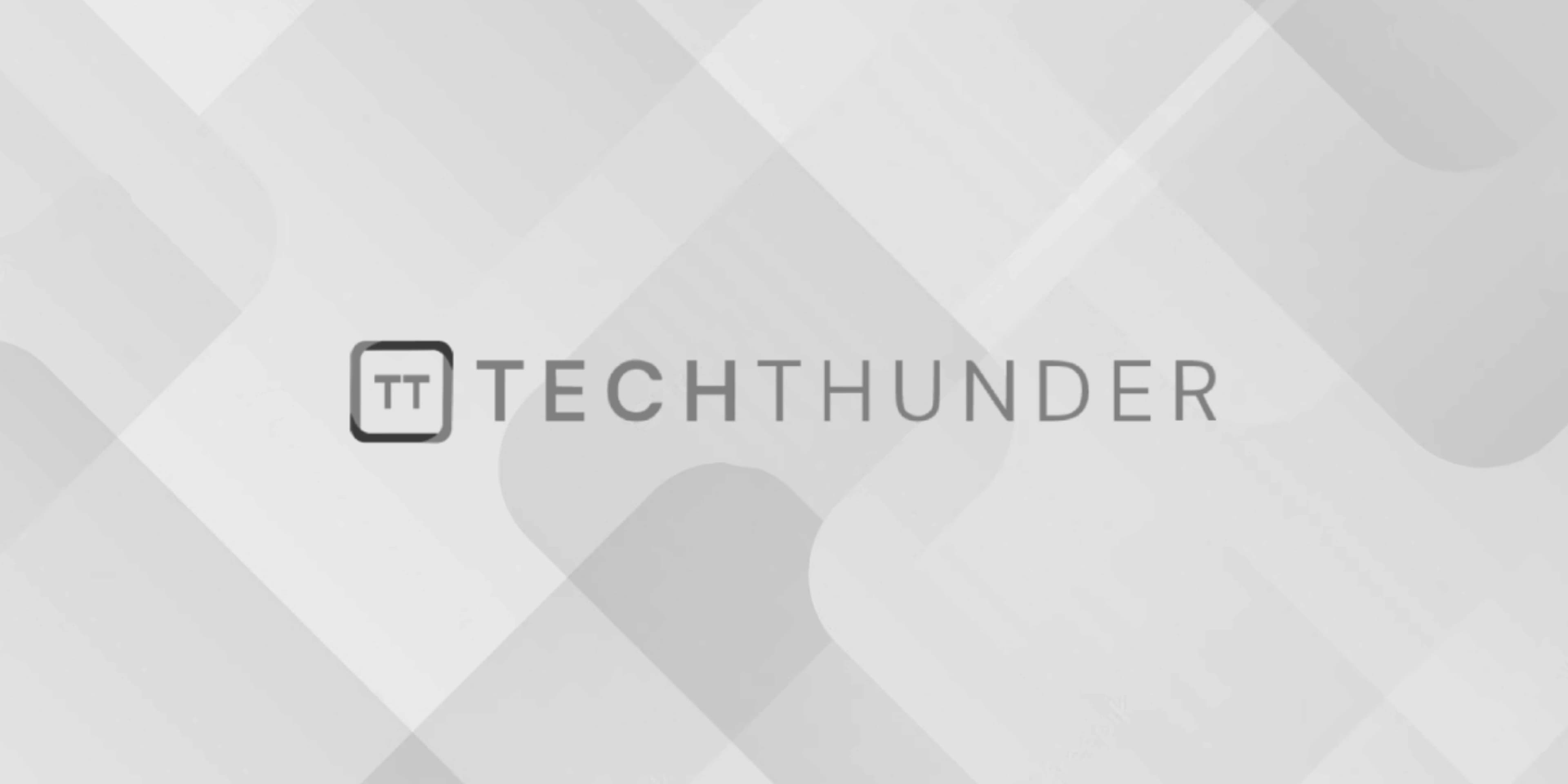
154 views
C++ int to string
The C++ can convert an int
to a std::string
using various methods. Here are two common approaches:
- Using
std::to_string
(C++11 and later): The most straightforward way to convert anint
to astd::string
is to use thestd::to_string
function, which is available in C++11 and later versions of the language.
C++
#include <iostream>
#include <string>
int main() {
int number = 42;
std::string str_number = std::to_string(number);
std::cout << "Integer as string: " << str_number << std::endl;
return 0;
}
This code will convert the integer 42
to the string "42"
.
- Using
std::stringstream
: Another way to convert anint
to astd::string
is to use astd::stringstream
. This approach is available in all C++ versions and can be especially useful when working with older compilers or environments that do not support C++11 features.
C++
#include <iostream>
#include <sstream>
#include <string>
int main() {
int number = 42;
std::stringstream ss;
ss << number;
std::string str_number = ss.str();
std::cout << "Integer as string: " << str_number << std::endl;
return 0;
}
This code achieves the same result as the previous example, converting the integer 42
to the string "42"
.
Both of these methods are efficient and straightforward for converting integers to strings in C++. The choice between them largely depends on your preference and the version of C++ you are using. If you are working with C++11 or later, std::to_string
is generally recommended for its simplicity.