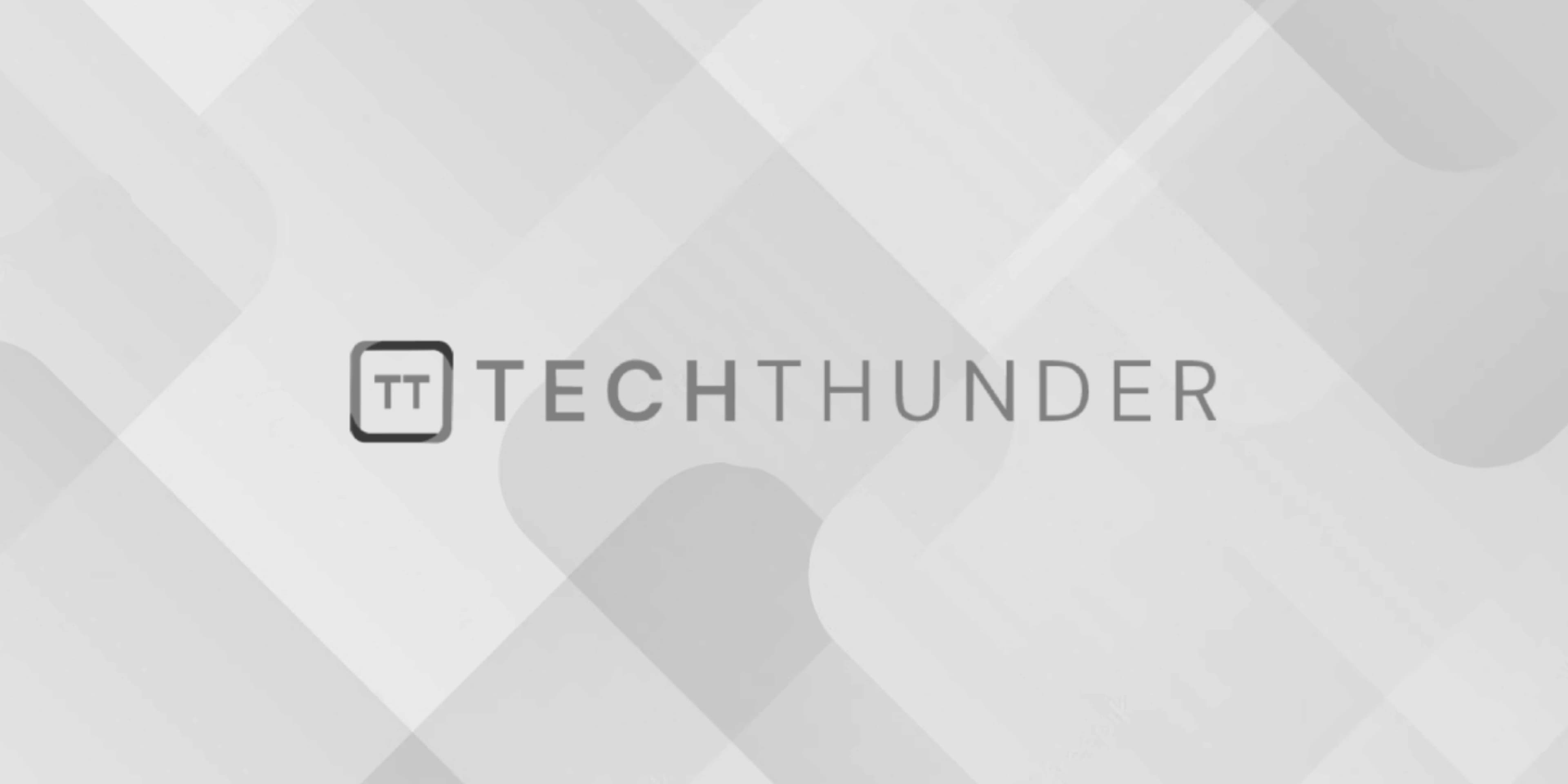
Exception Handling in C++ and JAVA
Exception handling is a programming construct that allows you to deal with unexpected or exceptional situations in your code. Both C++ and Java provide mechanisms for handling exceptions, but they have different syntax and approaches.
Exception Handling in C++:
In C++, exception handling is done using the try
, catch
, and throw
keywords:
try
: Thetry
block encloses the code where an exception might occur.catch
: Thecatch
block is used to catch and handle exceptions. It specifies the type of exception it can catch.throw
: Thethrow
keyword is used to throw an exception when a problem is encountered in thetry
block.
Here’s an example in C++:
#include <iostream>
#include <stdexcept>
int main() {
try {
int a = 10;
int b = 0;
if (b == 0) {
throw std::runtime_error("Division by zero");
}
int result = a / b;
std::cout << "Result: " << result << std::endl;
} catch (const std::exception& e) {
std::cerr << "Exception caught: " << e.what() << std::endl;
}
return 0;
}
In this example, a std::runtime_error
exception is thrown when dividing by zero, and it’s caught in the catch
block.
Exception Handling in Java:
In Java, exception handling is done using try
, catch
, finally
, and throw
:
try
: Thetry
block encloses the code where an exception might occur.catch
: Thecatch
block is used to catch and handle exceptions. It specifies the type of exception it can catch.finally
: Thefinally
block is used to specify code that should be executed regardless of whether an exception occurred or not.throw
: Thethrow
keyword is used to throw an exception when a problem is encountered in thetry
block.
Here’s an example in Java:
public class ExceptionHandlingExample {
public static void main(String[] args) {
try {
int a = 10;
int b = 0;
if (b == 0) {
throw new ArithmeticException("Division by zero");
}
int result = a / b;
System.out.println("Result: " + result);
} catch (ArithmeticException e) {
System.err.println("Exception caught: " + e.getMessage());
}
}
}
In this Java example, an ArithmeticException
is thrown when dividing by zero, and it’s caught in the catch
block.
While the concepts of exception handling are similar in both C++ and Java, the specific syntax, exception classes, and mechanisms can differ between the two languages. However, the core idea of gracefully handling exceptional situations remains the same.