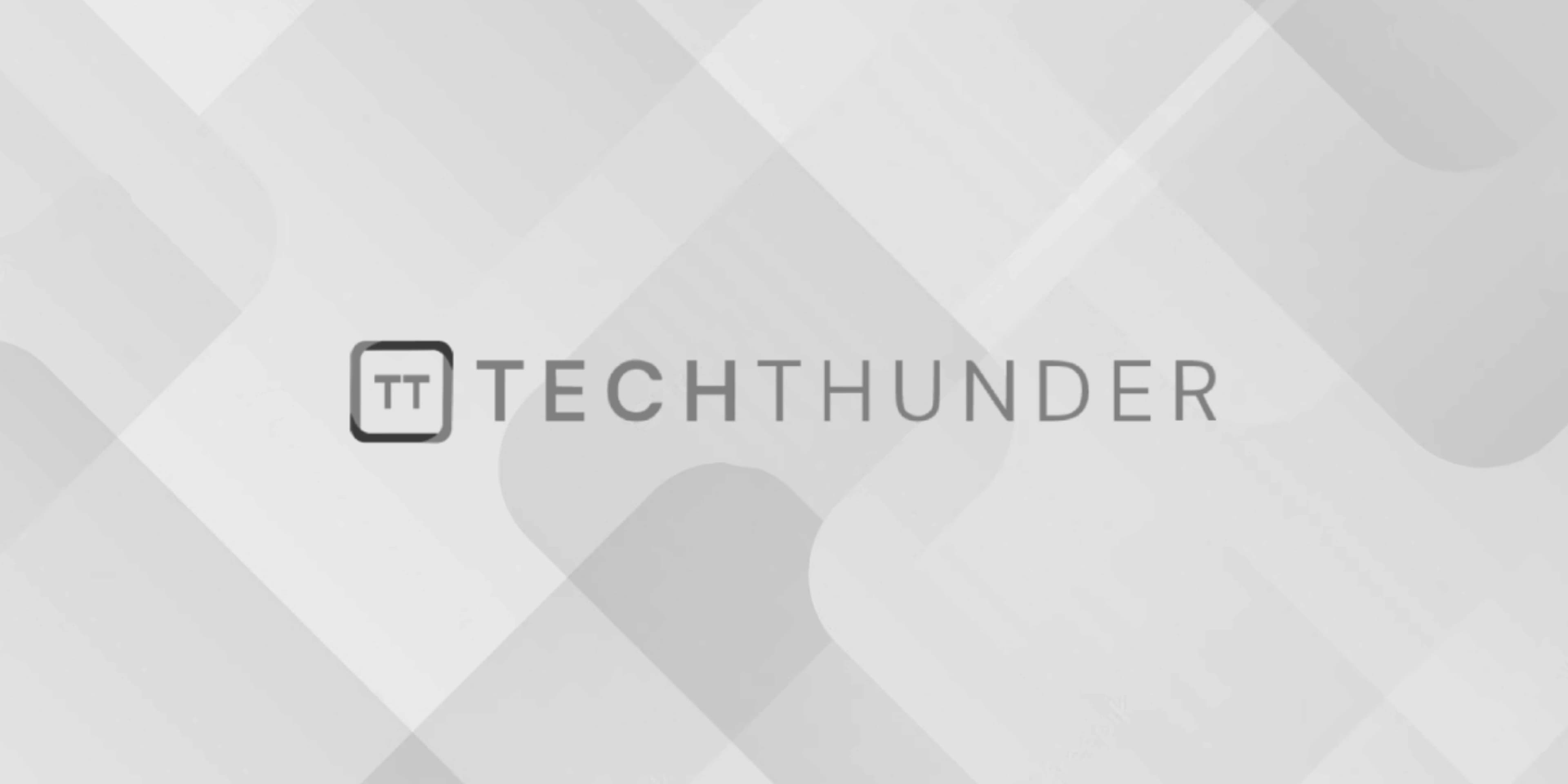
Fast input and output in C++
In competitive programming or other situations where input and output performance is critical, you can use techniques to achieve fast input and output in C++. The standard input/output streams (cin
and cout
) are convenient but may not be the fastest for large input or output. Here are some techniques to optimize input and output speed:
- Use
std::ios::sync_with_stdio(false)
: By default,cin
andcout
are synchronized with the C standard I/O functions, which can be slower than direct input/output. You can disable synchronization for a potential speed boost:
std::ios::sync_with_stdio(false);
Note that this should be called before any input/output operation.
- Use
cin.tie(nullptr)
: By default,cin
is tied tocout
, meaning thatcout
is flushed before reading fromcin
. You can untie them for better performance:
std::cin.tie(nullptr);
- Use
'\n'
instead ofendl
: Avoid usingendl
when printing tocout
because it flushes the output buffer. Instead, use'\n'
for line breaks:
std::cout << "Hello, World!\n";
- Use a custom input function: For reading large amounts of input data, using
cin
for each input operation can be slow. You can create a custom input function that reads data more efficiently. For example:
int readInt() {
int x;
std::cin >> x;
return x;
}
This function reads an integer using cin
and can be faster than direct cin
usage in certain situations.
- Use
printf
andscanf
for formatted output/input: If you prefer to use C-style I/O for better performance, you can useprintf
andscanf
for formatted output and input, respectively.
printf("Hello, World!\n");
int x;
scanf("%d", &x);
- Buffered Output: If you are performing a large number of output operations, consider using a custom output buffer to reduce the overhead of multiple
cout
calls.
Here’s an example of combining these techniques:
#include <iostream>
#include <cstdio>
int main() {
std::ios::sync_with_stdio(false);
std::cin.tie(nullptr);
// Faster input using scanf
int n;
scanf("%d", &n);
for (int i = 0; i < n; i++) {
int x;
scanf("%d", &x);
// Process x
}
// Faster output using printf
printf("Output: %d\n", 42);
return 0;
}
Keep in mind that the choice of optimization techniques depends on the specific requirements of your program and the input/output sizes. In most situations, the default I/O streams (cin
and cout
) are sufficient and easy to use, but for time-critical applications, these optimizations can make a noticeable difference in performance.