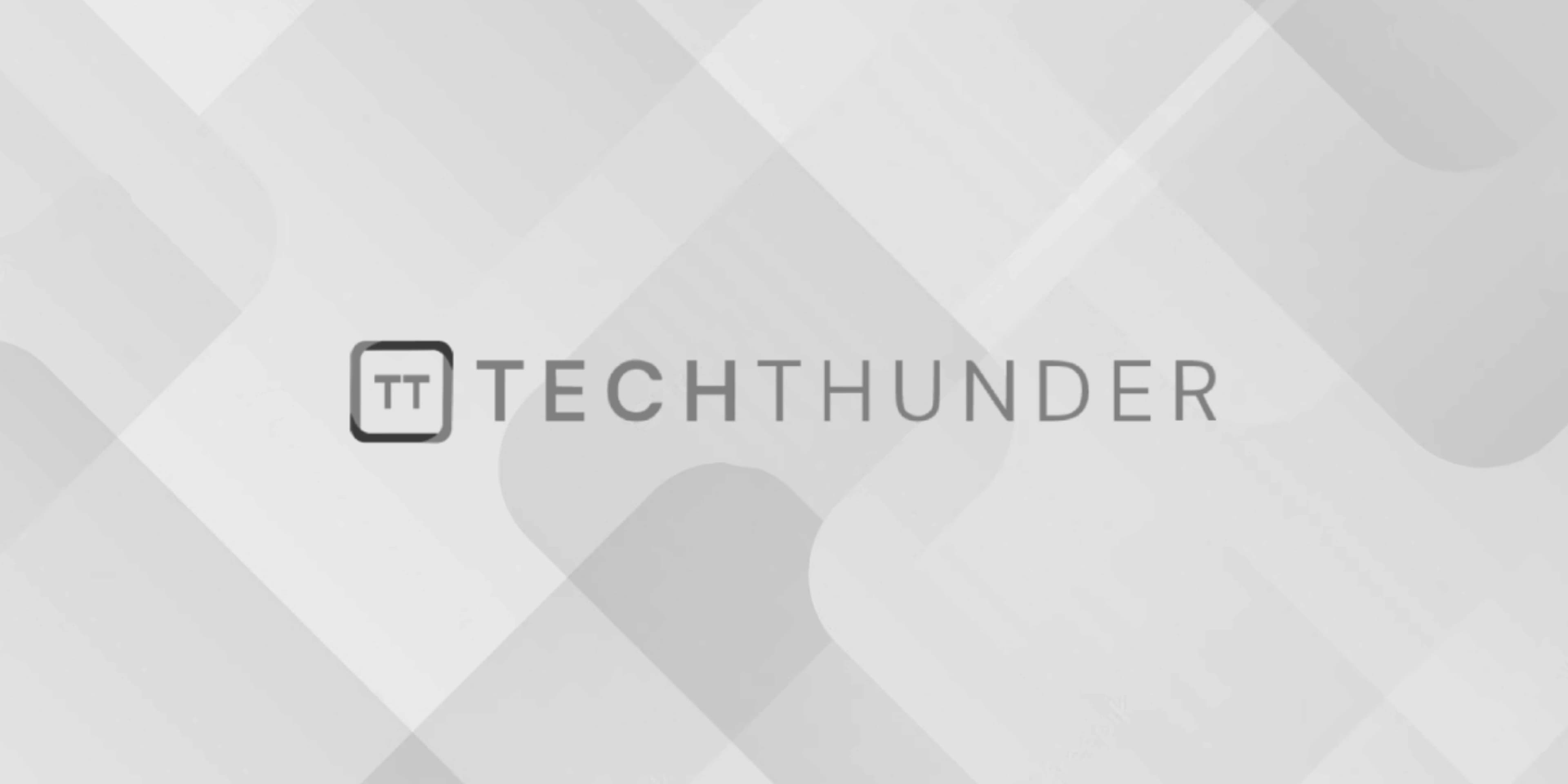
355 views
Difference between Circular Queue and Priority Queue in C++
Circular Queue and Priority Queue are two different data structures used in C++ for specific purposes. They have distinct characteristics and use cases:
Circular Queue:
- Data Structure Type: Circular Queue is a type of linear data structure that follows the First-In-First-Out (FIFO) principle, just like a regular queue.
- Order of Elements: Elements are stored in a sequential order, and when the queue is full, it wraps around and overwrites the oldest elements.
- Usage: Circular queues are used when you want to maintain a fixed-size queue and reuse the empty slots after it becomes full. They are useful in scenarios where elements need to be processed in a cyclic or circular manner.
- Operations: Common operations on a circular queue include enqueue (inserting an element at the rear), dequeue (removing an element from the front), checking if the queue is empty or full, and traversing the queue.
- Implementation: Circular queues can be implemented using arrays or linked lists.
Priority Queue:
- Data Structure Type: Priority Queue is a data structure that is not necessarily linear. It is often implemented as a binary heap, which is a tree-based data structure.
- Order of Elements: Elements in a priority queue are not stored in a specific order like a queue. Instead, they are ordered based on their priority or value. The element with the highest priority (or lowest value, depending on the implementation) is always at the front.
- Usage: Priority queues are used when you need to maintain elements in a way that allows quick access to the highest-priority element. They are used in algorithms like Dijkstra’s algorithm, heap sort, and various scheduling algorithms.
- Operations: Common operations on a priority queue include inserting elements with priorities, removing the highest-priority element, checking if the queue is empty, and peeking at the highest-priority element.
- Implementation: Priority queues are typically implemented as binary heaps. They are not limited by a fixed size, and elements can be dynamically added or removed while maintaining the priority order.
In summary, the key difference between a Circular Queue and a Priority Queue lies in their fundamental characteristics and usage. Circular queues are used for maintaining a fixed-size, cyclic buffer of elements, while priority queues are used for managing elements based on their priority or value, with dynamic resizing capabilities. Each data structure serves a specific purpose and is chosen based on the requirements of the problem at hand.