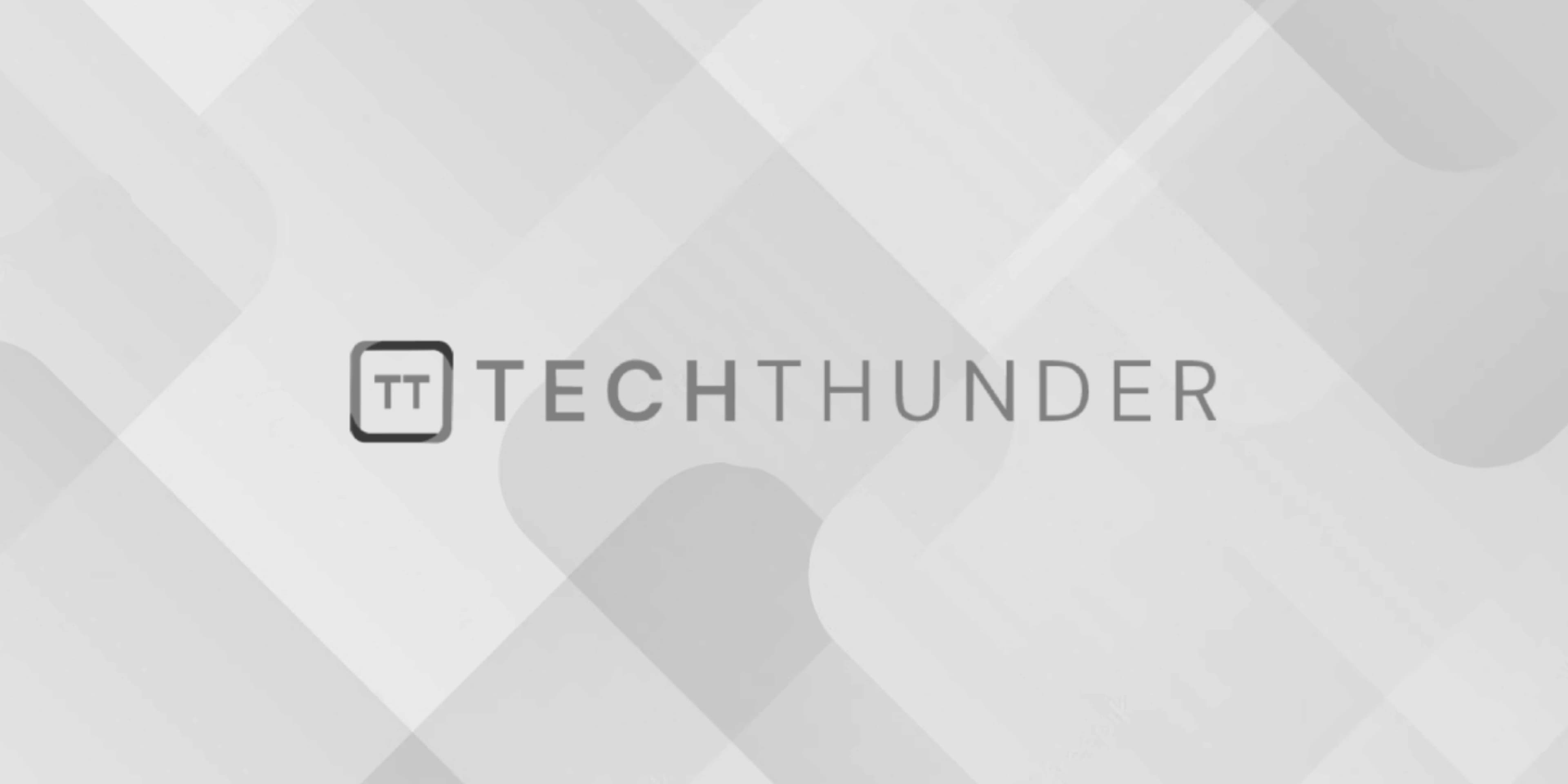
C++ List
The C++ list is a container data structure that allows you to store and manage a collection of elements. Unlike arrays, lists are dynamic in size and can grow or shrink as needed. The C++ Standard Library provides a doubly-linked list implementation through the std::list
container, which is part of the <list>
header.
Here’s a basic overview of how to use std::list
in C++:
- Include the necessary header:
#include <list>
- Declare a list and add elements to it:
std::list<int> myList; // Create an empty list of integers
myList.push_back(1); // Add an element to the end of the list
myList.push_back(2);
myList.push_front(0); // Add an element to the beginning of the list
- Access elements in the list:
You can iterate through the list using iterators, or you can directly access elements by their position using std::list::begin()
, std::list::end()
, and other member functions.
std::list<int>::iterator it;
for (it = myList.begin(); it != myList.end(); ++it) {
std::cout << *it << " ";
}
// Accessing elements by index is not efficient for lists
- Removing elements:
You can remove elements from the list using functions like std::list::pop_front()
, std::list::pop_back()
, or std::list::erase()
.
myList.pop_back(); // Remove the last element
myList.pop_front(); // Remove the first element
// Remove a specific element by value
myList.remove(2);
- Other common operations:
size()
: Returns the number of elements in the list.empty()
: Checks if the list is empty.sort()
: Sorts the elements in the list.reverse()
: Reverses the order of elements in the list.
if (!myList.empty()) {
myList.sort();
myList.reverse();
}
Remember that std::list
is a doubly-linked list, which means it allows for efficient insertions and deletions at both ends of the list. However, direct access to elements by index is slow, as you would need to traverse the list from the beginning or end to reach a specific element.
If you need random access to elements and want to take advantage of faster element access, you may want to consider other container types like std::vector
or std::deque
in C++.