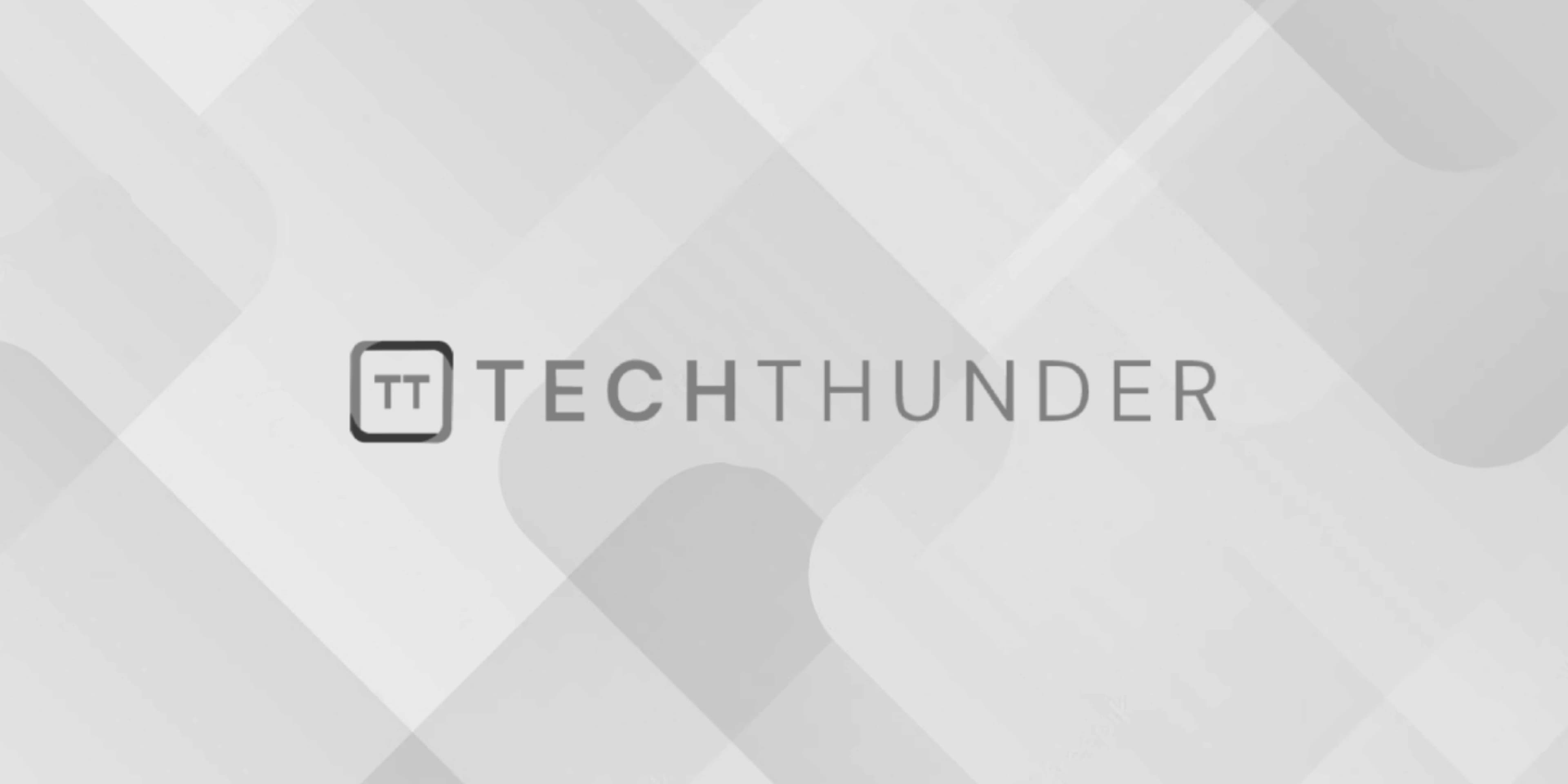
Raw string literal in C++
The C++ raw string literal is a way to define a string literal without the need to escape special characters (like backslashes and double quotes). Raw string literals are enclosed in parentheses and introduced with the R
prefix followed by a delimiter of your choice (commonly a pair of parentheses, square brackets, or curly braces), and the string content is placed between the delimiters.
Here’s the syntax for a raw string literal:
R"delimiter(string_content)delimiter"
R
: The prefix indicating that it’s a raw string literal.delimiter
: A sequence of characters that marks the start and end of the string content. You can choose the delimiter, and it should not appear in the string content.
Here are a few examples of raw string literals:
#include <iostream>
int main() {
// Using parentheses as delimiters
std::string s1 = R"(This is a raw string with () characters.)";
// Using square brackets as delimiters
std::string s2 = R"([This is another raw string with [] characters.])";
// Using curly braces as delimiters
std::string s3 = R"({This is a raw string with {} characters.})";
std::cout << s1 << std::endl;
std::cout << s2 << std::endl;
std::cout << s3 << std::endl;
return 0;
}
In this example:
- We define three raw string literals, each using different delimiters:
()
,[]
, and{}
. - The string content within the raw string literals doesn’t require escaping, making it convenient for representing strings with special characters.
Raw string literals are particularly useful when you need to include characters like backslashes, double quotes, or newline characters in your string without manually escaping them. They are commonly used in scenarios such as regular expressions, file paths, and multi-line string literals.