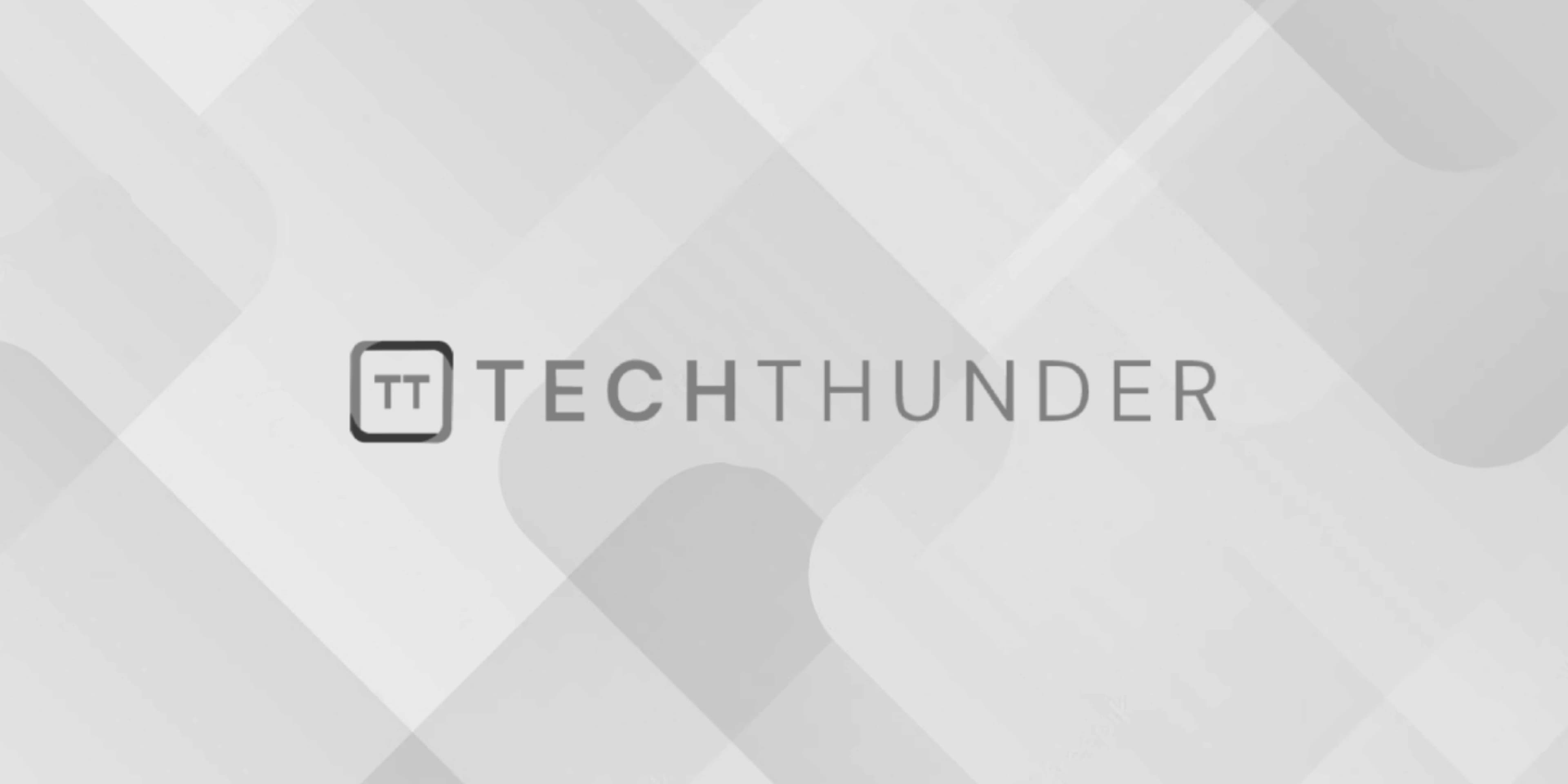
140 views
Constructor vs Destructor in C++
The C++ constructors and destructors are special member functions of a class that are used for object initialization and cleanup, respectively. They have distinct roles and are called at specific points in an object’s lifetime.
Constructor:
- Initialization: Constructors are used to initialize the data members (attributes or fields) of an object when it is created. They ensure that the object is in a valid state upon creation.
- Name Same as Class: Constructors have the same name as the class they belong to and do not have a return type (not even
void
). They can be overloaded, allowing you to define multiple constructors with different parameter lists. - Called Automatically: Constructors are called automatically when an object is created. You do not need to explicitly call a constructor; it is invoked when you create an object using the
new
operator or declare an object on the stack. - Initialization Order: The order in which constructors of base classes and member objects are called depends on the class hierarchy and the order of declaration in the class.
- Use Cases:
- Setting default values for object attributes.
- Allocating resources like memory, opening files, or establishing network connections.
- Initializing object state.
Example of a constructor:
C++
class MyClass {
public:
// Constructor with parameters
MyClass(int value) {
data = value;
}
private:
int data;
};
int main() {
MyClass obj(42); // Constructor is called automatically
return 0;
}
Destructor:
- Cleanup: Destructors are used to release resources and perform cleanup operations when an object goes out of scope or is explicitly deleted. They ensure that resources are deallocated and any necessary cleanup is performed.
- Name Same as Class with a Tilde: Destructors have the same name as the class with a tilde (
~
) prefix, and they do not take any parameters. - Called Automatically: Destructors are called automatically when an object’s scope ends or when
delete
is used to free dynamically allocated memory. - Execution Order: Destructors are executed in the reverse order of object creation. This means that the destructor of a derived class is called before the destructor of its base class.
- Use Cases:
- Releasing resources such as memory, file handles, or database connections.
- Cleaning up any internal state or dependencies created during the object’s lifetime.
Example of a destructor:
C++
class ResourceHolder {
public:
ResourceHolder() {
// Constructor allocates a resource
resource = new int[100];
}
~ResourceHolder() {
// Destructor releases the allocated resource
delete[] resource;
}
private:
int* resource;
};
int main() {
{
ResourceHolder obj; // Constructor is called automatically
} // Destructor is called automatically when obj goes out of scope
return 0;
}
In summary, constructors are responsible for initializing objects, while destructors are responsible for cleaning up resources and performing any necessary cleanup when objects are no longer needed. Together, they ensure that objects are created and destroyed in a controlled and predictable manner, helping manage resource allocation and deallocation.