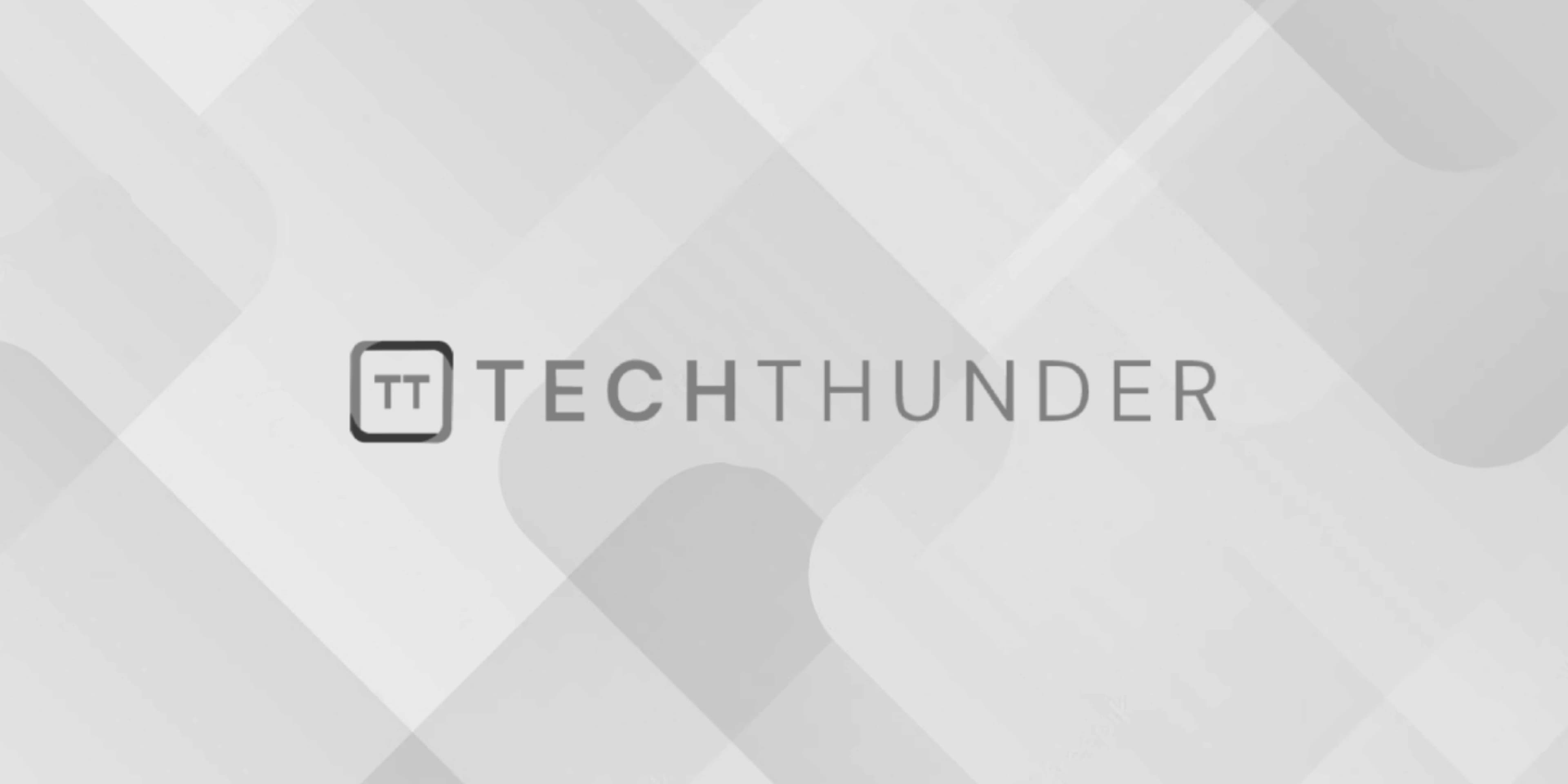
What is Top-Down Approach in C++
A top-down approach in programming refers to a problem-solving strategy where you start by solving a larger problem and then break it down into smaller subproblems. It involves solving the problem at hand by recursively solving its subproblems, which are often simpler and similar in nature. This approach is also known as “divide and conquer” because it divides the problem into manageable parts and solves them separately before combining the solutions to solve the larger problem.
The top-down approach is often associated with recursion and recursive algorithms. It’s particularly useful for solving problems that can be broken down into smaller, identical, or similar subproblems. However, without proper optimization, a naive top-down approach might lead to redundant calculations due to solving the same subproblem multiple times.
Here’s a general outline of the top-down approach:
- Start with the Original Problem: Identify the problem you want to solve.
- Divide the Problem: Break down the original problem into one or more smaller subproblems.
- Solve Subproblems Recursively: Solve the subproblems by recursively applying the same algorithm to each subproblem.
- Combine Solutions: Combine the solutions of the subproblems to solve the original problem.
- Base Cases: Include base cases or stopping conditions to end the recursion and solve the simplest cases directly.
- Optimize with Memoization: To avoid redundant calculations, use memoization (caching) to store solutions to subproblems that have already been solved.
Here’s a simple example using the top-down approach to calculate the nth Fibonacci number with memoization:
#include <iostream>
#include <vector>
std::vector<int> memo;
int fibonacci(int n) {
if (n <= 1) {
return n; // Base cases
}
if (memo[n] != -1) {
return memo[n]; // Use cached solution
}
memo[n] = fibonacci(n - 1) + fibonacci(n - 2); // Solve subproblems
return memo[n]; // Solution to the original problem
}
int main() {
int n = 6;
memo.resize(n + 1, -1); // Initialize memoization table
std::cout << "Fibonacci(" << n << ") = " << fibonacci(n) << std::endl;
return 0;
}
In this example, the top-down approach uses recursion to break down the problem of calculating the Fibonacci sequence into smaller subproblems. Memoization is used to store solutions to subproblems in the memo
vector, preventing redundant calculations. This approach provides efficient and optimized computation of Fibonacci numbers compared to a naive recursive solution.