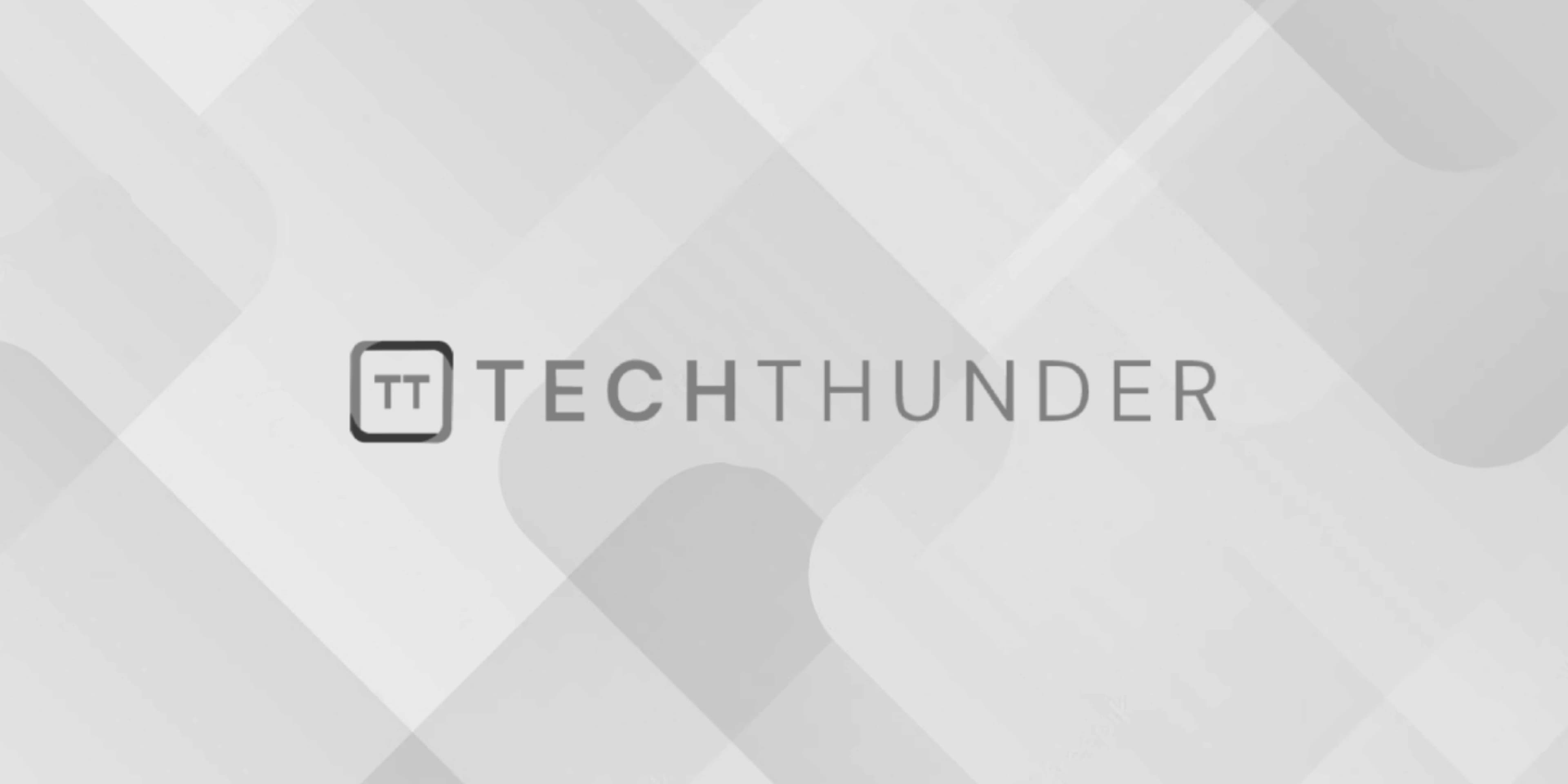
What is include iostream in C++
The #include <iostream>
is a preprocessor directive that tells the compiler to include the contents of the <iostream>
header file in your C++ program. This header file is a part of the C++ Standard Library and provides input and output functionality, allowing you to work with input and output streams, such as standard input (keyboard) and standard output (console).
The <iostream>
header file includes definitions for the following standard C++ stream objects:
std::cin
: This is the standard input stream object, which is used for reading input from the user or external sources like files.std::cout
: This is the standard output stream object, which is used for writing output to the console.std::cerr
: This is the standard error stream object, which is typically used for printing error messages to the console.std::endl
: This is a special value that represents the end of a line. It’s often used to move to the next line when writing output.
By including <iostream>
, you gain access to these stream objects and the various input and output functions provided by the C++ Standard Library, such as std::cin >> variable
for input and std::cout << "Hello, World!"
for output.
Here’s a simple example of using <iostream>
to print a message to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this code, <iostream>
is included, and std::cout
is used to print “Hello, World!” followed by a newline to the console.