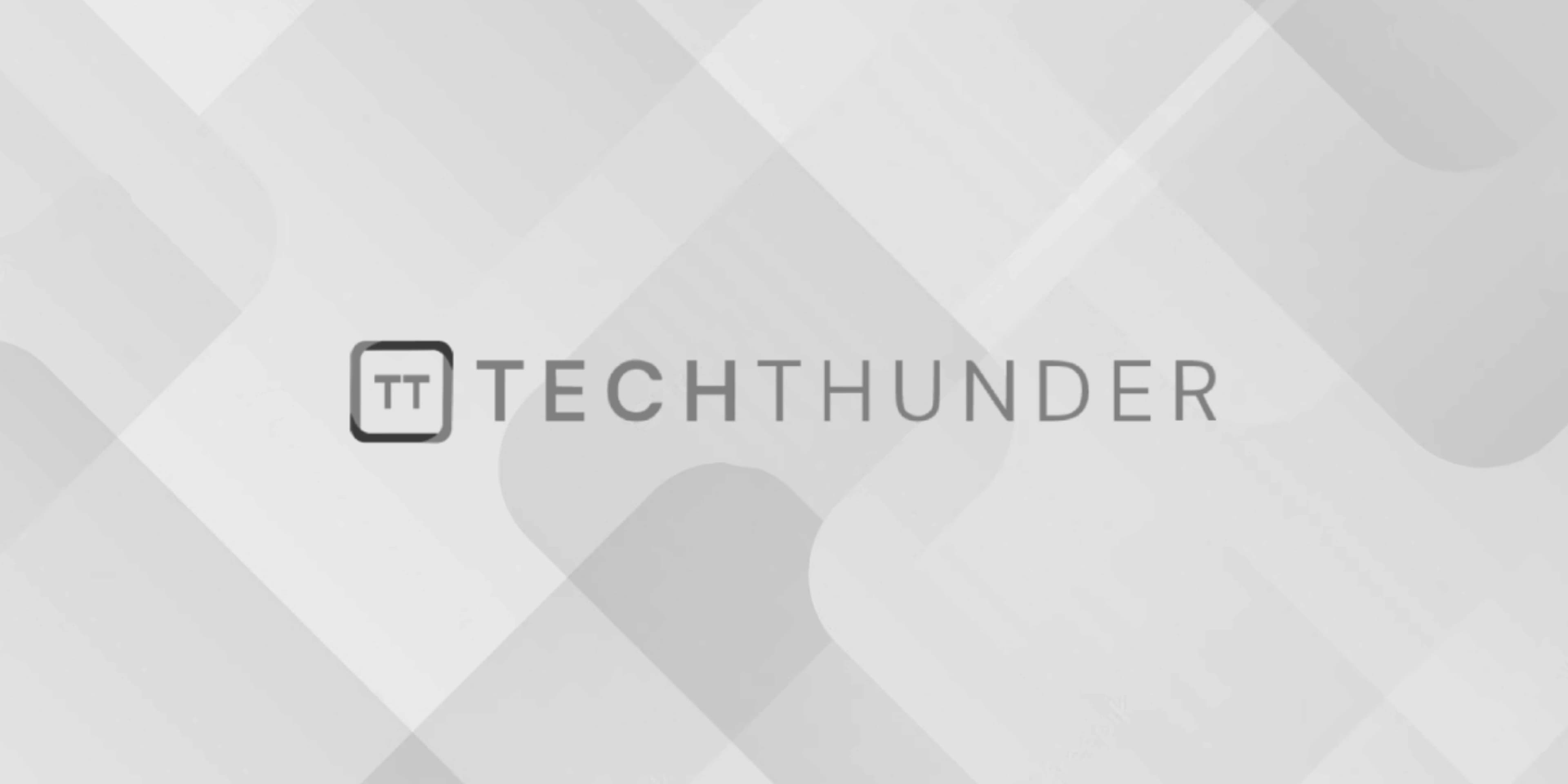
C++ static
The C++ static
keyword has multiple uses and meanings depending on the context in which it is used. Here are some common uses of the static
keyword:
- Static Variables (Static Data Members): In the context of class definitions, the
static
keyword is used to define static data members (or static variables). These variables belong to the class itself rather than to any specific instance (object) of the class. They are shared among all instances of the class.
class MyClass {
public:
static int staticVariable; // Declaration of a static variable
};
int MyClass::staticVariable = 0; // Initialization of the static variable
In this example, staticVariable
is a static data member of the MyClass
class. You can access it using the class name rather than an instance of the class: MyClass::staticVariable
.
- Static Member Functions: You can also declare static member functions in a class. These functions are associated with the class itself, rather than with instances of the class. They can be called using the class name, without the need for an object.
class MyClass {
public:
static void staticFunction() {
// Static member function code
}
};
You can call a static member function as follows: MyClass::staticFunction();
.
- Static Local Variables (Function-Level): Inside a function, the
static
keyword can be used to define static local variables. These variables retain their values between function calls, unlike regular local variables, which are reinitialized each time the function is called.
void myFunction() {
static int count = 0; // Static local variable
count++;
std::cout << "Count: " << count << std::endl;
}
In this example, count
is a static local variable, so its value persists across multiple calls to myFunction
.
- Static Global Variables: At the global (file) scope, the
static
keyword can be used to define static global variables. These variables have internal linkage, meaning they are only accessible within the file where they are declared.
static int globalStaticVariable = 42; // Static global variable
globalStaticVariable
can only be accessed within the same source file.
- Static Class Members (C++17 and later): Starting with C++17, you can use the
static
keyword in class templates to declare static data members. These are similar to static data members in regular classes but are associated with class templates.
template <typename T>
class MyTemplate {
public:
static int staticVariable;
};
template <typename T>
int MyTemplate<T>::staticVariable = 0;
Each instantiation of MyTemplate
has its own copy of staticVariable
, depending on the template argument.
The static
keyword serves various purposes in C++, such as creating shared variables, defining utility functions that don’t require object instances, and controlling variable lifetimes. Its usage varies based on the specific context in which it is used.