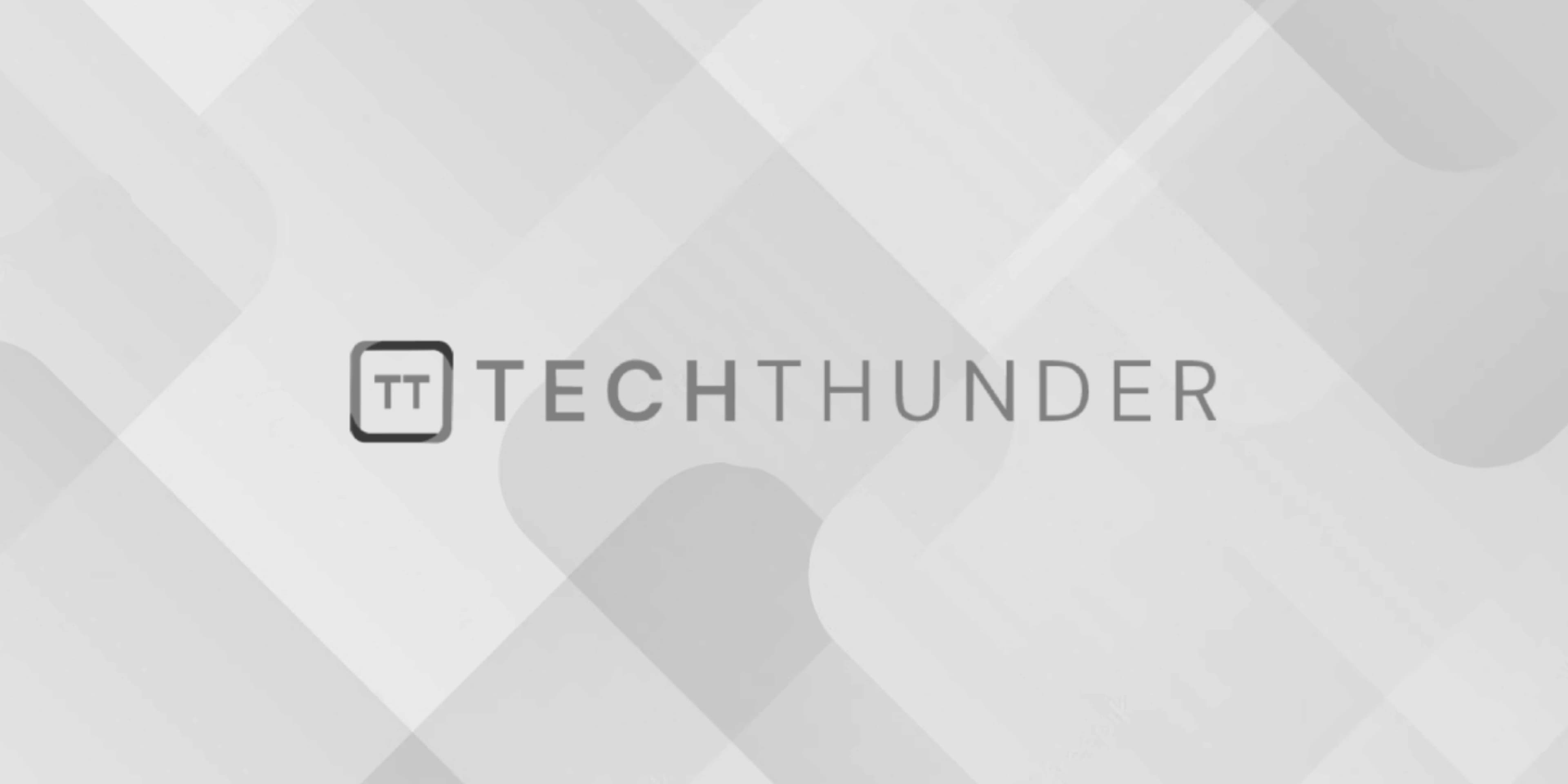
130 views
Add Two no in C++ program
The simple C++ program that adds two numbers entered by the user:
C++
#include <iostream>
int main() {
double num1, num2, sum;
// Input the first number
std::cout << "Enter the first number: ";
std::cin >> num1;
// Input the second number
std::cout << "Enter the second number: ";
std::cin >> num2;
// Add the two numbers
sum = num1 + num2;
// Display the result
std::cout << "The sum of " << num1 << " and " << num2 << " is: " << sum << std::endl;
return 0;
}
In this program:
- We declare three variables:
num1
,num2
, andsum
to store the two numbers entered by the user and the result of their addition, respectively. - We use
std::cout
andstd::cin
to display prompts to the user and to input the two numbers. - The
+
operator is used to addnum1
andnum2
, and the result is stored in thesum
variable. - Finally, we use
std::cout
to display the sum of the two numbers to the user.
When you run this program, it will ask the user for two numbers, add them, and then display the result.