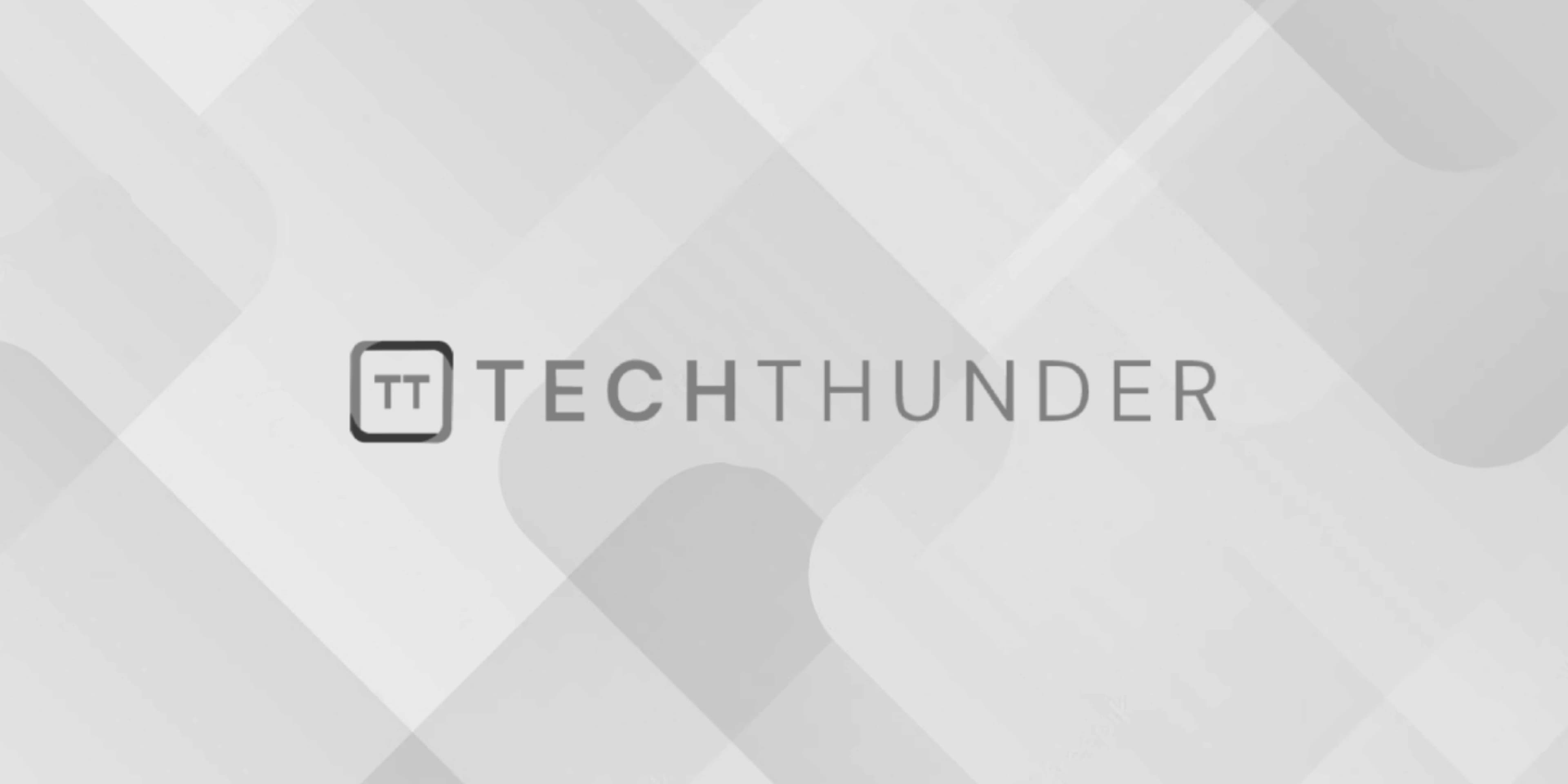
199 views
C++ Queue
The C++ queue is a linear data structure that follows the First-In-First-Out (FIFO) principle, meaning the element that is added first will be the one to be removed first. C++ provides a convenient container adapter called std::queue
in the Standard Library, which is implemented using other data structures, such as a deque (double-ended queue) by default.
Here’s how you can use std::queue
in C++:
C++
#include <iostream>
#include <queue>
int main() {
std::queue<int> myQueue;
// Enqueue elements
myQueue.push(10);
myQueue.push(20);
myQueue.push(30);
// Access the front element (the oldest element)
std::cout << "Front element: " << myQueue.front() << std::endl;
// Dequeue elements
myQueue.pop();
// Check if the queue is empty
if (myQueue.empty()) {
std::cout << "Queue is empty." << std::endl;
} else {
std::cout << "Queue is not empty." << std::endl;
}
// Get the size of the queue
std::cout << "Queue size: " << myQueue.size() << std::endl;
return 0;
}
In this example:
- We include the
<queue>
header to usestd::queue
. - We create a queue called
myQueue
to store integer values. - We use the
push
function to enqueue (add) elements to the queue. - The
front
function allows us to access the front (oldest) element without removing it. - We use the
pop
function to dequeue (remove) the front element. empty
checks whether the queue is empty.size
returns the number of elements in the queue.
std::queue
provides a simple and convenient way to work with queues in C++. If you need more control or specific features, you can also implement a queue using other data structures like a linked list or an array.