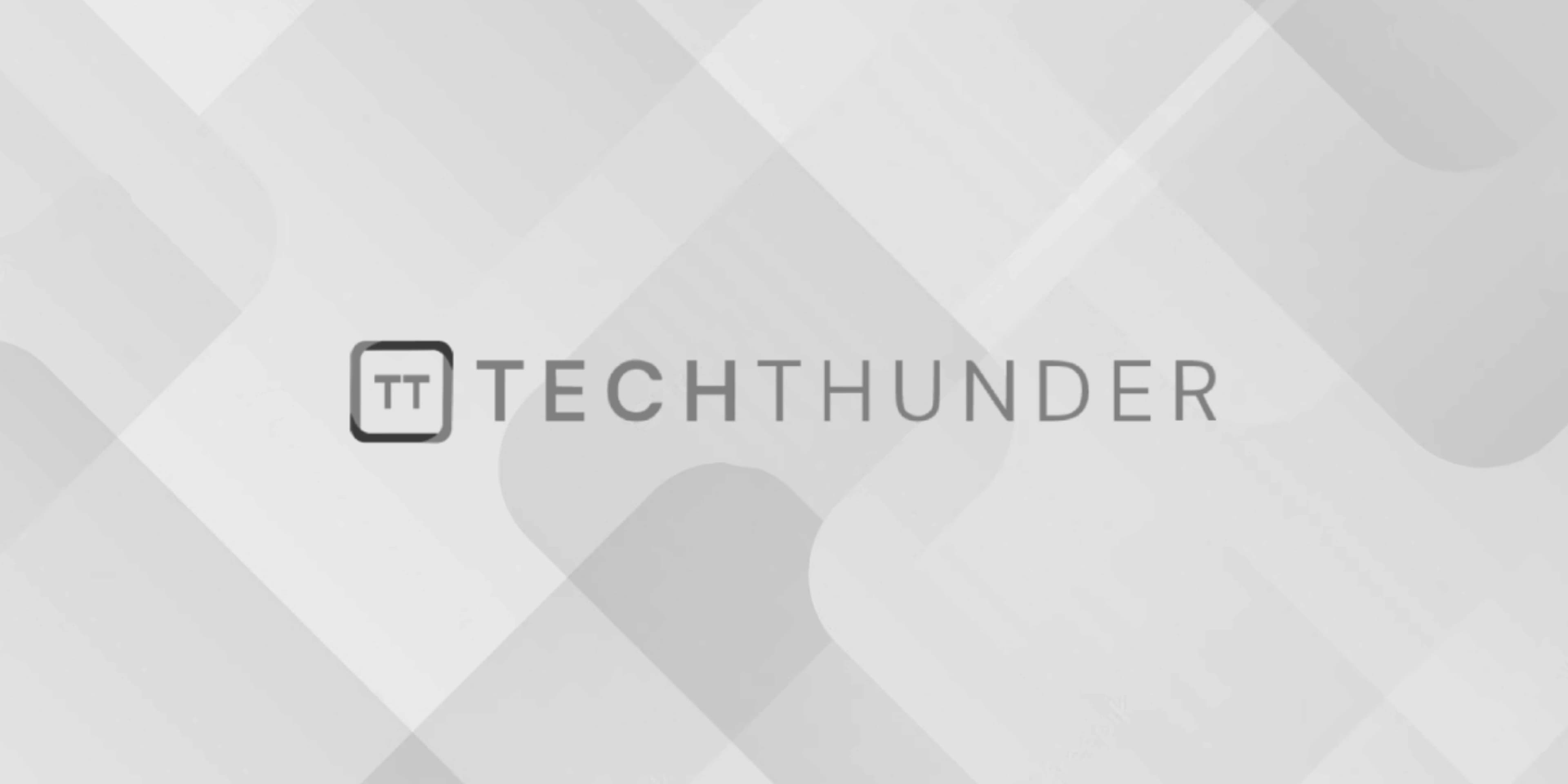
C++ References
The C++ reference is a variable that acts as an alias, allowing you to work with an existing variable using a different name. References provide a way to manipulate variables indirectly, and they have some important characteristics:
- Initialization: References must be initialized when declared, and once they are bound to a variable, they cannot be re-bound to another variable. This makes them different from pointers.
int x = 10;
int& ref = x; // 'ref' is a reference to 'x'
- No Null References: Unlike pointers, references cannot be null or uninitialized. They must always refer to a valid object.
- Readability: References can make code more readable, especially when used as function parameters or return values.
Here are some common use cases for references in C++:
1. Function Parameters and Return Values:
References are often used in function parameters and return values to avoid copying large objects and improve performance.
// Using a reference as a function parameter to avoid copying
void modifyValue(int& val) {
val *= 2;
}
int main() {
int num = 5;
modifyValue(num); // 'num' is modified directly
return 0;
}
// Using a reference as a function return value
int& getSomeValue() {
static int value = 42; // Static to keep it alive after the function exits
return value;
}
int main() {
int& ref = getSomeValue();
std::cout << ref << std::endl; // Outputs: 42
return 0;
}
2. Function Overloading and Operator Overloading:
References can be used to differentiate between overloaded functions or operators.
void printValue(const int& val) {
std::cout << "Value: " << val << std::endl;
}
void printValue(const double& val) {
std::cout << "Value (double): " << val << std::endl;
}
int main() {
int x = 10;
double y = 3.14;
printValue(x); // Calls the int version
printValue(y); // Calls the double version
return 0;
}
3. Avoiding Copies:
References are used to avoid copying objects, which can be particularly important when dealing with large data structures.
std::vector<int> createLargeVector() {
std::vector<int> vec(1000000, 42); // A large vector
return vec; // Without a reference, this would trigger a copy
}
int main() {
std::vector<int>& ref = createLargeVector(); // Avoids unnecessary copy
// ...
return 0;
}
Keep in mind that while references are powerful, they also come with some caveats. It’s crucial to be aware of the lifetime of the objects they refer to, as using a reference to an object that has gone out of scope leads to undefined behavior. Additionally, references are not suitable for all situations, and you should choose between references and pointers based on your specific requirements.