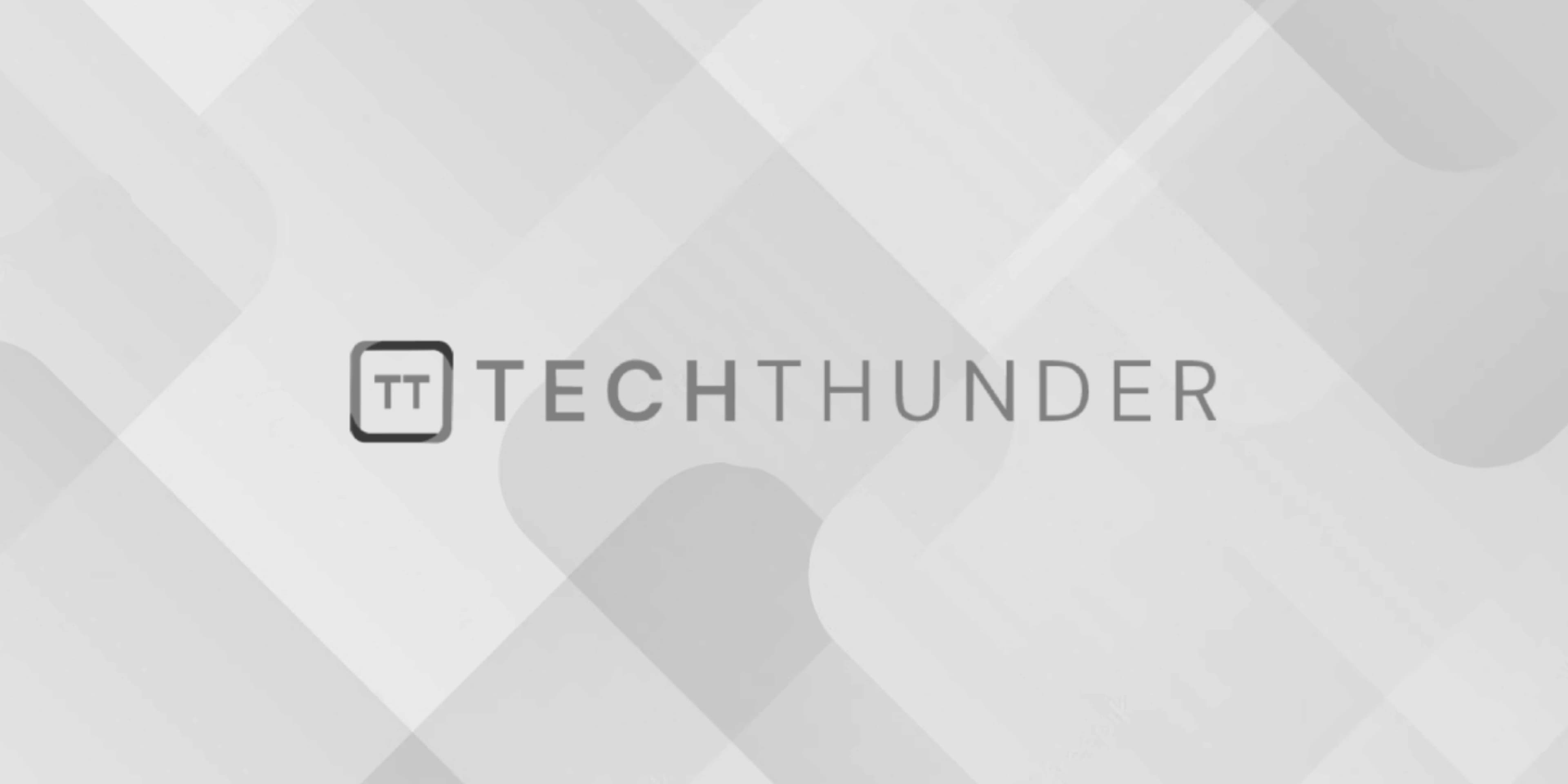
forward_list::cbefore_begin() in C++ STL
The C++ STL (Standard Template Library) std::forward_list
is a singly linked list container that provides constant time insertions and erasures at the beginning of the list. forward_list::cbefore_begin()
is a member function of std::forward_list
that returns a constant iterator pointing to the position just before the first element in the forward list. This iterator is “const,” which means you can use it to access elements and traverse the list, but you cannot modify the elements through this iterator.
Here’s an example of how to use forward_list::cbefore_begin()
:
#include <iostream>
#include <forward_list>
int main() {
std::forward_list<int> myList = {1, 2, 3, 4};
// Using cbegin() to get a constant iterator pointing to the position before the first element.
std::forward_list<int>::const_iterator it = myList.cbefore_begin();
// Insert an element at the beginning of the list using the constant iterator.
myList.insert_after(it, 0);
// Print the elements in the forward_list.
for (const int& value : myList) {
std::cout << value << " ";
}
return 0;
}
In this example, we first create a std::forward_list
called myList
containing some elements. We then use cbefore_begin()
to obtain a constant iterator it
pointing to the position just before the first element. We insert a new element with the value 0
at the beginning of the list using insert_after()
with the constant iterator.
The result will be that 0
is inserted at the beginning of the list, and the elements in the forward_list
are printed, resulting in the output: 0 1 2 3 4
.