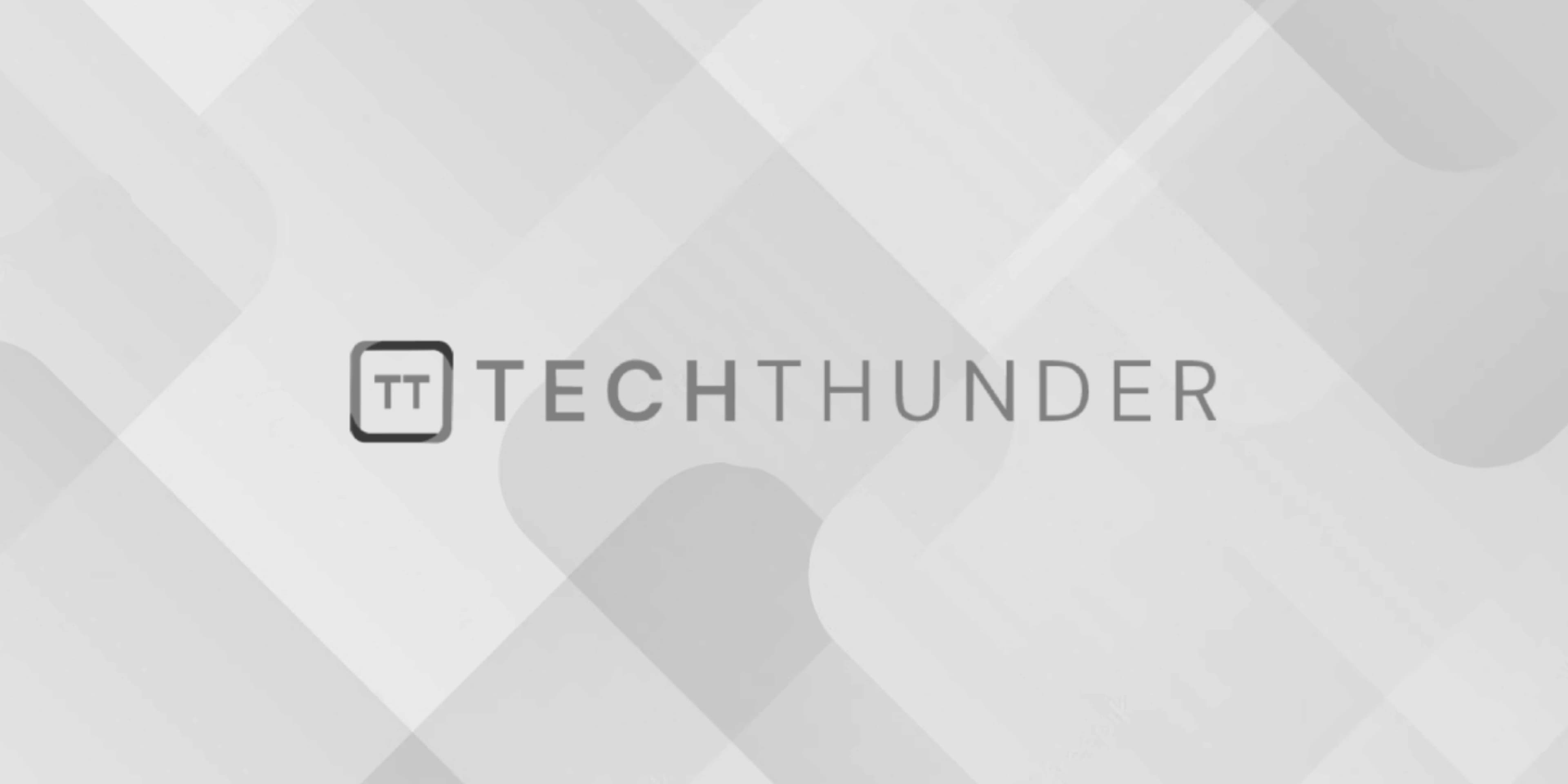
Dynamic Initialization of Objects in C++
The C++ dynamic initialization of objects typically involves using pointers or smart pointers to create objects on the heap (i.e., in dynamic memory) rather than on the stack (i.e., in automatic or local memory). This allows you to create and manage objects with a dynamic lifetime, controlled by you. Here’s how to dynamically initialize objects in C++:
- Using Pointers (Raw Pointers): You can allocate memory for objects on the heap using
new
anddelete
operators. Here’s an example:
#include <iostream>
class MyClass {
public:
MyClass(int val) : value(val) {}
void printValue() const {
std::cout << "Value: " << value << std::endl;
}
private:
int value;
};
int main() {
// Dynamic allocation of an object using new
MyClass* ptr = new MyClass(42);
// Access and use the dynamically created object
ptr->printValue();
// Deallocate memory using delete
delete ptr;
return 0;
}
In this example, we create an instance of MyClass
on the heap using new
, and then we access and use it through the pointer ptr
. Finally, we release the memory using delete
.
- Using Smart Pointers (C++11 and Later): C++11 introduced smart pointers (
std::shared_ptr
,std::unique_ptr
, andstd::weak_ptr
) that make dynamic memory management safer and more convenient. Here’s an example usingstd::unique_ptr
:
#include <iostream>
#include <memory> // Include the memory header for smart pointers
class MyClass {
public:
MyClass(int val) : value(val) {}
void printValue() const {
std::cout << "Value: " << value << std::endl;
}
private:
int value;
};
int main() {
// Dynamic allocation of an object using std::unique_ptr
std::unique_ptr<MyClass> ptr = std::make_unique<MyClass>(42);
// Access and use the dynamically created object
ptr->printValue();
// No need to manually deallocate memory; it's handled automatically when 'ptr' goes out of scope
return 0;
}
In this example, we use std::make_unique
to create a std::unique_ptr
that manages the lifetime of the object. The memory is automatically deallocated when the std::unique_ptr
goes out of scope.
Using smart pointers is generally recommended because they help prevent memory leaks and are safer than raw pointers. The choice between std::shared_ptr
and std::unique_ptr
depends on your specific requirements for ownership and sharing of objects.