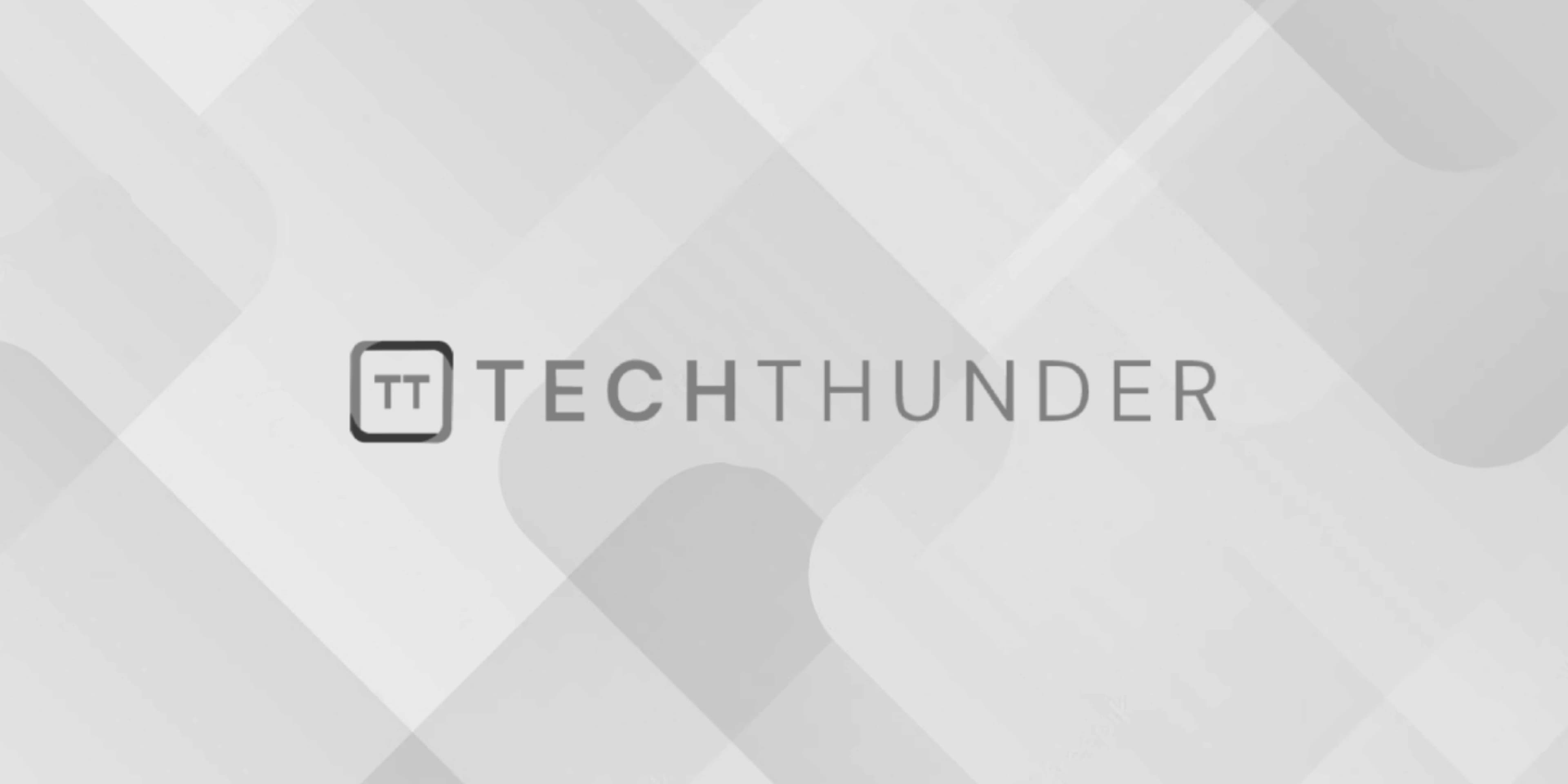
177 views
How many Children does a Binary Tree have in C++
The binary tree, each node can have at most two children: a left child and a right child. This property distinguishes a binary tree from other tree data structures, where nodes may have more than two children.
Here’s an example of a simple binary tree node structure in C++:
C++
struct TreeNode {
int data;
TreeNode* left;
TreeNode* right;
TreeNode(int value) : data(value), left(nullptr), right(nullptr) {}
};
In this example:
TreeNode
is a structure representing a node in a binary tree.- It has an integer
data
to store the value of the node. - It has two pointers
left
andright
, which point to the left child and right child nodes, respectively. - The constructor initializes the node with a given value and sets both children pointers to
nullptr
.
This structure represents a binary tree node where each node can have at most two children. Keep in mind that a binary tree can be empty (no nodes), have only a root node (with no children), or have many levels of nodes with left and right children.