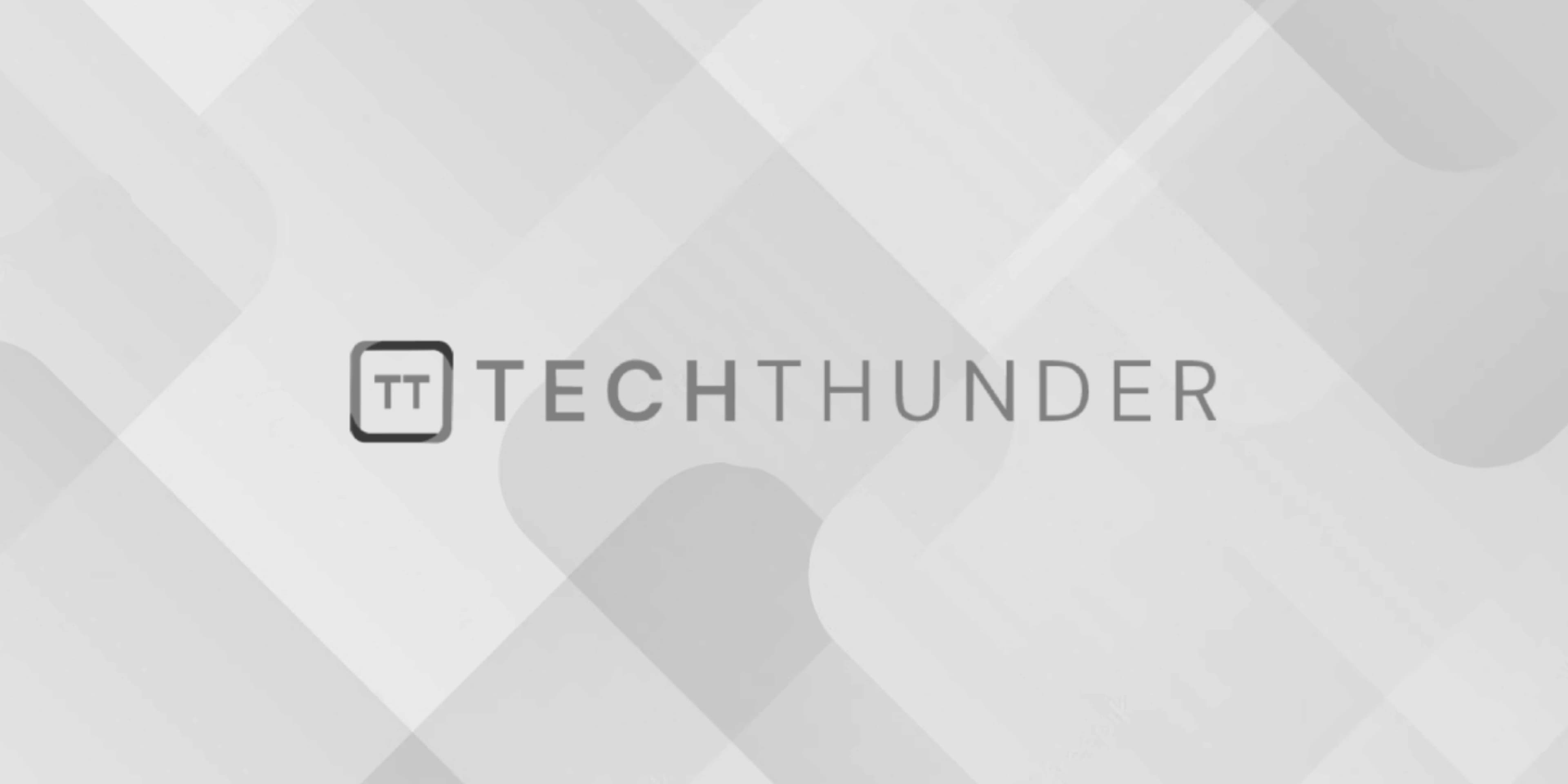
Foreach in C++ and JAVA
Both C++ and Java provide constructs for iterating over collections of data, but they use different syntax and mechanisms. Here’s a comparison of the foreach
or “enhanced for loop” constructs in both languages:
Java (Enhanced For Loop):
In Java, the enhanced for loop allows you to iterate over elements of an iterable (such as an array or a collection) without explicitly using an index. It provides a more concise and readable way to iterate through elements.
// Using enhanced for loop in Java
int[] numbers = {1, 2, 3, 4, 5};
for (int number : numbers) {
System.out.println(number);
}
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
for (String name : names) {
System.out.println(name);
}
In the Java enhanced for loop, you specify the type of elements you are iterating over, followed by a colon :
and the collection you want to iterate through. The loop variable (number
and name
in the examples) takes on each element of the collection in turn.
C++ (Range-based for Loop):
In C++, the range-based for loop was introduced in C++11 to provide a similar convenient way to iterate over elements of a container. It works with arrays, standard library containers (e.g., vectors, lists), and user-defined types that support the range-based for loop.
// Using range-based for loop in C++
int numbers[] = {1, 2, 3, 4, 5};
for (int number : numbers) {
std::cout << number << std::endl;
}
std::vector<std::string> names = {"Alice", "Bob", "Charlie"};
for (const std::string& name : names) {
std::cout << name << std::endl;
}
In C++, the range-based for loop iterates over elements of a container or array, and the loop variable (number
and name
in the examples) represents each element in sequence.
It’s important to note that while the syntax and usage of enhanced/foreach loops in Java and range-based for loops in C++ are similar, there are differences in how they handle elements and types, especially when it comes to value vs. reference semantics. In C++, you can use auto
to deduce the type of the loop variable to simplify the code further.