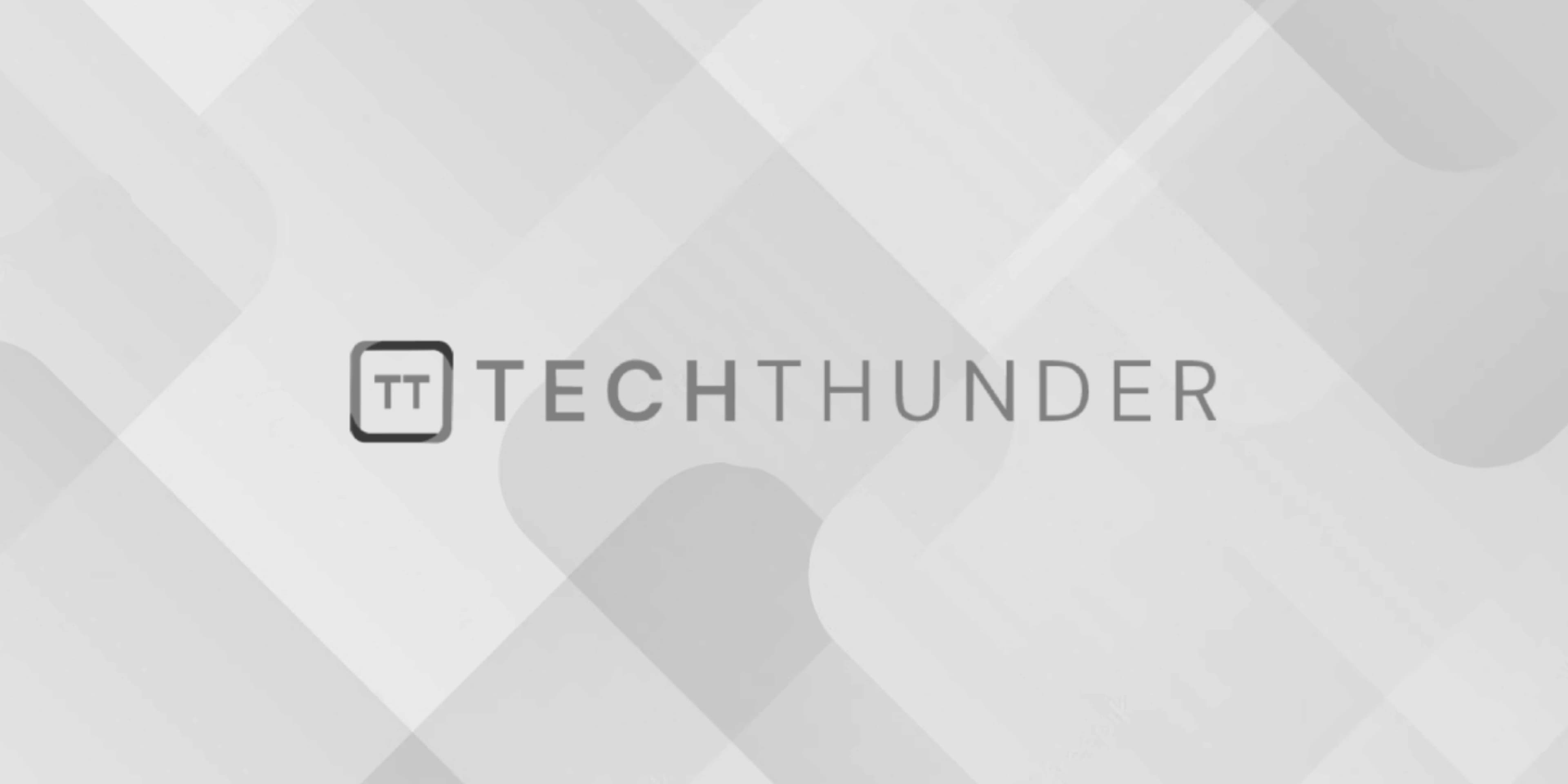
203 views
Hotel Management in C++
Creating a hotel management system in C++ involves designing a program that can manage various aspects of a hotel, such as room booking, guest check-in/check-out, room availability, billing, and more. Below is a simplified example of a hotel management system in C++. Please note that this is a basic implementation and does not cover all the complexities that a real-world system would entail.
C++
#include <iostream>
#include <vector>
#include <string>
using namespace std;
// Define a class to represent a hotel room
class HotelRoom {
public:
HotelRoom(int roomNumber, int capacity, double pricePerNight)
: roomNumber(roomNumber), capacity(capacity), pricePerNight(pricePerNight), isOccupied(false) {}
int getRoomNumber() const {
return roomNumber;
}
int getCapacity() const {
return capacity;
}
double getPricePerNight() const {
return pricePerNight;
}
bool isOccupied() const {
return isOccupied;
}
void occupyRoom() {
isOccupied = true;
}
void vacateRoom() {
isOccupied = false;
}
private:
int roomNumber;
int capacity;
double pricePerNight;
bool isOccupied;
};
// Define a class to represent a hotel
class Hotel {
public:
Hotel(const string& name) : name(name) {}
// Add a room to the hotel
void addRoom(int roomNumber, int capacity, double pricePerNight) {
rooms.push_back(HotelRoom(roomNumber, capacity, pricePerNight));
}
// Display available rooms
void displayAvailableRooms() {
cout << "Available Rooms at " << name << ":" << endl;
for (const HotelRoom& room : rooms) {
if (!room.isOccupied()) {
cout << "Room Number: " << room.getRoomNumber() << ", Capacity: " << room.getCapacity()
<< ", Price per Night: $" << room.getPricePerNight() << endl;
}
}
}
// Book a room
void bookRoom(int roomNumber) {
for (HotelRoom& room : rooms) {
if (room.getRoomNumber() == roomNumber && !room.isOccupied()) {
room.occupyRoom();
cout << "Room " << roomNumber << " has been booked." << endl;
return;
}
}
cout << "Room " << roomNumber << " is not available for booking." << endl;
}
// Check-out from a room
void checkOut(int roomNumber) {
for (HotelRoom& room : rooms) {
if (room.getRoomNumber() == roomNumber && room.isOccupied()) {
room.vacateRoom();
cout << "Checked out from Room " << roomNumber << "." << endl;
return;
}
}
cout << "Room " << roomNumber << " is not occupied." << endl;
}
private:
string name;
vector<HotelRoom> rooms;
};
int main() {
Hotel myHotel("MyHotel");
// Add rooms to the hotel
myHotel.addRoom(101, 2, 100.0);
myHotel.addRoom(102, 4, 200.0);
myHotel.addRoom(103, 1, 50.0);
// Display available rooms
myHotel.displayAvailableRooms();
// Book a room
myHotel.bookRoom(102);
// Display available rooms after booking
myHotel.displayAvailableRooms();
// Check-out from a room
myHotel.checkOut(102);
// Display available rooms after check-out
myHotel.displayAvailableRooms();
return 0;
}
This is a basic implementation to give you an idea of how a hotel management system in C++ could be structured. In a real-world scenario, you would likely need to include additional features like guest information, reservation management, billing, and more advanced error handling.