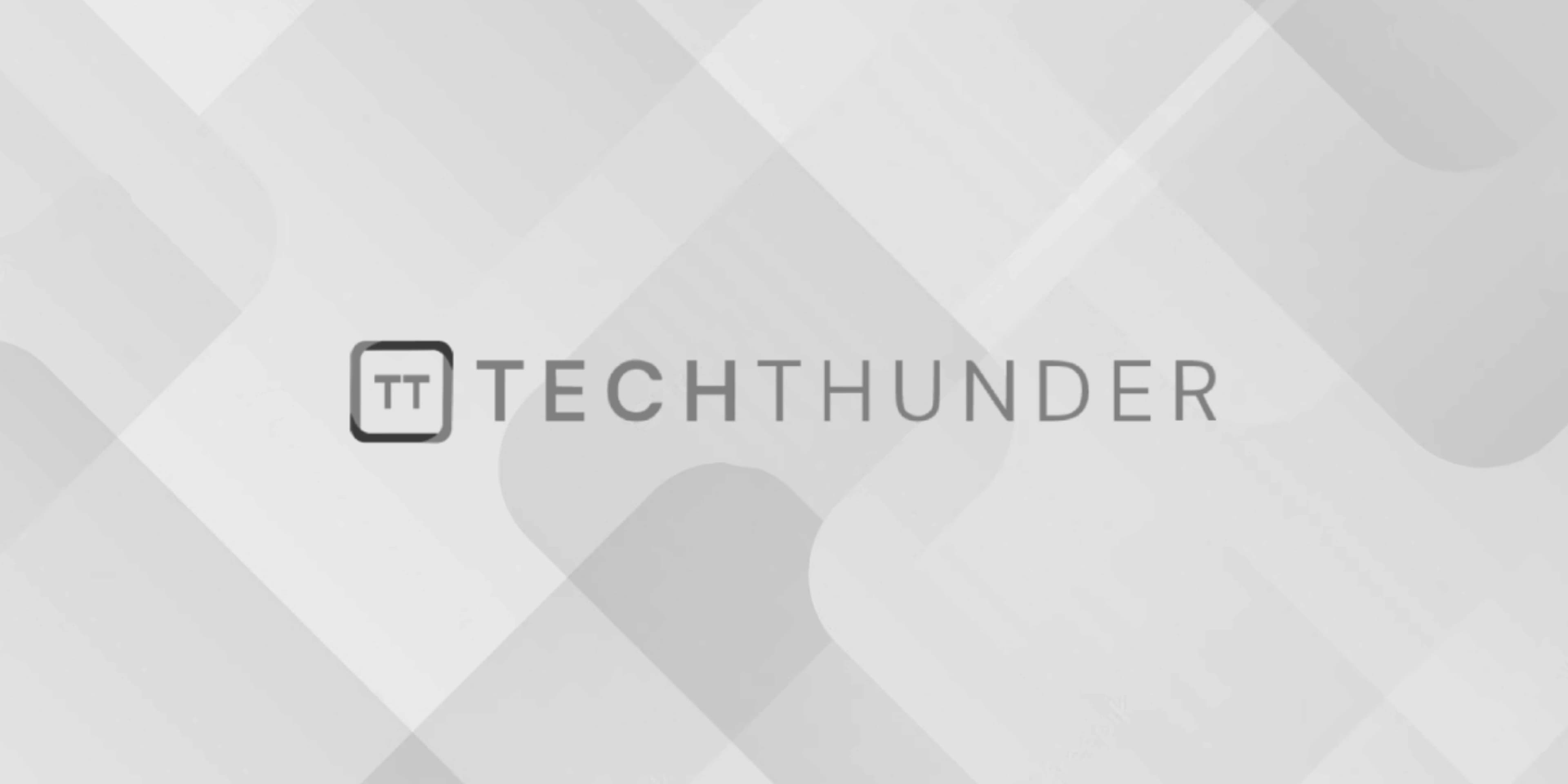
284 views
C++ Comments
The C++ comments are used to add explanatory notes or remarks within your code. Comments are ignored by the compiler and serve as documentation or explanations for the code’s readers, including other developers who may work on the code or your future self.
C++ supports two types of comments:
- Single-Line Comments: These comments begin with
//
and continue until the end of the line. Anything after//
on the same line is treated as a comment and is not compiled.
C++
// This is a single-line comment
int x = 42; // This is a comment at the end of a line
- Multi-Line Comments: These comments start with
/*
and end with*/
. Anything between these delimiters is treated as a comment, and you can span multiple lines within the comment.
C++
/*
This is a multi-line comment.
It can span multiple lines.
*/
Comments are essential for making your code more readable and maintaining it effectively. Here are some common use cases for comments:
- Explanatory Comments: Explain the purpose of a variable, function, or code block.
C++
int age; // The age of the user
- Documentation Comments: Provide documentation for functions, classes, or complex algorithms. These are often written using a special format like Doxygen or Javadoc.
C++
/**
* @brief Calculate the area of a rectangle.
* @param length The length of the rectangle.
* @param width The width of the rectangle.
* @return The area of the rectangle.
*/
double calculateRectangleArea(double length, double width);
- Temporary Comments: Leave temporary comments for debugging or making quick notes. It’s a good practice to remove or replace such comments when they are no longer needed.
C++
int x = 42; // TODO: Remove this line before production
- Commenting Out Code: Temporarily disable a block of code for testing or debugging purposes.
C++
/*
int result = someFunction();
// int result = anotherFunction(); // Commenting out this line
*/
- Legal Notices: Include licensing information and copyright notices in your code.
C++
/*
This code is distributed under the MIT License.
Copyright (c) 2023 John Doe.
*/
Good commenting practices can make your code more maintainable and help others understand your intentions and design decisions. However, it’s important not to over-comment; only include comments when they add value or clarity to the code.