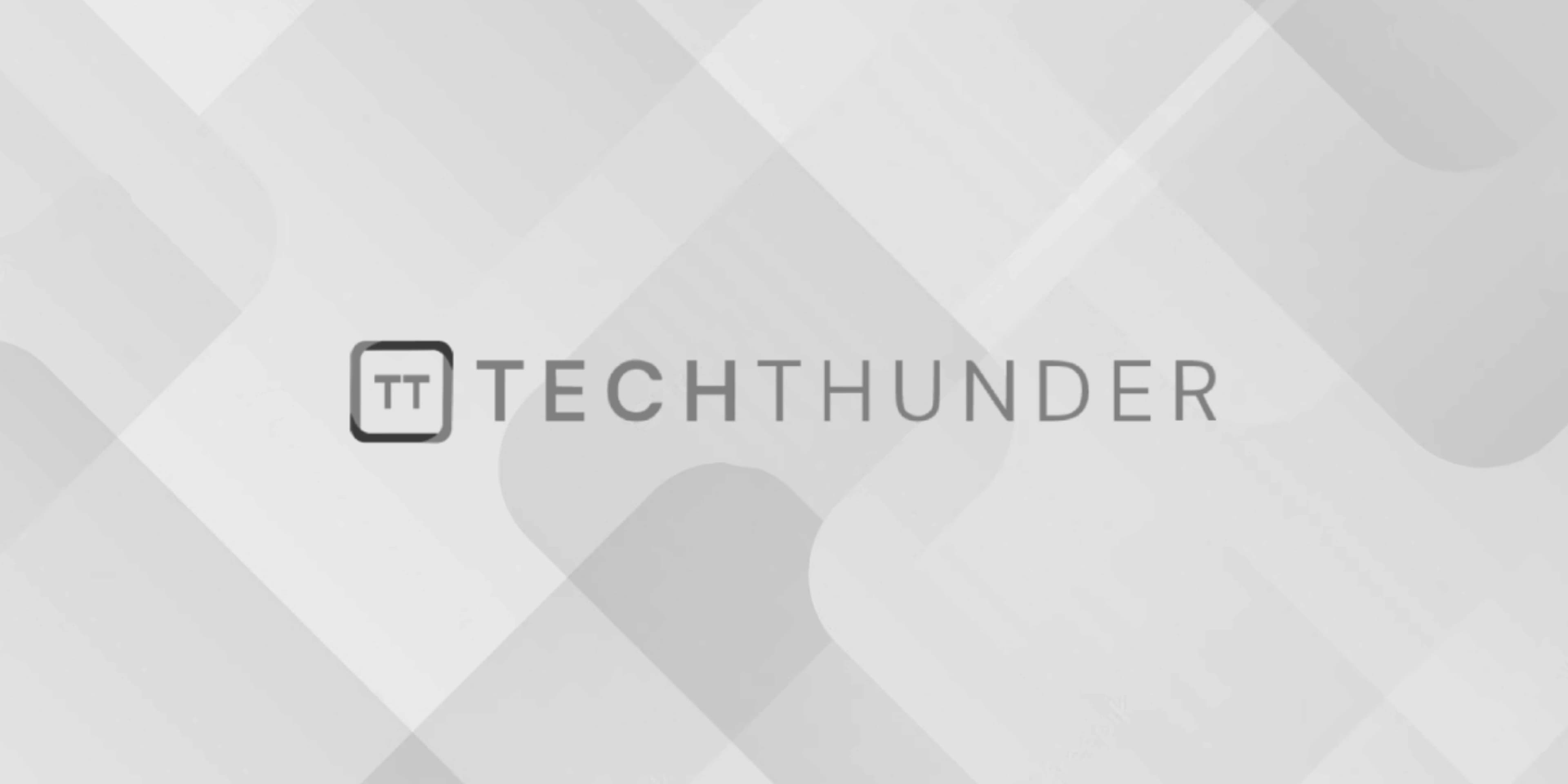
C++ try/catch
The C++ try
and catch
blocks are used for exception handling, allowing you to gracefully handle and recover from unexpected or exceptional situations that may arise during the execution of your program. Exception handling helps improve program robustness by providing a mechanism to deal with errors without causing the program to crash.
Here’s the basic syntax and usage of try
and catch
in C++:
try {
// Code that may throw an exception
// ...
}
catch (ExceptionType1 e1) {
// Handle ExceptionType1
}
catch (ExceptionType2 e2) {
// Handle ExceptionType2
}
// ...
catch (ExceptionTypeN eN) {
// Handle ExceptionTypeN
}
Here’s how exception handling works in C++:
- The code that may potentially throw an exception is placed within the
try
block. - If an exception occurs during the execution of the code within the
try
block, the program immediately jumps to the appropriatecatch
block based on the type of exception thrown. - Each
catch
block is associated with a specific exception type (ExceptionType1
,ExceptionType2
, etc.), and only onecatch
block is executed, depending on the type of exception thrown. - Once the exception is handled in a
catch
block, the program continues to execute the code after thetry-catch
construct.
Here’s an example demonstrating the use of try
and catch
to handle exceptions:
#include <iostream>
#include <stdexcept>
int main() {
try {
int num1, num2;
std::cout << "Enter two numbers: ";
std::cin >> num1 >> num2;
if (num2 == 0) {
throw std::runtime_error("Division by zero is not allowed.");
}
double result = static_cast<double>(num1) / num2;
std::cout << "Result: " << result << std::endl;
}
catch (std::runtime_error& e) {
std::cerr << "Error: " << e.what() << std::endl;
}
catch (...) {
std::cerr << "An unknown error occurred." << std::endl;
}
return 0;
}
In this example:
- The code within the
try
block attempts to perform division, and if the denominator (num2
) is zero, it throws astd::runtime_error
exception. - If the exception is thrown, the program jumps to the appropriate
catch
block based on the exception’s type. In this case, it goes to thecatch (std::runtime_error& e)
block, where the error message is printed. - If no exception is thrown, the program continues to execute the code after the
try-catch
block.
Remember that you can catch exceptions of various types and handle them accordingly. Additionally, it’s considered good practice to catch specific exception types rather than catching all exceptions with a generic catch (...)
block, as this allows for more precise error handling and debugging.