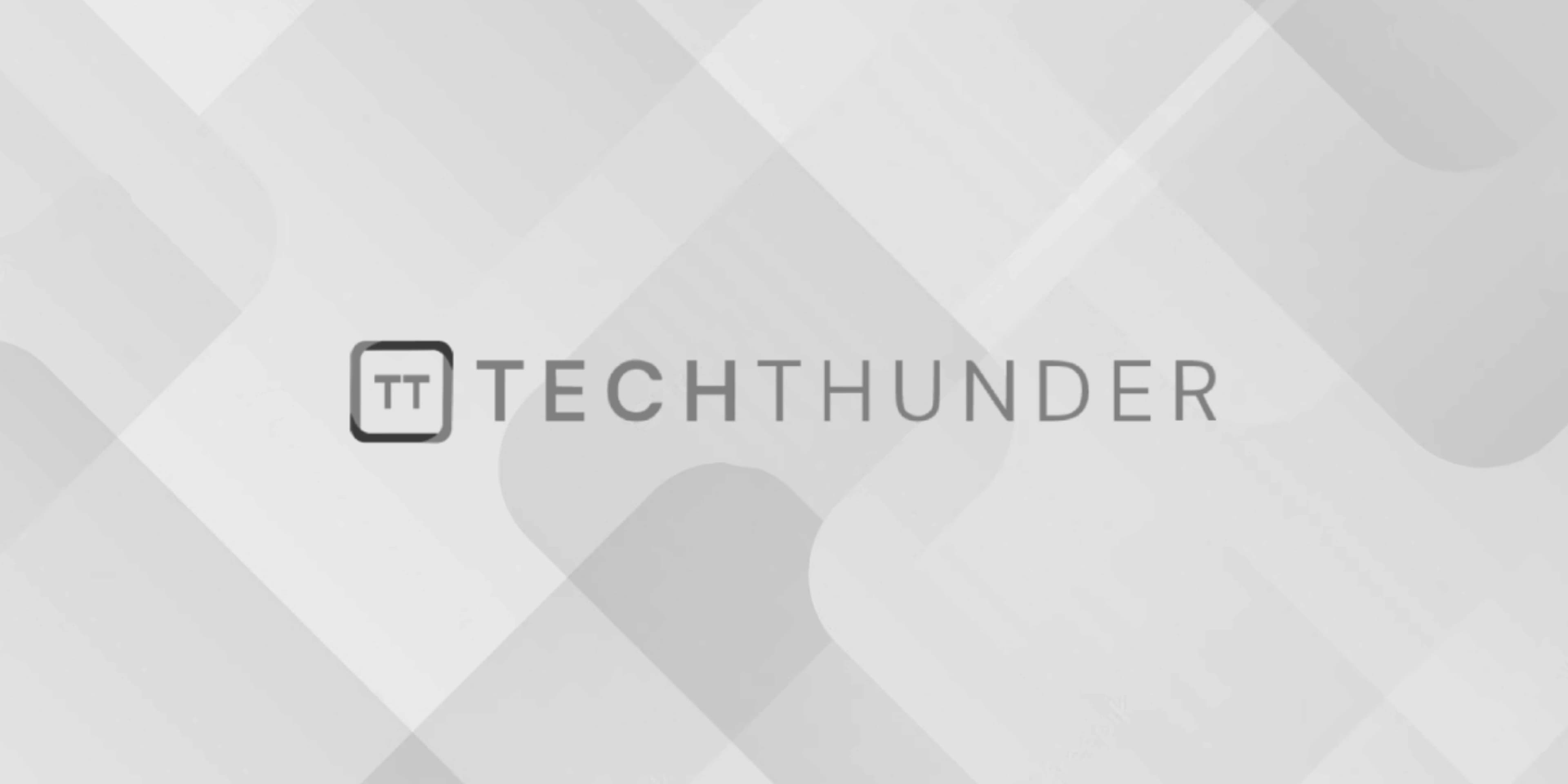
C++ Templates
C++ templates are a powerful feature that allow you to write generic code that can work with different data types or classes without knowing their specific types in advance. Templates provide a way to create reusable code and achieve type abstraction, making C++ a highly flexible and versatile language. There are two main types of templates in C++: function templates and class templates.
- Function Templates: Function templates allow you to define generic functions that can work with different data types. You define a template function by using the
template
keyword followed by thetypename
orclass
keyword, and then specify one or more template parameters. Here’s an example of a simple function template that swaps two values:
template <typename T>
void swapValues(T& a, T& b) {
T temp = a;
a = b;
b = temp;
}
int main() {
int x = 5, y = 10;
swapValues(x, y); // Calls the swapValues function with int type
double a = 3.14, b = 2.71;
swapValues(a, b); // Calls the swapValues function with double type
return 0;
}
In this example, the swapValues
function template can be used with different data types (int
and double
) without having to write separate functions for each type.
- Class Templates: Class templates allow you to create generic classes that can work with different data types. You define a template class similarly to a function template, but you specify template parameters for the class itself. Here’s an example of a simple class template for a generic stack:
template <typename T>
class Stack {
private:
std::vector<T> elements;
public:
void push(const T& value) {
elements.push_back(value);
}
void pop() {
if (!elements.empty()) {
elements.pop_back();
}
}
T top() const {
if (!elements.empty()) {
return elements.back();
}
throw std::runtime_error("Stack is empty");
}
bool empty() const {
return elements.empty();
}
};
int main() {
Stack<int> intStack;
intStack.push(42);
intStack.push(10);
std::cout << intStack.top() << std::endl; // Outputs 10
Stack<std::string> stringStack;
stringStack.push("Hello");
stringStack.push("World");
std::cout << stringStack.top() << std::endl; // Outputs "World"
return 0;
}
In this example, the Stack
class template can be instantiated with different types (int
and std::string
) to create stack objects tailored to those types.
- Template Specialization: You can provide specialized implementations for specific data types or situations using template specialization. Template specialization allows you to override the generic template behavior for specific cases.
// Template specialization for a specific type
template <>
void swapValues<std::string>(std::string& a, std::string& b) {
std::swap(a, b);
}
In this example, we specialize the swapValues
function template for std::string
to use std::swap
for more efficient string swapping.
Templates are a fundamental feature in modern C++ and are used extensively in libraries and frameworks to create generic and flexible code. They enable you to write code that is both efficient and highly adaptable to different data types and classes.