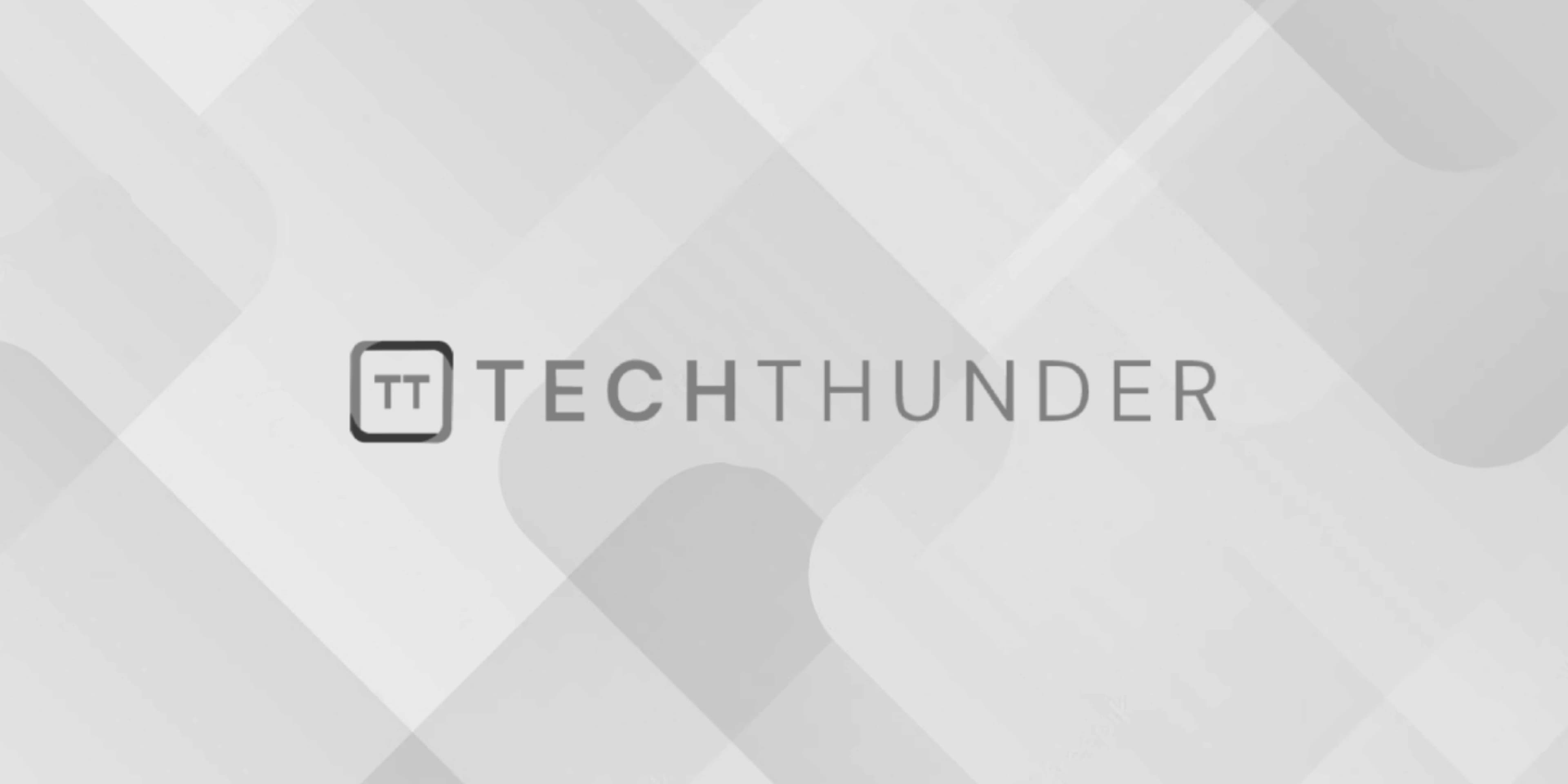
C++ Math Functions
The C++ can perform mathematical operations using various built-in math functions provided by the Standard Library’s <cmath>
or <math.h>
(for C compatibility) header. These functions cover a wide range of mathematical operations, including basic arithmetic, trigonometry, logarithms, exponentiation, and more. Here are some commonly used math functions:
- Basic Arithmetic Functions:
+
,-
,*
,/
: These are the basic arithmetic operators for addition, subtraction, multiplication, and division, respectively.
- Trigonometric Functions:
sin(x)
: Computes the sine ofx
(in radians).cos(x)
: Computes the cosine ofx
(in radians).tan(x)
: Computes the tangent ofx
(in radians). You can also find their inverse counterparts usingasin()
,acos()
, andatan()
.
- Exponentiation Functions:
exp(x)
: Calculates the exponential function e^x.log(x)
: Computes the natural logarithm (base e) ofx
.log10(x)
: Computes the base-10 logarithm ofx
.
- Power Functions:
pow(x, y)
: Raisesx
to the power ofy
.
- Square Root:
sqrt(x)
: Calculates the square root ofx
.
- Absolute Value:
fabs(x)
: Returns the absolute (positive) value ofx
.
- Rounding Functions:
ceil(x)
: Roundsx
up to the nearest integer.floor(x)
: Roundsx
down to the nearest integer.round(x)
: Roundsx
to the nearest integer (round half up).
- Random Number Generation:
rand()
: Generates a pseudo-random integer between 0 andRAND_MAX
. Remember to seed the random number generator withsrand()
before usingrand()
.
- Other Functions:
min(x, y)
: Returns the smaller ofx
andy
.max(x, y)
: Returns the larger ofx
andy
.abs(x)
: Equivalent tofabs(x)
; returns the absolute value ofx
.fmod(x, y)
: Computes the remainder whenx
is divided byy
.
To use these math functions, you typically include the <cmath>
header and call them with appropriate arguments. For example:
#include <iostream>
#include <cmath>
int main() {
double x = 2.5;
double y = 3.0;
double result = pow(x, y); // Compute x^y
std::cout << "Result: " << result << std::endl;
return 0;
}
Remember that mathematical functions like sin
, cos
, and exp
often expect angles to be in radians, so you may need to convert degrees to radians if necessary. Additionally, floating-point arithmetic may introduce some precision issues, so be aware of potential rounding errors in your calculations.