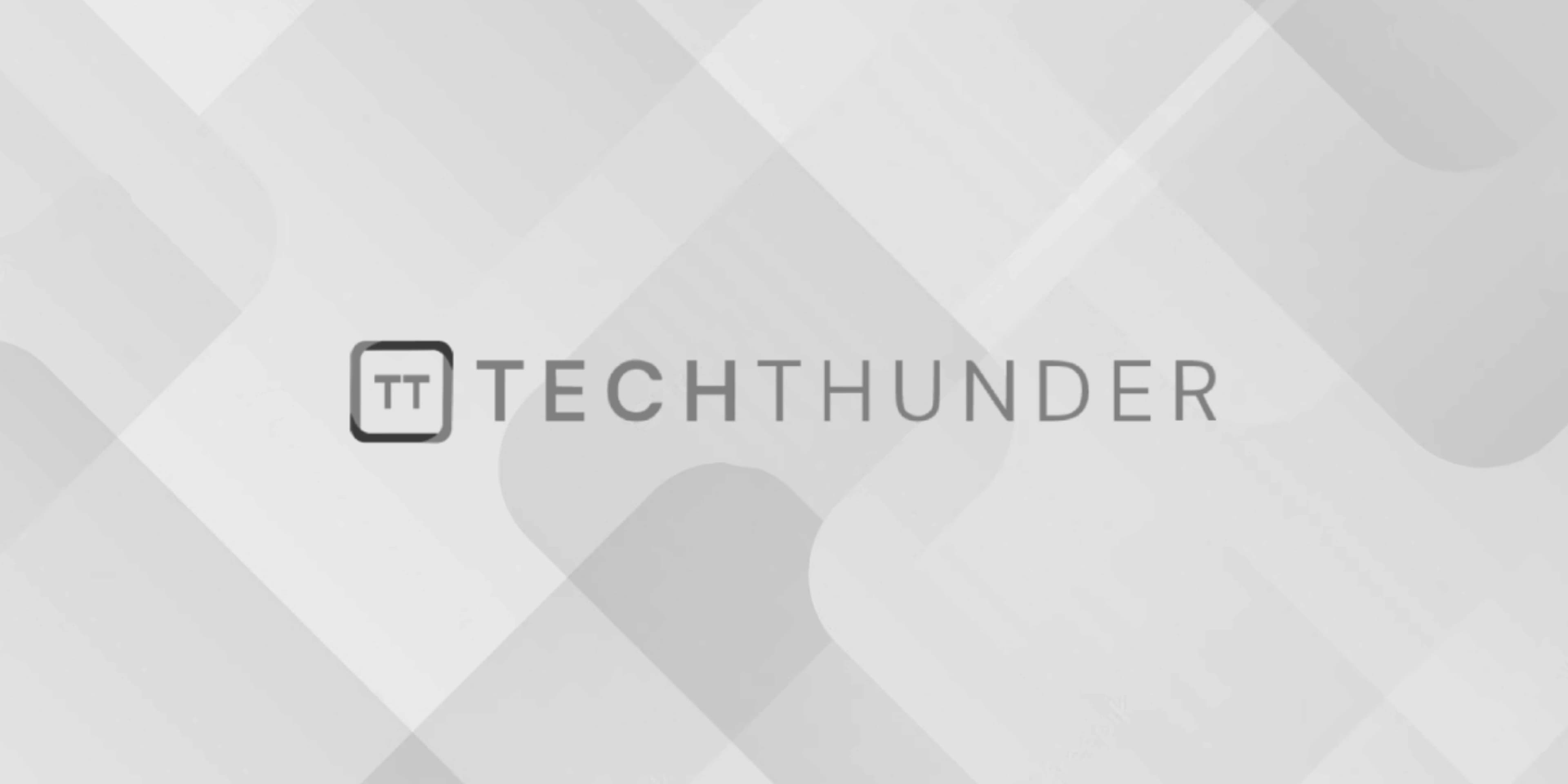
173 views
Stoi function in C++
The C++ std::stoi
function is part of the C++ Standard Library and is used to convert a string to an integer (int). It stands for “string to integer.” The std::stoi
function takes a string as input, parses it, and returns the corresponding integer value. Here’s how to use it:
C++
#include <iostream>
#include <string>
int main() {
std::string str = "12345"; // Input string containing an integer
try {
int intValue = std::stoi(str); // Convert the string to an integer
std::cout << "Integer value: " << intValue << std::endl;
} catch (const std::invalid_argument& e) {
std::cerr << "Invalid argument: " << e.what() << std::endl;
} catch (const std::out_of_range& e) {
std::cerr << "Out of range: " << e.what() << std::endl;
}
return 0;
}
In this example:
- We include the necessary headers,
<iostream>
and<string>
. - We have a string
str
containing the text representation of an integer. - We use a
try-catch
block to handle potential exceptions that may occur during the conversion. - Inside the
try
block, we callstd::stoi(str)
to convert the string to an integer. - If the conversion is successful, we print the integer value.
- If an invalid argument or an out-of-range value is encountered during the conversion, it will throw an exception, and we handle it in the
catch
blocks.
The std::stoi
function is a safe and convenient way to convert a string to an integer while also handling potential errors gracefully. It’s a commonly used function when dealing with string parsing and integer conversion in C++.