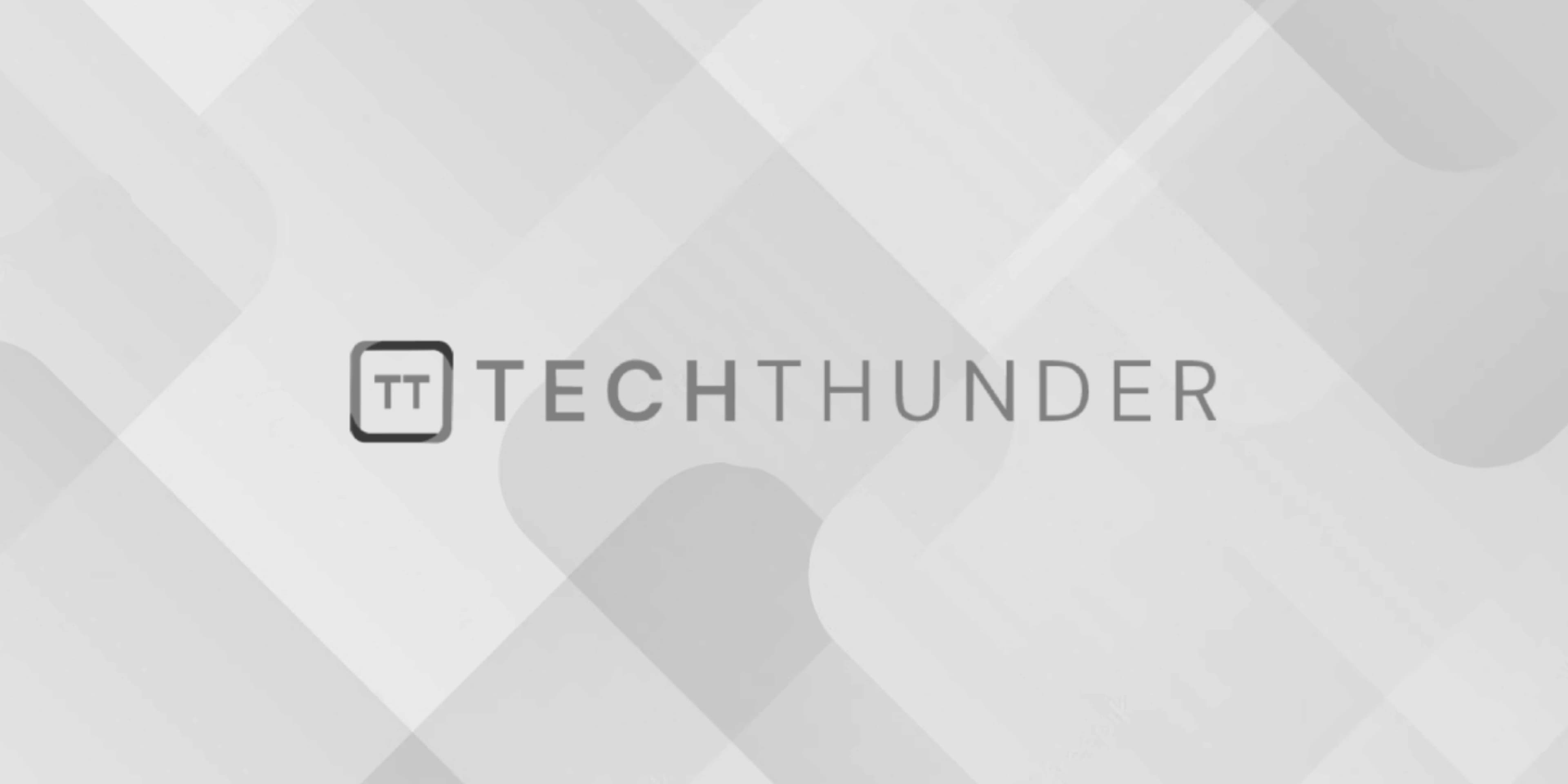
125 views
Add two Array in C++
To add two arrays in C++, you can create a new array that stores the sum of corresponding elements from the two input arrays. Here’s an example of how to do this:
C++
#include <iostream>
int main() {
const int size = 5; // Size of the arrays
int array1[size] = {1, 2, 3, 4, 5};
int array2[size] = {6, 7, 8, 9, 10};
int sumArray[size]; // Array to store the sum
// Add the corresponding elements from array1 and array2
for (int i = 0; i < size; ++i) {
sumArray[i] = array1[i] + array2[i];
}
// Display the result
std::cout << "Sum of arrays:" << std::endl;
for (int i = 0; i < size; ++i) {
std::cout << sumArray[i] << " ";
}
std::cout << std::endl;
return 0;
}
In this program:
- We declare two arrays,
array1
andarray2
, each with the same size (size
). - We also declare a third array,
sumArray
, of the same size to store the sum of the corresponding elements ofarray1
andarray2
. - We use a
for
loop to iterate through the elements of the arrays and add the corresponding elements together, storing the result insumArray
. - Finally, we display the
sumArray
to show the result.
When you run this program, it will add the two input arrays element-wise and display the sum.