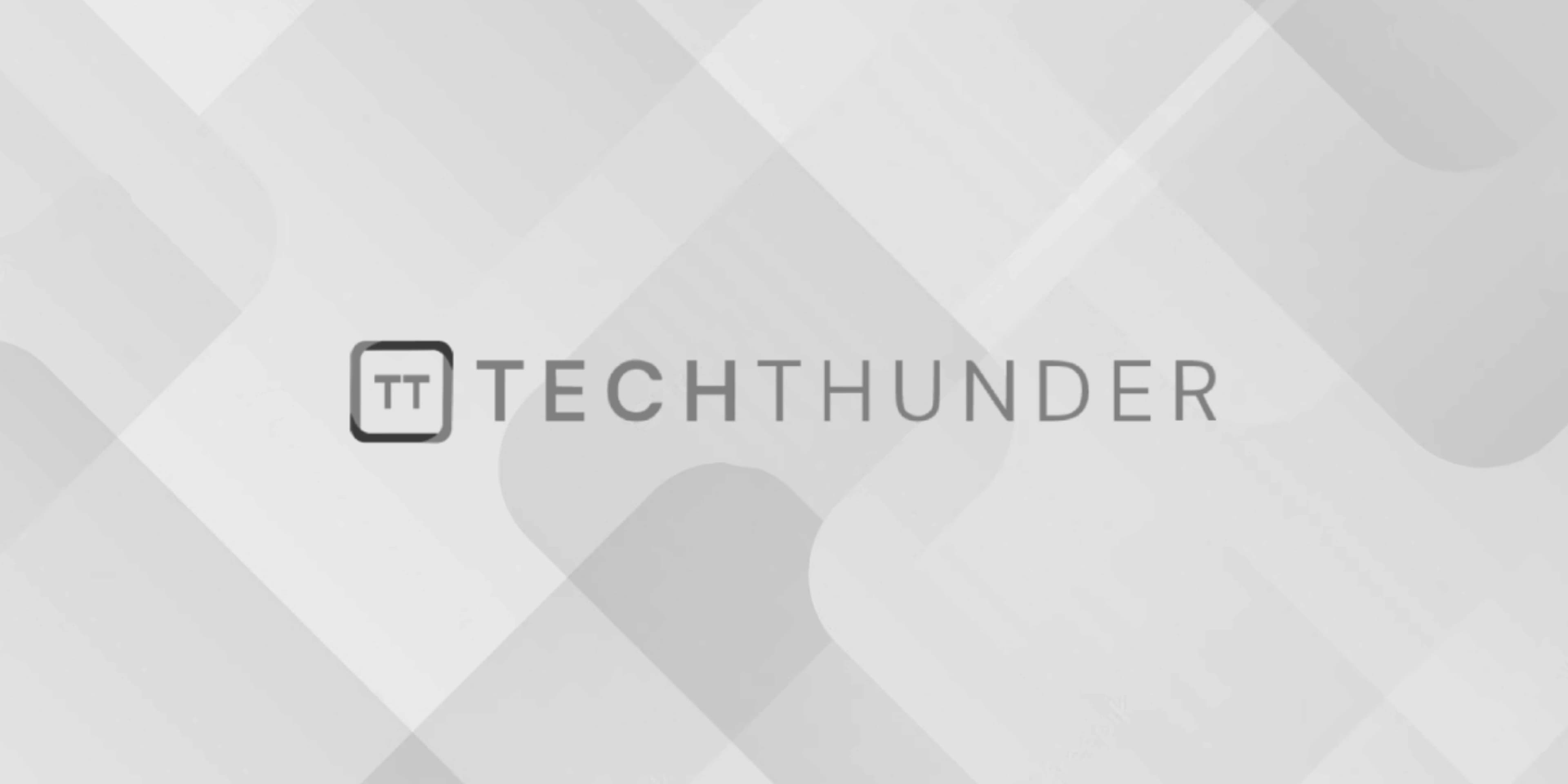
166 views
Different Ways to Compare Strings in C++
The C++, there are multiple ways to compare strings, and the choice of method depends on your specific requirements, such as case sensitivity or the need for custom comparison criteria. Here are some common methods for comparing strings in C++:
==
Operator (Equality Comparison): You can use the==
operator to check if two strings are equal in content. This comparison is case-sensitive.
C++
std::string str1 = "Hello";
std::string str2 = "hello";
if (str1 == str2) {
// Strings are equal
} else {
// Strings are not equal
}
!=
Operator (Inequality Comparison): The!=
operator is used to check if two strings are not equal in content.
C++
std::string str1 = "Hello";
std::string str2 = "World";
if (str1 != str2) {
// Strings are not equal
} else {
// Strings are equal
}
compare()
Method: Thecompare()
method of thestd::string
class allows you to compare two strings with more control. It returns an integer value indicating the result of the comparison.
C++
std::string str1 = "apple";
std::string str2 = "banana";
int result = str1.compare(str2);
if (result == 0) {
// Strings are equal
} else if (result < 0) {
// str1 is lexicographically less than str2
} else {
// str1 is lexicographically greater than str2
}
You can also use compare()
with additional parameters to control case sensitivity and specify a subrange of the strings to compare.
<
,<=
,>
,>=
Operators (Lexicographic Comparison): C++ allows you to use the less than<
, less than or equal to<=
, greater than>
, and greater than or equal to>=
operators to compare strings lexicographically.
C++
std::string str1 = "apple";
std::string str2 = "banana";
if (str1 < str2) {
// str1 is lexicographically less than str2
} else if (str1 > str2) {
// str1 is lexicographically greater than str2
} else {
// Strings are equal
}
- Custom Comparison Criteria: If you have specific requirements for string comparison, you can write your own custom comparison function or use lambda expressions with the
std::sort()
function or other algorithms. This allows you to implement case-insensitive comparison or other custom logic.
C++
std::string str1 = "apple";
std::string str2 = "Banana";
bool result = std::equal(str1.begin(), str1.end(), str2.begin(), str2.end(),
[](char a, char b) { return std::tolower(a) == std::tolower(b); });
if (result) {
// Strings are equal (case-insensitive)
} else {
// Strings are not equal (case-insensitive)
}
Remember to choose the appropriate method for comparing strings based on your specific needs, such as case sensitivity, lexicographic order, or custom comparison criteria.