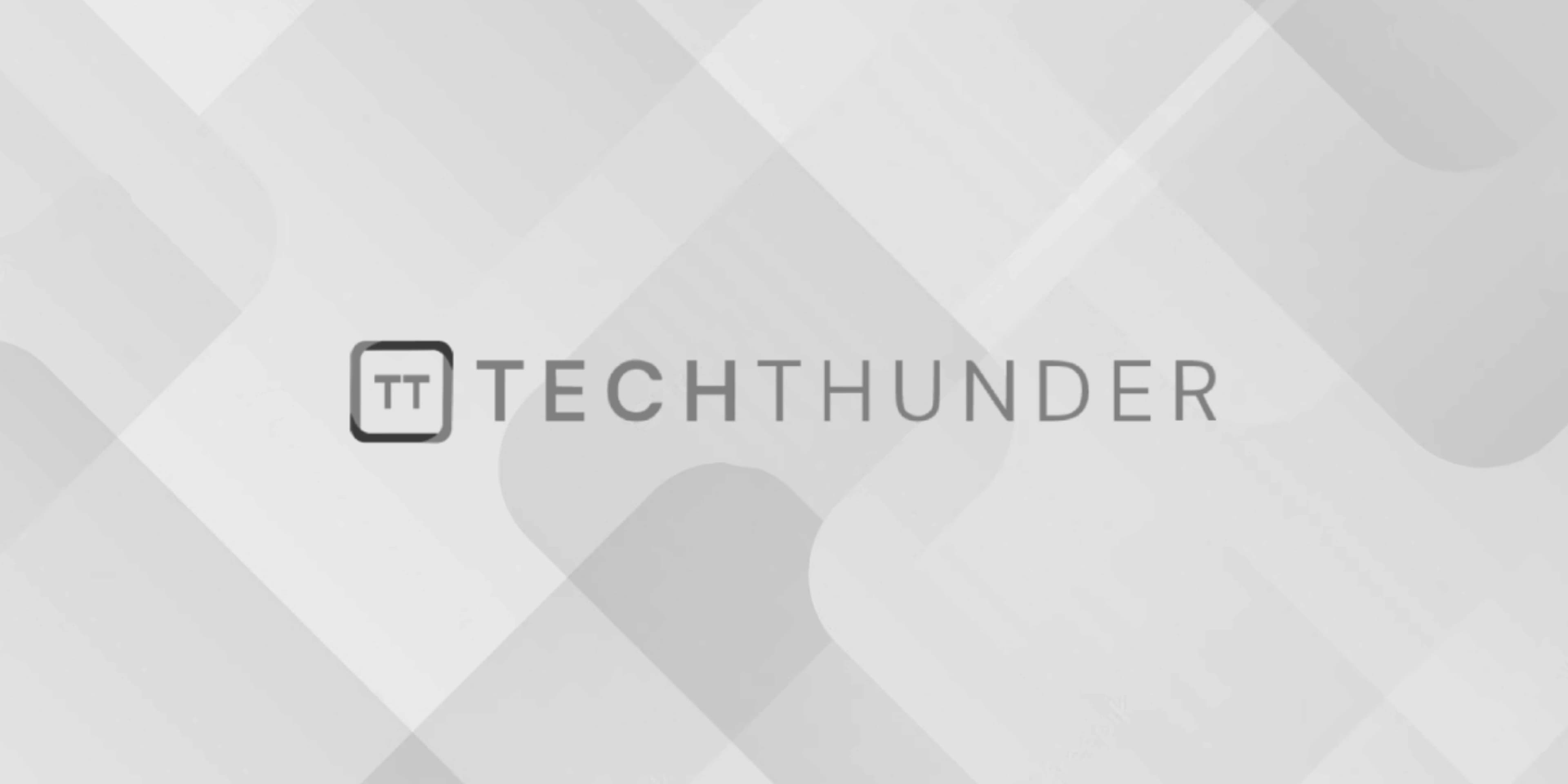
C++ Friend Function
The C++ friend function is a function that is not a member of a class but is granted access to the private and protected members of that class. Friend functions are often used when you need to allow external functions or classes to operate on private or protected data members of a class. They can be declared inside the class with the friend
keyword and then defined outside the class.
Here’s how to declare and use friend functions in C++:
class MyClass {
private:
int data;
public:
MyClass(int d) : data(d) {}
// Declare the friend function inside the class
friend void friendFunction(const MyClass& obj);
};
// Define the friend function outside the class
void friendFunction(const MyClass& obj) {
// The friend function can access the private data member of MyClass
std::cout << "Friend Function: " << obj.data << std::endl;
}
int main() {
MyClass obj(42);
friendFunction(obj);
return 0;
}
In this example, we have a MyClass
class with a private data member data
and a friend function friendFunction
declared inside the class. The friend function is then defined outside the class. Inside friendFunction
, we can access the private data
member of MyClass
because it has been declared as a friend of the class.
Key points about friend functions in C++:
- Friend Functions Are Not Members: Friend functions are not part of the class’s member functions. They are standalone functions that are given special access privileges to the class’s private and protected members.
- Declaration Inside the Class: Friend functions are declared inside the class with the
friend
keyword. This declaration informs the compiler that the function is a friend of the class and has access to its private and protected members. - Definition Outside the Class: The friend function is defined outside the class, just like any other regular function.
- Access to Private Members: Friend functions can access private and protected members of the class for which they are declared as friends. This allows for more flexible encapsulation and controlled access.
- No Member Access Rights: Friend functions do not have the
this
pointer and do not belong to any class. They cannot access class members that are not explicitly declared as friends. - Friendship Is Not Inherited: Friendship is not inherited. If class A is a friend of class B, and class C derives from class B, class C does not automatically have access to the private members of class A.
- Use with Caution: While friend functions can be useful in certain situations, they should be used sparingly. Overusing friend functions can break encapsulation and hinder the benefits of object-oriented programming.
Friend functions are a way to provide controlled access to a class’s private and protected members for specific external functions or classes that need such access. They are often used in scenarios where encapsulation needs to be relaxed slightly to accommodate certain operations while still maintaining overall data integrity.