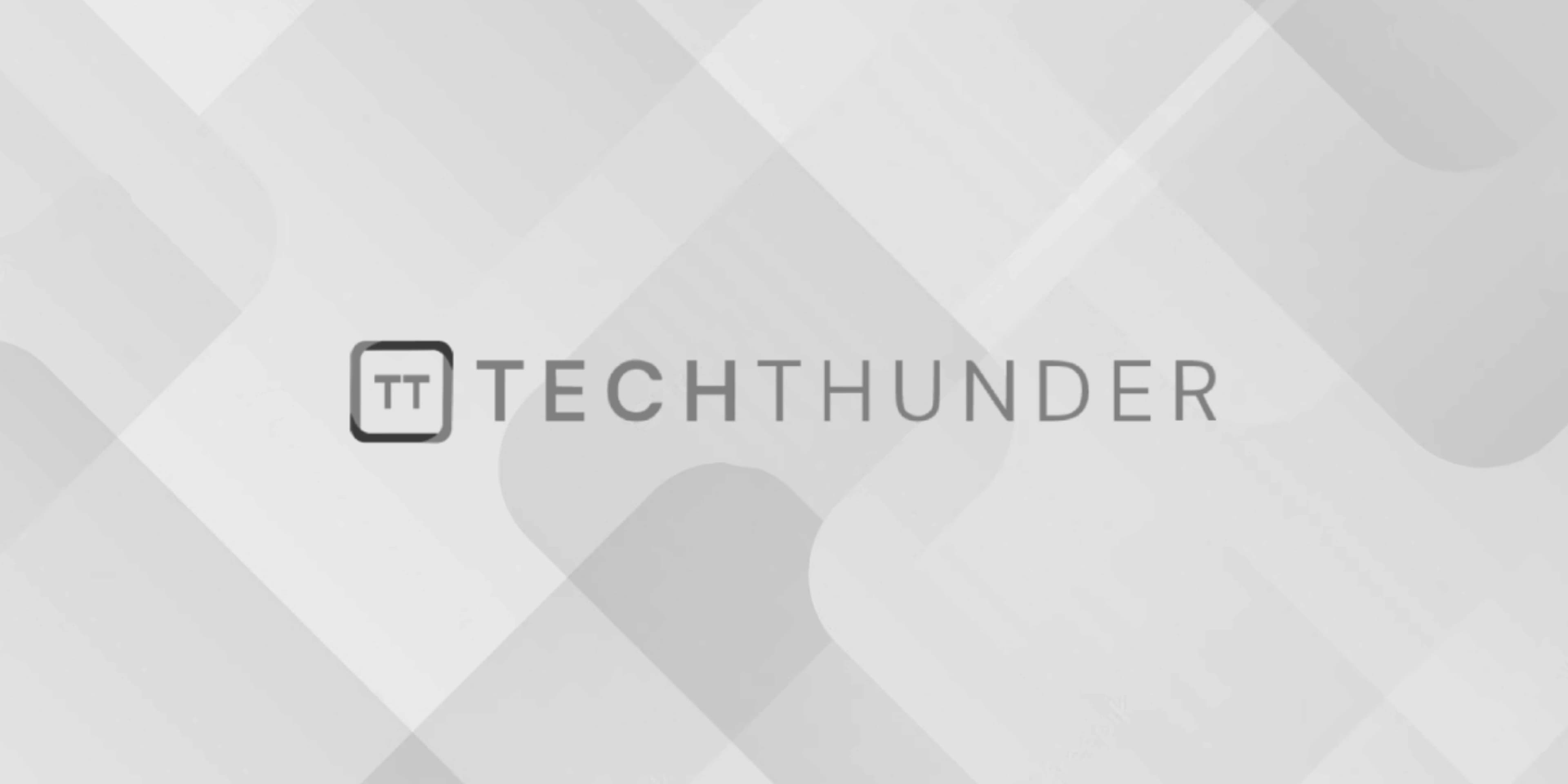
213 views
Scope Resolution Operator vs this Pointer in C++
The C++ scope resolution operator (::
) and the this
pointer serve different purposes and are used in different contexts. Let’s explore their roles and differences:
- Scope Resolution Operator (
::
):
- The
::
operator is primarily used for accessing global variables, class-level variables, and static members of a class. - It allows you to specify the scope from which a particular variable or function should be accessed, especially when there is a name conflict or when you want to access members of a different scope.
- It is not specific to object instances and is used at the class or global scope.
- It is often used to access static class members or to define and implement functions outside the class definition. Example of using
::
to access a static member:
C++
class MyClass {
public:
static int myStaticVar;
};
int MyClass::myStaticVar = 42; // Definition of the static member
int main() {
int globalVar = 10;
int localVar = 5;
MyClass::myStaticVar = 20; // Accessing a static member with ::
return 0;
}
this
Pointer:
- The
this
pointer is a pointer that is implicitly available within non-static member functions of a class. It points to the current object for which the member function is called. - It is used to access or modify the data members (variables) of the current instance of the class.
- It is primarily used for disambiguating between member variables and function parameters when they have the same name within a member function.
- The
this
pointer is automatically dereferenced, so you can access class members directly using the arrow operator (->
) or with the dot operator (.
). Example of using thethis
pointer:
C++
class MyClass {
private:
int myVar;
public:
void setVar(int myVar) {
this->myVar = myVar; // Use this pointer to access the member variable.
}
};
In summary, the scope resolution operator ::
is used to access class-level and global entities, while the this
pointer is used within member functions to access instance-specific data members. They serve different purposes and are not interchangeable.