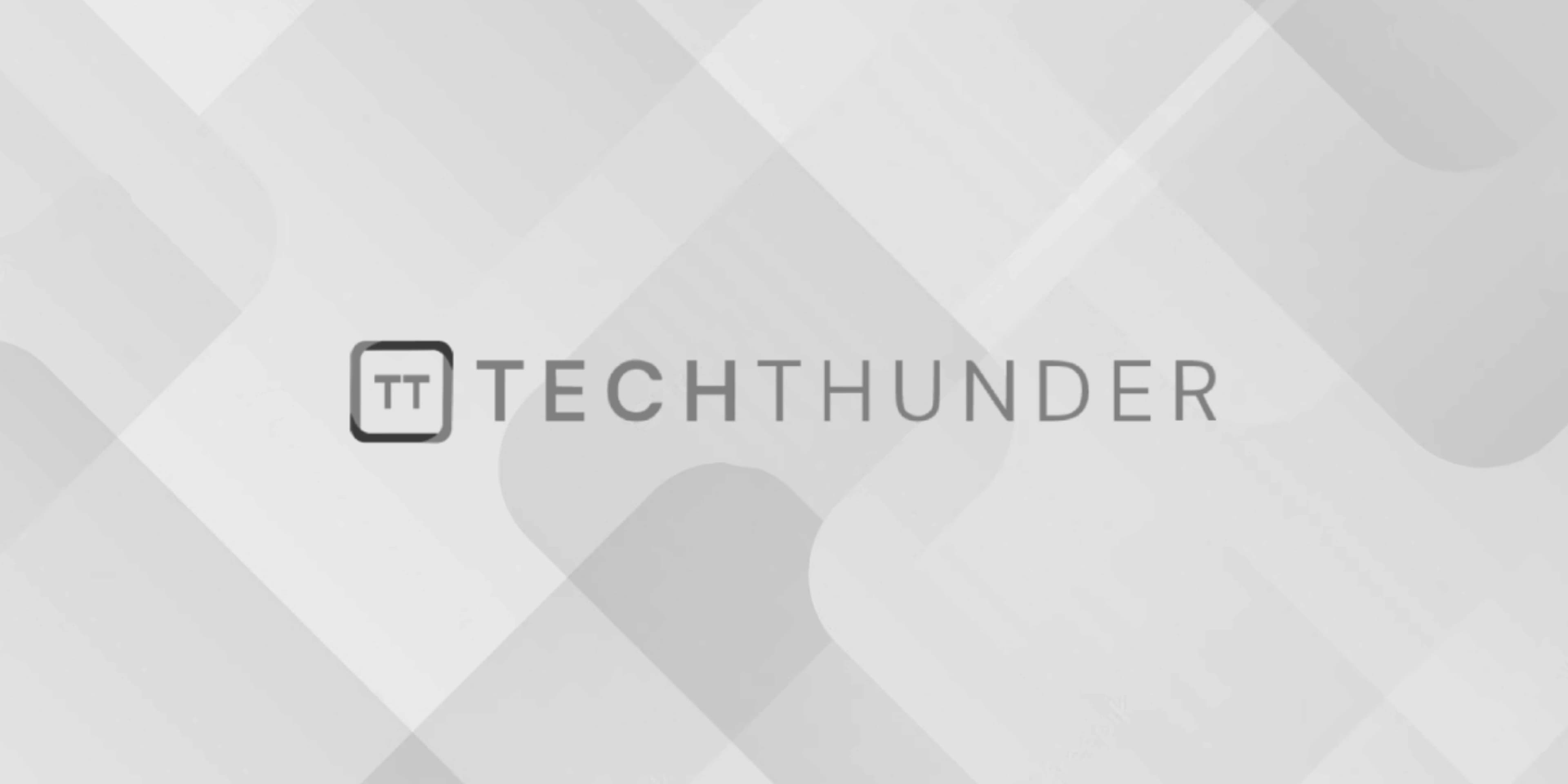
What is runtime type information in C++
Runtime Type Information (RTTI) in C++ is a feature that allows you to obtain information about the type of objects during runtime, as opposed to compile-time type information, which is determined at compile time. RTTI provides mechanisms for checking the actual dynamic type of an object and performing actions based on that type.
The primary components of RTTI in C++ include:
typeid
operator: Thetypeid
operator allows you to obtain information about the type of an object at runtime. It returns a reference to atype_info
object that represents the type of the object. You can use this information to perform type checking or type-safe casting. Example of usingtypeid
:
#include <typeinfo>
class Base {
virtual void print() {}
};
class Derived : public Base {
};
int main() {
Base* ptr = new Derived;
if (typeid(*ptr) == typeid(Derived)) {
std::cout << "Derived type matched." << std::endl;
}
delete ptr;
return 0;
}
dynamic_cast
operator: Thedynamic_cast
operator is used for safe downcasting (converting a base class pointer/reference to a derived class pointer/reference) by checking the object’s type during runtime. If the object is of the specified type, it returns a valid pointer; otherwise, it returns a null pointer. Example of usingdynamic_cast
:
Base* basePtr = new Derived;
Derived* derivedPtr = dynamic_cast<Derived*>(basePtr);
if (derivedPtr != nullptr) {
// Successfully downcasted to Derived.
derivedPtr->doDerivedOperation();
}
type_info
class: Thetype_info
class, defined in the<typeinfo>
header, is used to represent type information. You can comparetype_info
objects obtained throughtypeid
to determine whether two objects share the same type.
RTTI is a powerful feature, but it should be used with care as it introduces some runtime overhead and is generally avoided in high-performance code where compile-time polymorphism (e.g., virtual functions) is preferred. It is most commonly used in situations where you need to perform type-specific operations or implement complex dynamic behaviors, such as object serialization, plugin systems, or reflection.