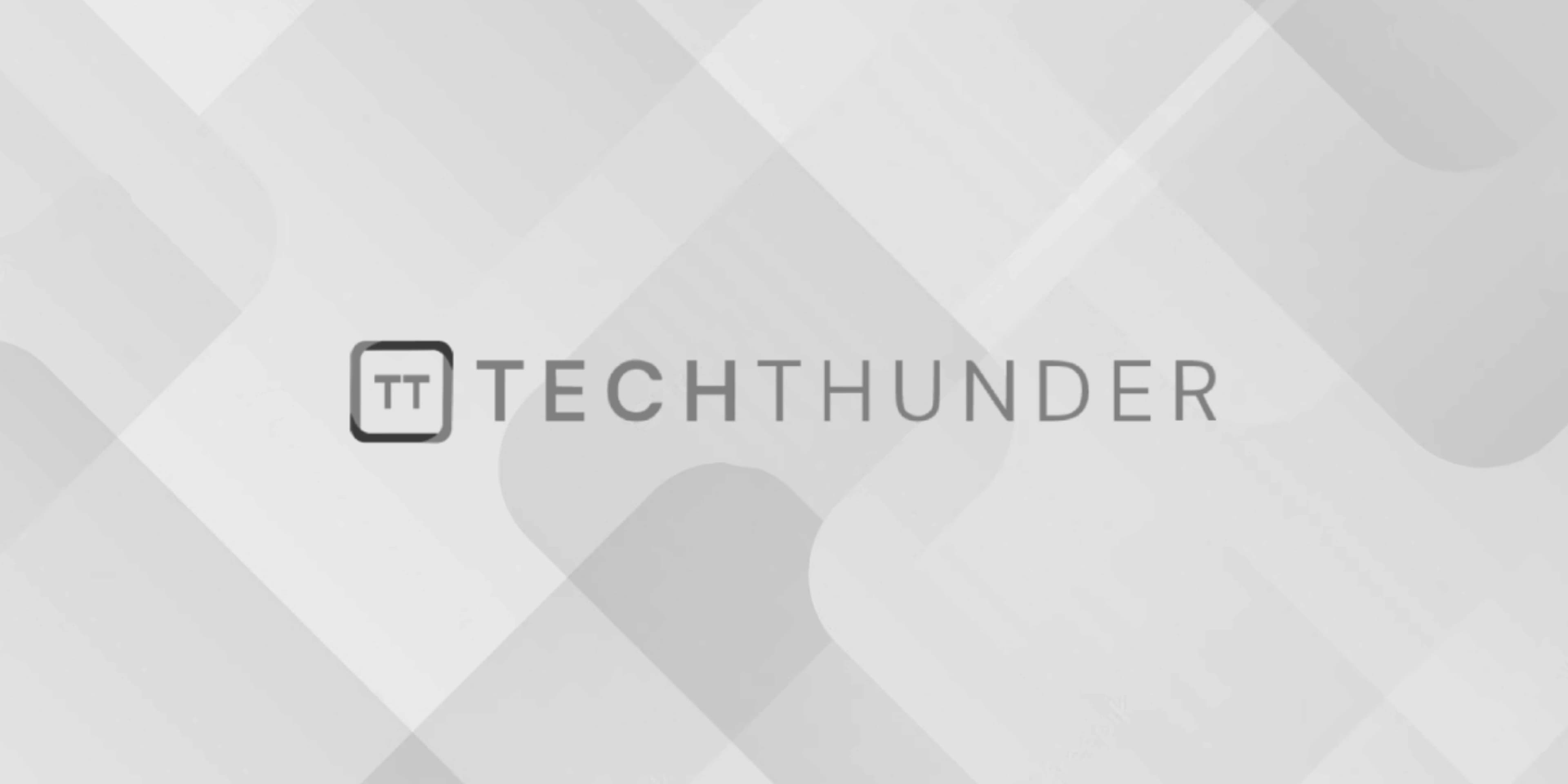
171 views
Type Casting in C++
Type casting in C++ is the process of converting a value from one data type to another. C++ provides several ways to perform type casting, depending on the situation and the desired outcome. There are mainly four types of type casting in C++:
- Static Cast: The
static_cast
operator is the most common and safest way to perform type casting in C++. It can be used for most type conversions that do not involve pointers to classes with virtual functions. Static cast is checked at compile-time whenever possible.
C++
double x = 3.14;
int y = static_cast<int>(x); // Converts double to int
- Dynamic Cast: The
dynamic_cast
operator is used for safe downcasting of pointers in the context of polymorphism (when you have a base class pointer to a derived class object). It checks the validity of the cast at runtime and returns a null pointer if the cast is not valid for a given object.
C++
class Base { virtual void foo() {} };
class Derived : public Base { };
Base* basePtr = new Derived();
Derived* derivedPtr = dynamic_cast<Derived*>(basePtr); // Safe downcast
if (derivedPtr != nullptr) {
// Successfully casted to Derived
}
- Reinterpret Cast: The
reinterpret_cast
operator is used to perform low-level type casting. It converts any pointer type to any other pointer type, and it can also be used for converting pointers to integers and vice versa. It should be used with caution because it can lead to undefined behavior if used incorrectly.
C++
int x = 42;
float* floatPtr = reinterpret_cast<float*>(&x); // Converts int* to float*
- Const Cast: The
const_cast
operator is used to add or remove theconst
qualifier from a pointer or reference. It should be used carefully, as modifying aconst
object may lead to undefined behavior.
C++
const int value = 10;
int* nonConstPtr = const_cast<int*>(&value); // Removes const from value
*nonConstPtr = 20; // Modifying a const variable (use with caution)
Remember that type casting should be used judiciously and with care. It’s important to understand the implications and potential risks associated with type casting, especially when working with pointers and references. In many cases, it’s better to design your code to avoid the need for explicit type casting when possible.