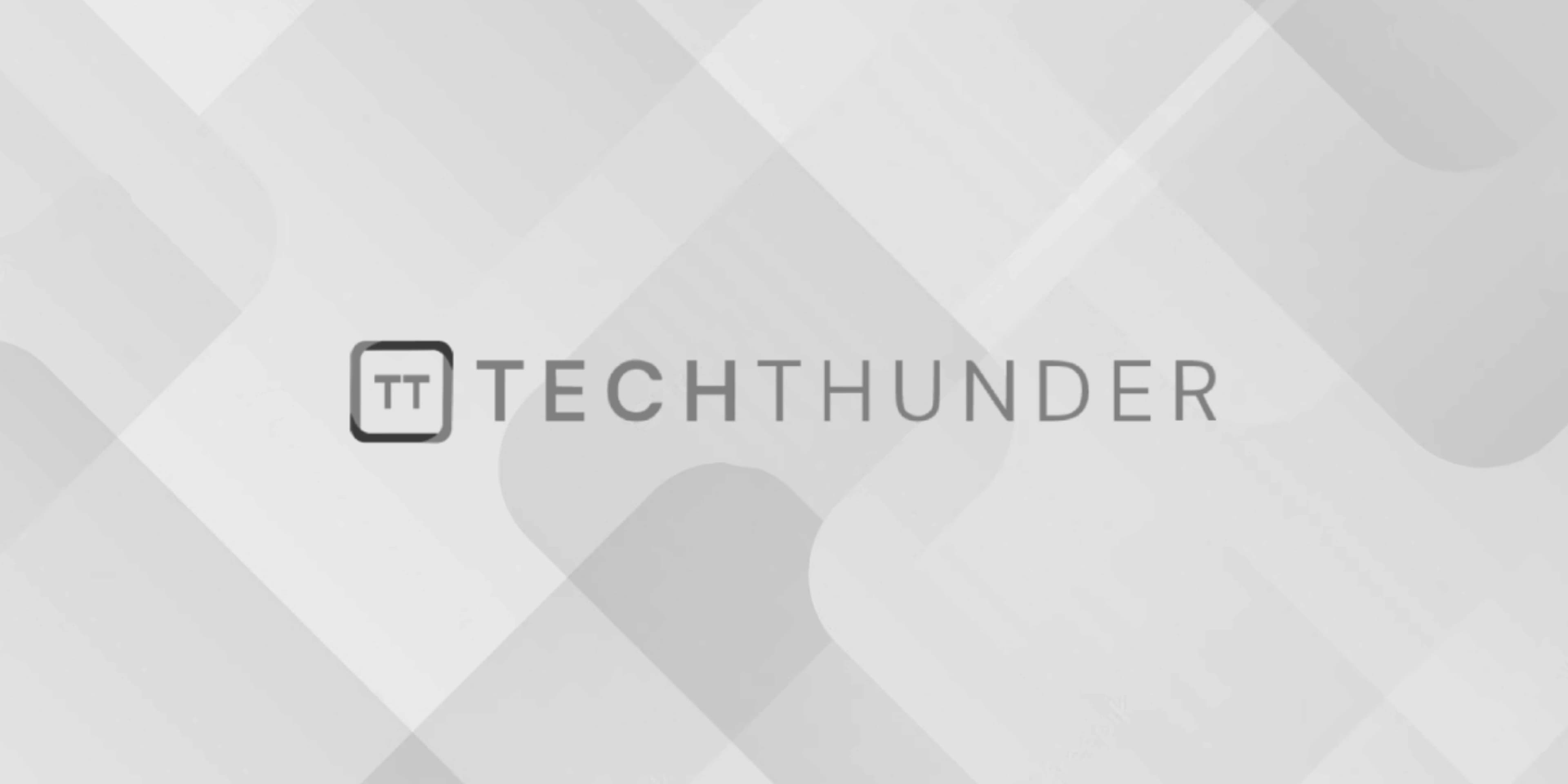
144 views
C++ STL Components
The C++ Standard Library (STL) is a powerful collection of C++ templates to provide general-purpose classes and functions with templates that implement many popular and commonly used algorithms and data structures. The STL components can be broadly categorized into the following sections:
- Containers:
- Containers are classes or data structures that hold collections of objects.
- Common container types in STL include:
- Vector: A dynamic array that can grow or shrink in size.
- List: A doubly-linked list.
- Deque: A double-ended queue.
- Set: A collection of unique, sorted elements.
- Map: A collection of key-value pairs.
- Stack: A Last-In-First-Out (LIFO) data structure.
- Queue: A First-In-First-Out (FIFO) data structure.
- Containers have various methods for insertion, deletion, and access to elements.
- Iterators:
- Iterators are used to traverse and manipulate elements in containers.
- They provide a uniform way to access elements regardless of the container type.
- Examples include
begin()
andend()
iterators for container traversal.
- Algorithms:
- The STL includes a wide range of algorithms that operate on containers.
- These algorithms include searching, sorting, and modifying functions.
- Examples include
sort()
,find()
,for_each()
, andtransform()
.
- Function Objects (Functors):
- Functors are objects that can be invoked like functions.
- They are often used with algorithms to customize their behavior.
- Functors can be defined as classes that overload the
operator()
function.
- Allocator:
- The allocator is responsible for memory management for STL containers.
- You can customize memory allocation strategies using allocators.
- Utilities:
- STL provides various utility classes and functions.
- Examples include
pair
(a simple structure for holding two values) andtuple
(a more general structure for holding multiple values).
- Strings:
- The
string
class provides a convenient way to work with strings in C++. - It includes various member functions for string manipulation.
- Streams:
- The
iostream
library provides input and output streams. cin
andcout
are standard streams for console input and output.- File streams (
ifstream
andofstream
) are used for file I/O.
- Numeric and Math Functions:
- The
<cmath>
header provides mathematical functions likesin()
,cos()
,sqrt()
, etc. - The
<numeric>
header contains numeric algorithms likeaccumulate()
andinner_product()
.
- Concurrency and Parallelism:
- C++11 and later versions of the STL include features for multi-threading and parallelism, such as
std::thread
and the<thread>
header. - Parallel algorithms like
std::for_each
with execution policies (e.g.,std::execution::par
) are available for parallel processing.
- C++11 and later versions of the STL include features for multi-threading and parallelism, such as
- Smart Pointers:
- C++11 introduced smart pointers like
std::shared_ptr
,std::unique_ptr
, andstd::weak_ptr
to manage dynamic memory more efficiently and safely.
- C++11 introduced smart pointers like
- Regular Expressions:
- The
<regex>
header provides support for regular expressions, enabling pattern matching and text manipulation.
- The
These are the major components of the C++ Standard Library (STL) that make C++ a powerful and versatile language for a wide range of programming tasks. They help simplify common programming tasks by providing efficient and well-tested implementations of data structures and algorithms.