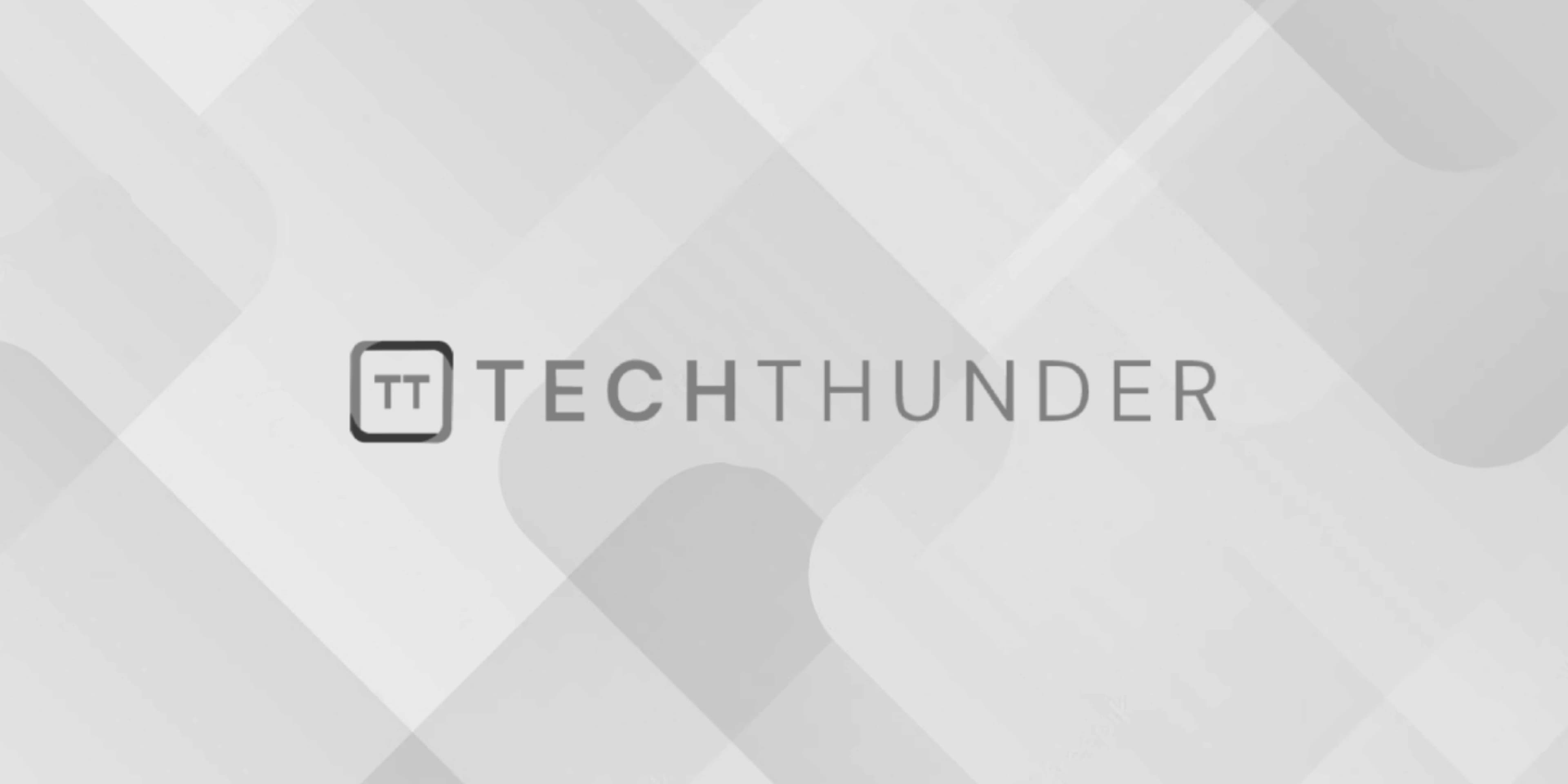
258 views
C++ Bidirectional Iterator
The C++ Bidirectional Iterator is an iterator category that allows traversal in both forward and backward directions through a sequence. It provides more functionality compared to Input and Output Iterators and is typically used with data structures that support bidirectional traversal, such as doubly linked lists.
Bidirectional Iterators have the following characteristics:
- Increment and Decrement: You can increment (
++
) and decrement (--
) a Bidirectional Iterator to move it forward and backward in the sequence. This allows for efficient bidirectional traversal. - Multiple Passes: Bidirectional Iterators support multiple passes through the sequence, meaning you can traverse the same sequence more than once.
- Dereferencing: You can dereference a Bidirectional Iterator (
*
) to access the value it points to. This allows you to read and modify the elements in the sequence. - Equality Comparison: Bidirectional Iterators support equality comparison (
==
and!=
) to determine if two iterators point to the same position in the sequence.
Here’s an example of using a Bidirectional Iterator with a std::list
(a doubly linked list) in C++:
C++
#include <iostream>
#include <list>
int main() {
std::list<int> myList = {1, 2, 3, 4, 5};
// Create a Bidirectional Iterator pointing to the beginning of the list
std::list<int>::iterator it = myList.begin();
// Forward traversal
while (it != myList.end()) {
std::cout << *it << " "; // Dereference to access the value
++it; // Move to the next element
}
std::cout << std::endl;
// Backward traversal
std::list<int>::reverse_iterator rit = myList.rbegin(); // Reverse iterator for backward traversal
while (rit != myList.rend()) {
std::cout << *rit << " ";
++rit; // Move to the previous element
}
std::cout << std::endl;
return 0;
}
In this example:
- We use a Bidirectional Iterator (
it
) to traverse thestd::list
in both the forward and backward directions. - The
std::list
container supports efficient bidirectional traversal because it’s implemented as a doubly linked list.
Bidirectional Iterators are useful when you need to traverse data structures like doubly linked lists and when you want the flexibility to move in both directions through a sequence.