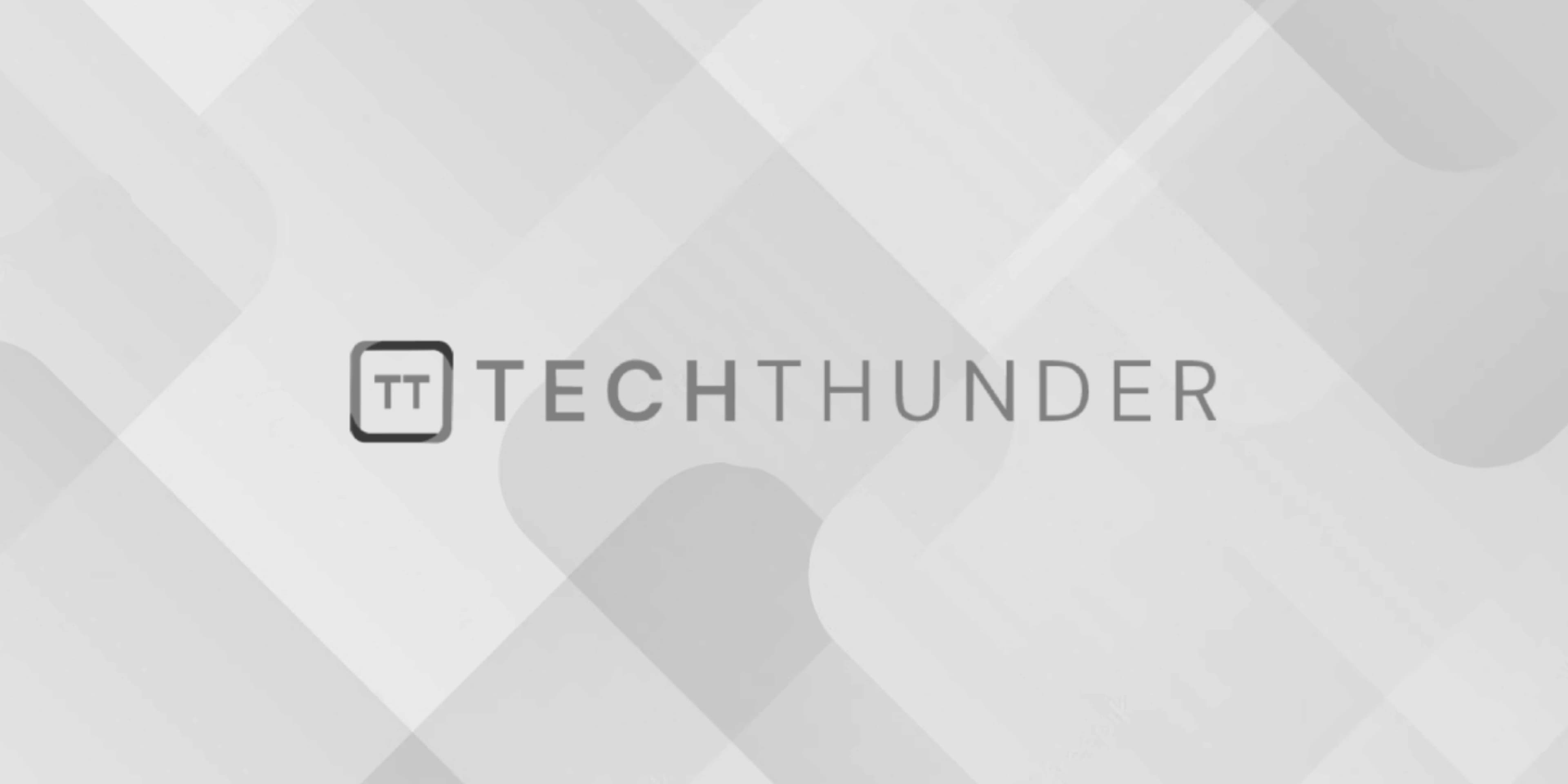
C++ Array to Function
The C++, you can pass an array to a function in several ways. When passing an array to a function, it’s essential to understand that arrays are treated as pointers to their first elements. Here are the common methods to pass an array to a function:
Method 1: Pass by Pointer
You can pass an array to a function by passing a pointer to its first element. This method allows the function to modify the original array.
void printArray(int* arr, int size) {
for (int i = 0; i < size; ++i) {
std::cout << arr[i] << " ";
}
std::cout << std::endl;
}
int main() {
int myArray[] = {1, 2, 3, 4, 5};
int size = sizeof(myArray) / sizeof(myArray[0]);
printArray(myArray, size);
return 0;
}
Method 2: Pass by Reference
You can also pass an array by reference to a function, which allows the function to modify the original array. This is often preferred in C++.
void modifyArray(int (&arr)[5]) {
for (int i = 0; i < 5; ++i) {
arr[i] *= 2;
}
}
int main() {
int myArray[] = {1, 2, 3, 4, 5};
modifyArray(myArray);
for (int i = 0; i < 5; ++i) {
std::cout << myArray[i] << " ";
}
std::cout << std::endl;
return 0;
}
Method 3: Use a Pointer and Specify Array Size
You can pass an array by using a pointer along with specifying the array size as a separate parameter.
void printArray(int* arr, int size) {
for (int i = 0; i < size; ++i) {
std::cout << arr[i] << " ";
}
std::cout << std::endl;
}
int main() {
int myArray[] = {1, 2, 3, 4, 5};
int size = sizeof(myArray) / sizeof(myArray[0]);
printArray(myArray, size);
return 0;
}
Method 4: Use std::array
or std::vector
In modern C++, you can use std::array
or std::vector
from the C++ Standard Library, which provide better safety and flexibility compared to traditional C-style arrays. These containers can be passed to functions like other data types.
#include <iostream>
#include <array>
#include <vector>
void printArray(const std::array<int, 5>& arr) {
for (int element : arr) {
std::cout << element << " ";
}
std::cout << std::endl;
}
void printVector(const std::vector<int>& vec) {
for (int element : vec) {
std::cout << element << " ";
}
std::cout << std::endl;
}
int main() {
std::array<int, 5> myArray = {1, 2, 3, 4, 5};
std::vector<int> myVector = {1, 2, 3, 4, 5};
printArray(myArray);
printVector(myVector);
return 0;
}
Using std::array
or std::vector
is often recommended in modern C++ because they provide better safety and maintainability compared to raw arrays.