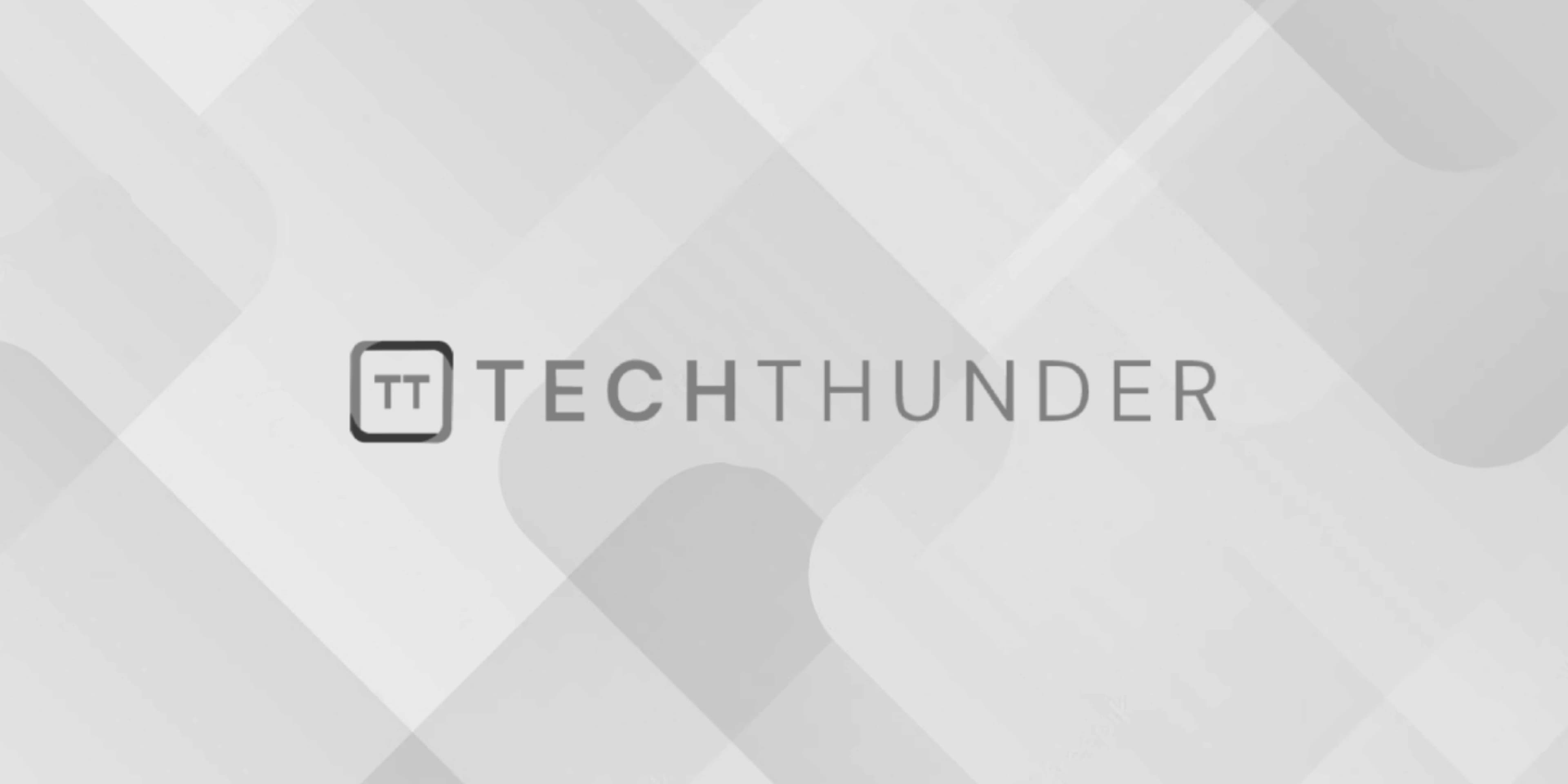
109 views
Fibonacci Triangle in C++
A Fibonacci triangle in C++ is a pattern of numbers where each number in a row is the sum of the two numbers directly above it in the previous row, similar to the Fibonacci sequence. Here’s how you can create a Fibonacci triangle in C++:
C++
#include <iostream>
int main() {
int n;
// Input the number of rows for the Fibonacci triangle
std::cout << "Enter the number of rows: ";
std::cin >> n;
int a = 0, b = 1, c;
for (int i = 1; i <= n; ++i) {
a = 0;
b = 1;
for (int j = 1; j <= i; ++j) {
std::cout << a << " ";
c = a + b;
a = b;
b = c;
}
std::cout << std::endl;
}
return 0;
}
In this program:
- The user is prompted to enter the number of rows for the Fibonacci triangle.
- Two variables
a
andb
are used to keep track of the last two Fibonacci numbers, initially set to 0 and 1. - Nested loops are used to iterate through the rows and columns of the triangle.
- In the inner loop, the current Fibonacci number (
a
) is printed, and the next Fibonacci number is calculated (c = a + b
). Then,a
is updated tob
, andb
is updated toc
. - The result is a pattern of numbers forming a Fibonacci triangle.
For example, if you enter 5
as the number of rows, the program will generate the following Fibonacci triangle:
Plaintext
0
1 1
1 2 3
5 8 13 21
34 55 89 144 233
You can adjust the number of rows by changing the value of n
to create a Fibonacci triangle of the desired size.