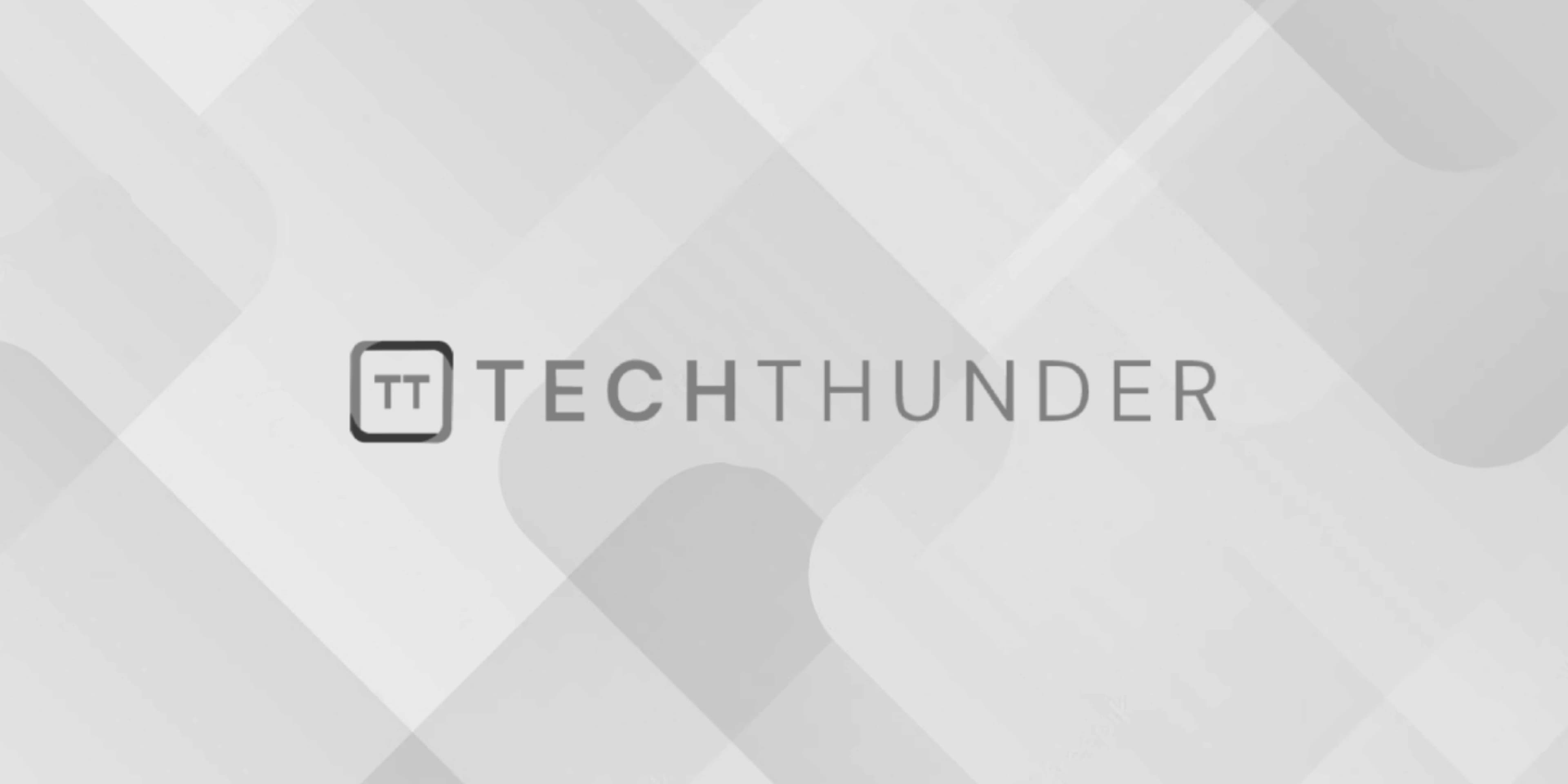
Name Mangling and Extern C in C++
The name mangling and the extern "C"
linkage specification are related concepts that deal with how C++ and C functions are represented and linked together when you have mixed-language code, i.e., a combination of C++ and C code in the same program. These concepts help ensure that C++ code can call C functions and vice versa without any issues related to function name resolution and symbol linkage.
Name Mangling:
- Name mangling is a technique used by C++ compilers to encode the names of functions and variables in a way that encodes additional information, such as function signatures (overloaded functions) and namespaces. This is necessary because C++ supports function overloading, whereas C does not.
- When you declare or define functions or classes in C++ code, the compiler mangles their names to include information about the function’s arguments and return types. For example, if you have two overloaded functions like
int add(int a, int b)
anddouble add(double a, double b)
, their mangled names will be different. - Name mangling ensures that overloaded C++ functions can be distinguished from each other during the linking process.
extern "C"
Linkage Specification:
- The
extern "C"
linkage specification is used in C++ to declare that a particular function should have C linkage, meaning that its name should not be mangled, and it should be linked using the C calling convention. - When you declare a C function within a C++ source file and specify
extern "C"
, it tells the C++ compiler to use C linkage for that function. This is useful when you want to call C functions from C++ code or when you’re linking with external C libraries.
Here’s an example demonstrating the usage of extern "C"
:
// C++ code
#include <iostream>
// Declaration of a C function with C linkage
extern "C" {
int c_function(int a, int b);
}
int main() {
int result = c_function(5, 3);
std::cout << "Result: " << result << std::endl;
return 0;
}
// C code (c_functions.c)
int c_function(int a, int b) {
return a + b;
}
In this example:
- The C++ code declares a C function
c_function
usingextern "C"
linkage. This tells the C++ compiler to use C linkage for this function. - The C code in the
c_functions.c
file defines thec_function
as a standard C function.
When you compile and link these files together, there won’t be any name mangling for c_function
, and it can be called from C++ code without any issues.
By using extern "C"
linkage specification, you can seamlessly integrate C and C++ code in mixed-language projects and ensure that the function names are compatible between the two languages.