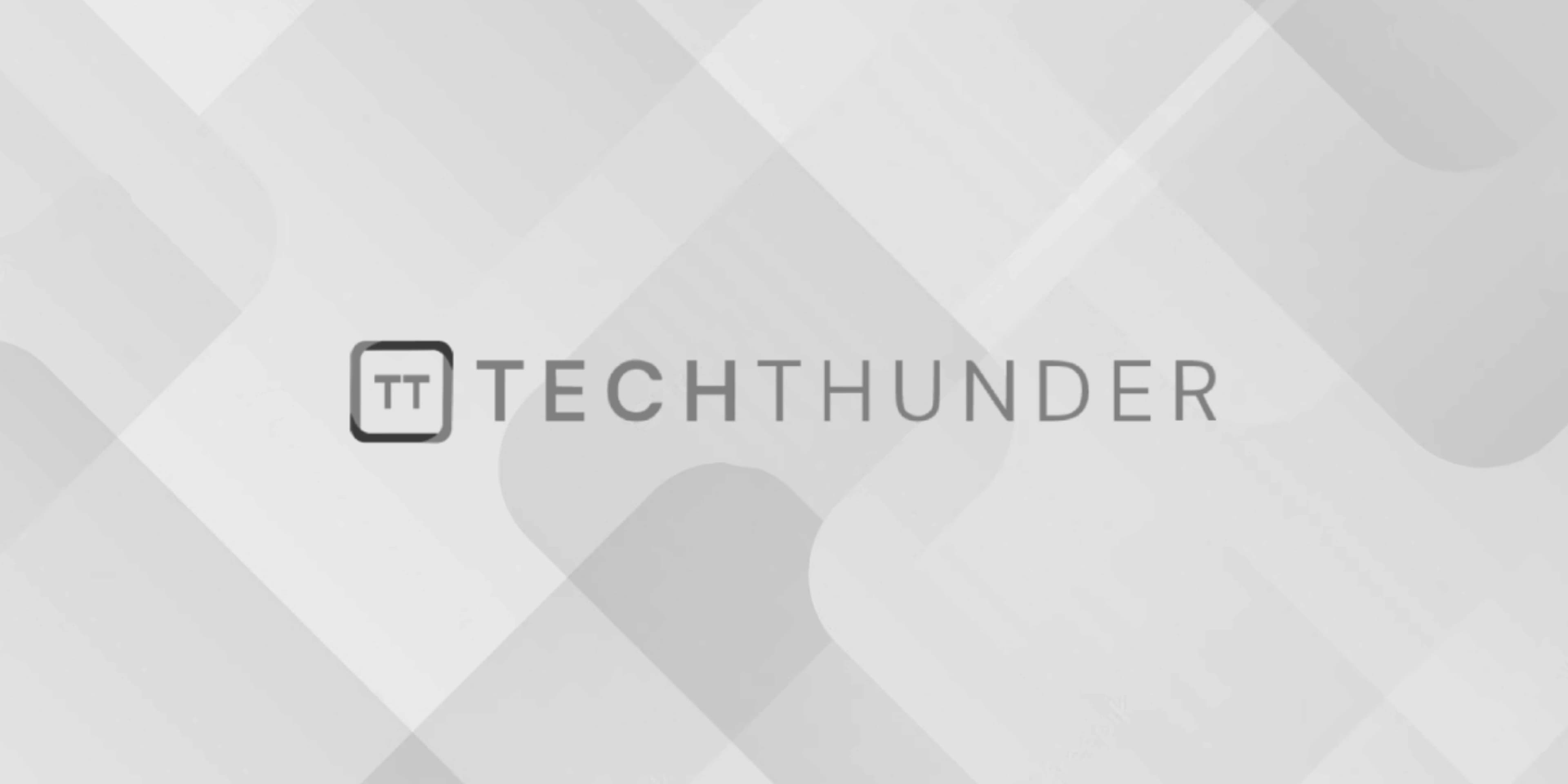
C++ Structs
The C++ struct (short for “structure”) is a composite data type that allows you to group together variables of different data types under a single name. Unlike classes, which can have member functions and support access control keywords (private
, protected
, public
), structs are typically used for simple data structures where the focus is on grouping related data rather than encapsulating behavior.
Here’s how you define and use a struct in C++:
struct Point {
int x;
int y;
};
In this example, we’ve defined a struct called Point
with two data members: x
and y
. Each instance of the Point
struct can hold two integers to represent a point’s coordinates.
You can create instances (objects) of the struct and access its members as follows:
Point p1; // Declare an instance of the Point struct
p1.x = 5; // Access and set the x coordinate
p1.y = 10; // Access and set the y coordinate
// Access and print the coordinates
std::cout << "x: " << p1.x << ", y: " << p1.y << std::endl;
Key points about C++ structs:
- Member Variables: A struct defines its member variables inside its body. These variables can have different data types and are accessible directly without the need for accessor methods (getters and setters).
- Public Access: By default, all members of a struct are
public
, which means they can be accessed and modified from outside the struct. There’s no access control likeprivate
orprotected
in classes. - Initialization: You can initialize struct members when declaring a struct variable.
Point p2 = {3, 7}; // Initializing members at the time of declaration
- Array of Structs: You can create arrays or collections of structs to represent multiple instances of the same structure.
Point points[10]; // Array of 10 Point structs
points[0].x = 1;
points[0].y = 2;
- Passing Structs to Functions: You can pass structs to functions by value or by reference, just like any other data type.
void printPoint(const Point& p) {
std::cout << "x: " << p.x << ", y: " << p.y << std::endl;
}
- C++ vs. C: In C, structs are primarily used for data grouping, while classes are used for encapsulating data and behavior. In C++, structs and classes are quite similar, but classes have access control features, whereas structs have public members by default.
- Naming Conventions: Typically, struct names are in TitleCase, similar to class names. Member variables of structs often follow a lowerCamelCase naming convention.
Structs are a simple and effective way to group related data together in C++, making them a useful tool for representing objects with just data and no behavior. They are commonly used in scenarios where data needs to be aggregated and passed around as a single unit.