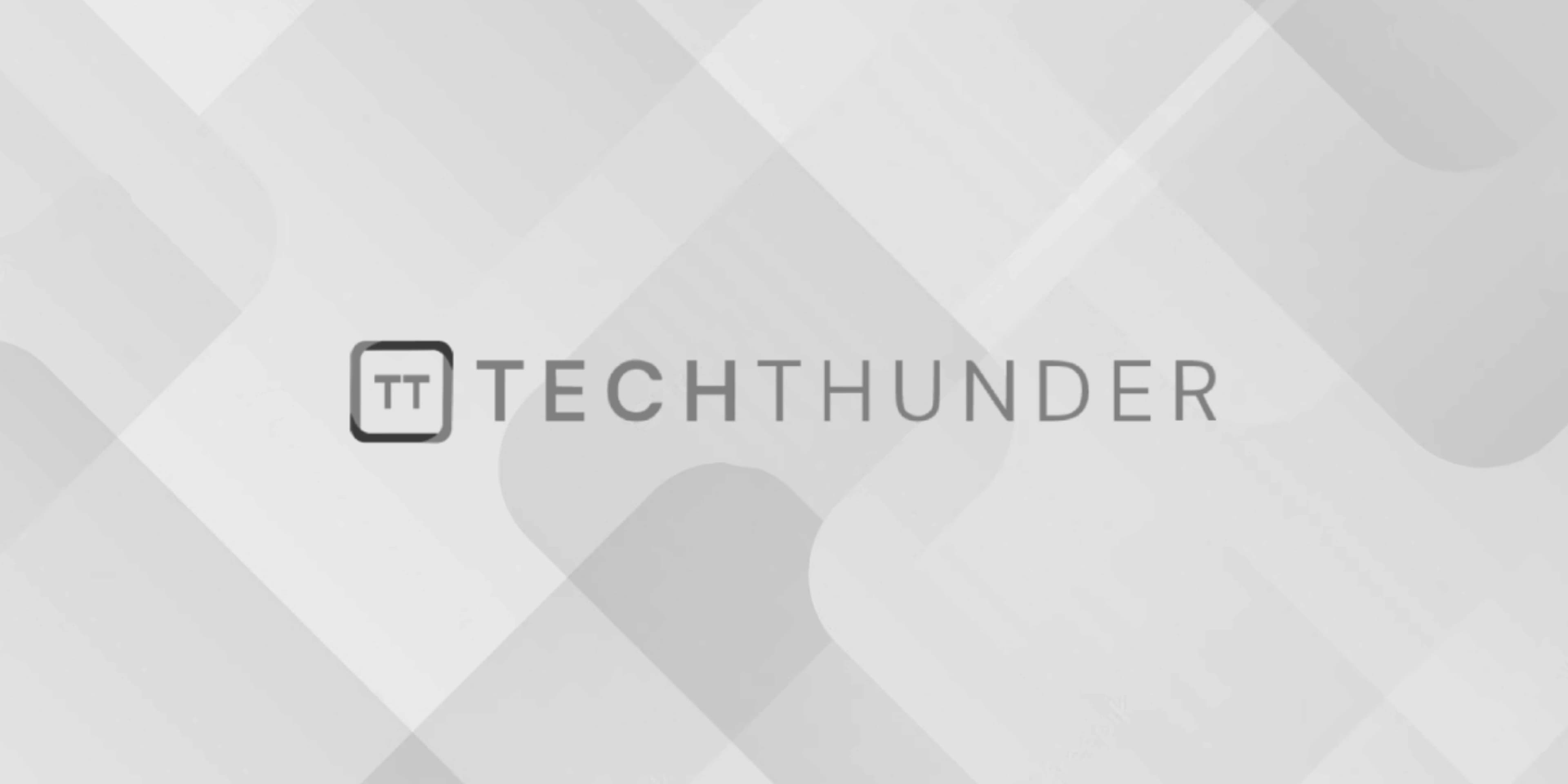
151 views
Print all Substrings of a String that has equal number of vowels and consonants in C++
To print all substrings of a string that have an equal number of vowels and consonants in C++, you can follow these steps:
- Create a function to check if a character is a vowel.
- Iterate through all possible substrings of the input string.
- For each substring, count the number of vowels and consonants.
- If the counts are equal, print the substring.
Here’s a C++ program to achieve this:
C++
#include <iostream>
#include <string>
// Function to check if a character is a vowel
bool isVowel(char ch) {
ch = tolower(ch); // Convert the character to lowercase for case-insensitive check
return (ch == 'a' || ch == 'e' || ch == 'i' || ch == 'o' || ch == 'u');
}
// Function to print substrings with an equal number of vowels and consonants
void printSubstringsWithEqualVowelsAndConsonants(const std::string& str) {
int n = str.length();
for (int i = 0; i < n; i++) {
int vowelCount = 0;
int consonantCount = 0;
for (int j = i; j < n; j++) {
char ch = str[j];
if (isVowel(ch)) {
vowelCount++;
} else if (isalpha(ch)) { // Check if the character is an alphabet (consonant)
consonantCount++;
}
if (vowelCount == consonantCount) {
std::cout << str.substr(i, j - i + 1) << std::endl;
}
}
}
}
int main() {
std::string inputString = "hello";
std::cout << "Substrings with an equal number of vowels and consonants:" << std::endl;
printSubstringsWithEqualVowelsAndConsonants(inputString);
return 0;
}
In this program:
- The
isVowel
function checks if a character is a vowel. It converts the character to lowercase to make the check case-insensitive. - The
printSubstringsWithEqualVowelsAndConsonants
function iterates through all possible substrings of the input string and counts the number of vowels and consonants in each substring. - If the counts are equal, it prints the substring.
- In the
main
function, you can change the value ofinputString
to test different input strings.
Keep in mind that this program assumes English vowels and consonants. You can modify the isVowel
function to suit different languages if needed.