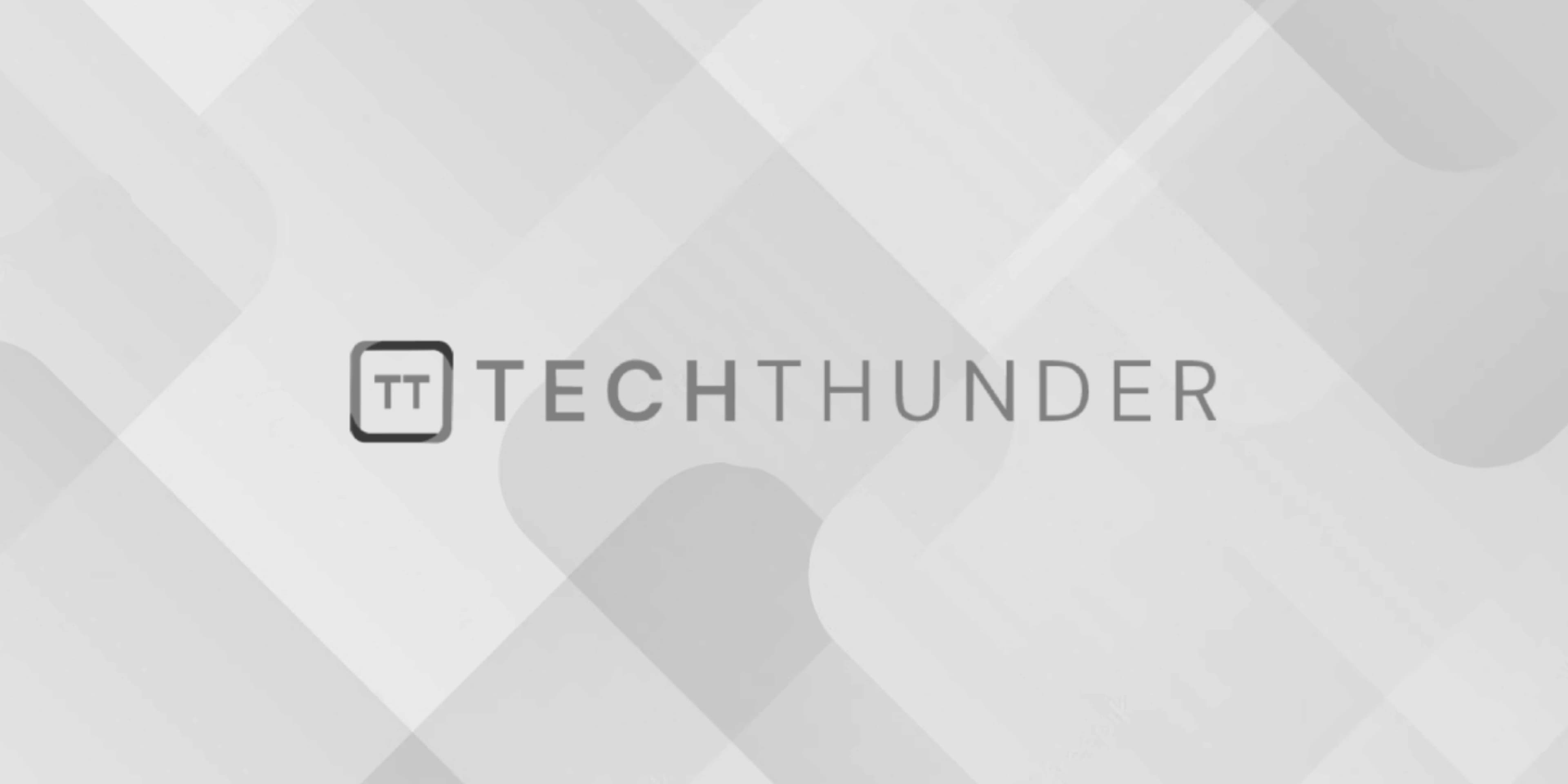
University Management System in C++
Creating a complete University Management System in C++ is a complex task that involves multiple functionalities such as managing students, courses, faculty, grades, and more. It’s beyond the scope of a single response to provide a full-fledged implementation. However, I can give you an outline and some basic code snippets to get started. You can expand and refine the system based on your specific requirements.
University Management System Outline:
- Student Class:
- Attributes: Student ID, Name, Age, Courses Enrolled, Grades, etc.
- Methods: Enroll Courses, View Courses, View Grades, Update Profile, etc.
- Course Class:
- Attributes: Course Code, Title, Instructor, Schedule, etc.
- Methods: View Course Details, Modify Schedule, Assign Instructor, etc.
- Faculty Class:
- Attributes: Faculty ID, Name, Courses Teaching, Salary, etc.
- Methods: View Courses, View Salary, Update Profile, Assign Courses, etc.
- Grade Class:
- Attributes: Student ID, Course Code, Grade, etc.
- Methods: Assign Grade, View Grade Report, Calculate GPA, etc.
- University Class:
- Attributes: List of Students, List of Courses, List of Faculty, etc.
- Methods: Add Student, Remove Student, Add Course, Remove Course, Assign Faculty, Generate Reports, etc.
- Menu System:
- Implement a menu-based system to interact with the user. This can be done using loops and switch-case statements.
Example Code Snippets:
Here are some basic code snippets to give you an idea of how to structure your program:
// Student Class
class Student {
private:
int studentID;
string name;
// Add more attributes as needed
public:
// Constructor, getters, setters, and methods
};
// Course Class
class Course {
private:
string courseCode;
string title;
// Add more attributes as needed
public:
// Constructor, getters, setters, and methods
};
// Faculty Class
class Faculty {
private:
int facultyID;
string name;
// Add more attributes as needed
public:
// Constructor, getters, setters, and methods
};
// Grade Class
class Grade {
private:
int studentID;
string courseCode;
char grade;
// Add more attributes as needed
public:
// Constructor, getters, setters, and methods
};
// University Class
class University {
private:
vector<Student> students;
vector<Course> courses;
vector<Faculty> faculty;
vector<Grade> grades;
public:
// Methods to manage students, courses, faculty, grades, etc.
};
// Main function with menu system
int main() {
University university;
int choice;
do {
// Display menu options and get user input
// Based on user input, call appropriate functions in the University class
} while (choice != 0);
return 0;
}
Please note that this is a simplified outline and code snippets. Depending on the specific requirements of your University Management System, you may need to add more functionalities and handle edge cases. Additionally, you may want to implement data persistence (e.g., file storage or databases) for a real-world application.