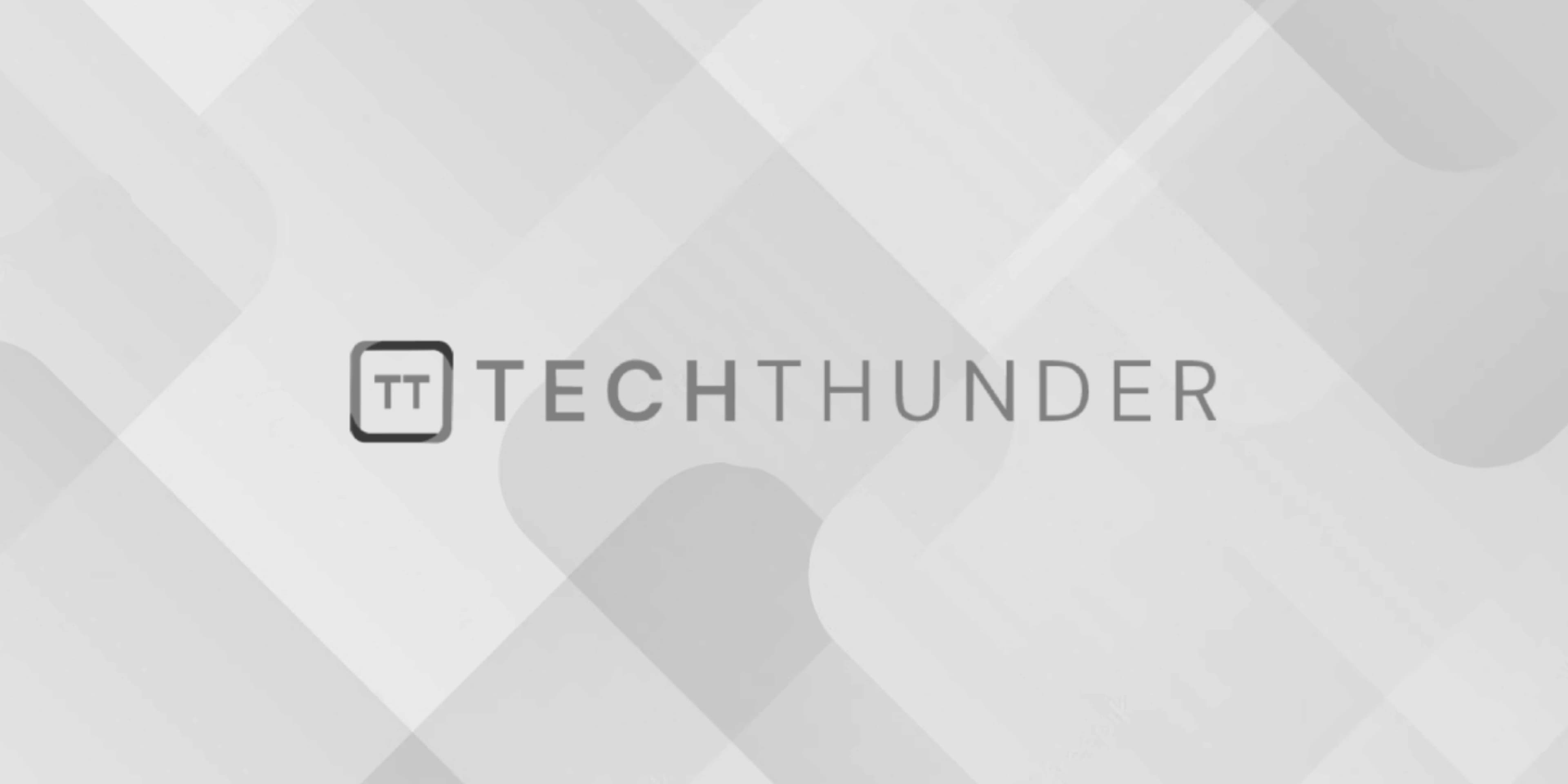
160 views
C++ Forward Iterator
The C++ Forward Iterator is an iterator category that allows you to traverse a sequence of elements in one direction, typically forward. Forward Iterators provide basic iterator functionality, including reading and modifying elements, but they don’t support operations like moving backward or random access.
Here are the key characteristics of Forward Iterators:
- Increment: You can use the
++
operator to increment a Forward Iterator, moving it to the next element in the sequence. This operation is well-defined and makes it suitable for single-pass traversal. - Dereferencing: You can use the
*
operator to dereference a Forward Iterator and access the value it points to. This allows you to read and modify the elements in the sequence. - Single-Pass: Forward Iterators are designed for single-pass traversal, meaning you can go through the sequence once but can’t efficiently move backward or perform random access operations.
- Equality Comparison: Forward Iterators support equality comparison (
==
and!=
) to check if two iterators point to the same position in the sequence.
Here’s a simple example of using a Forward Iterator with a std::forward_list
(a singly linked list) in C++:
C++
#include <iostream>
#include <forward_list>
int main() {
std::forward_list<int> myList = {1, 2, 3, 4, 5};
// Create a Forward Iterator pointing to the beginning of the list
std::forward_list<int>::iterator it = myList.begin();
// Traverse the list using the Forward Iterator
while (it != myList.end()) {
std::cout << *it << " "; // Dereference to access the value
++it; // Move to the next element
}
std::cout << std::endl;
return 0;
}
In this example:
- We use a Forward Iterator (
it
) to traverse astd::forward_list
, which is implemented as a singly linked list. - The Forward Iterator allows us to move forward through the list using
++
and access the elements using*
.
While Forward Iterators are less powerful than Bidirectional and Random Access Iterators in terms of traversal capabilities, they are still useful in scenarios where you only need to traverse a sequence once and do not require backward movement or random access.