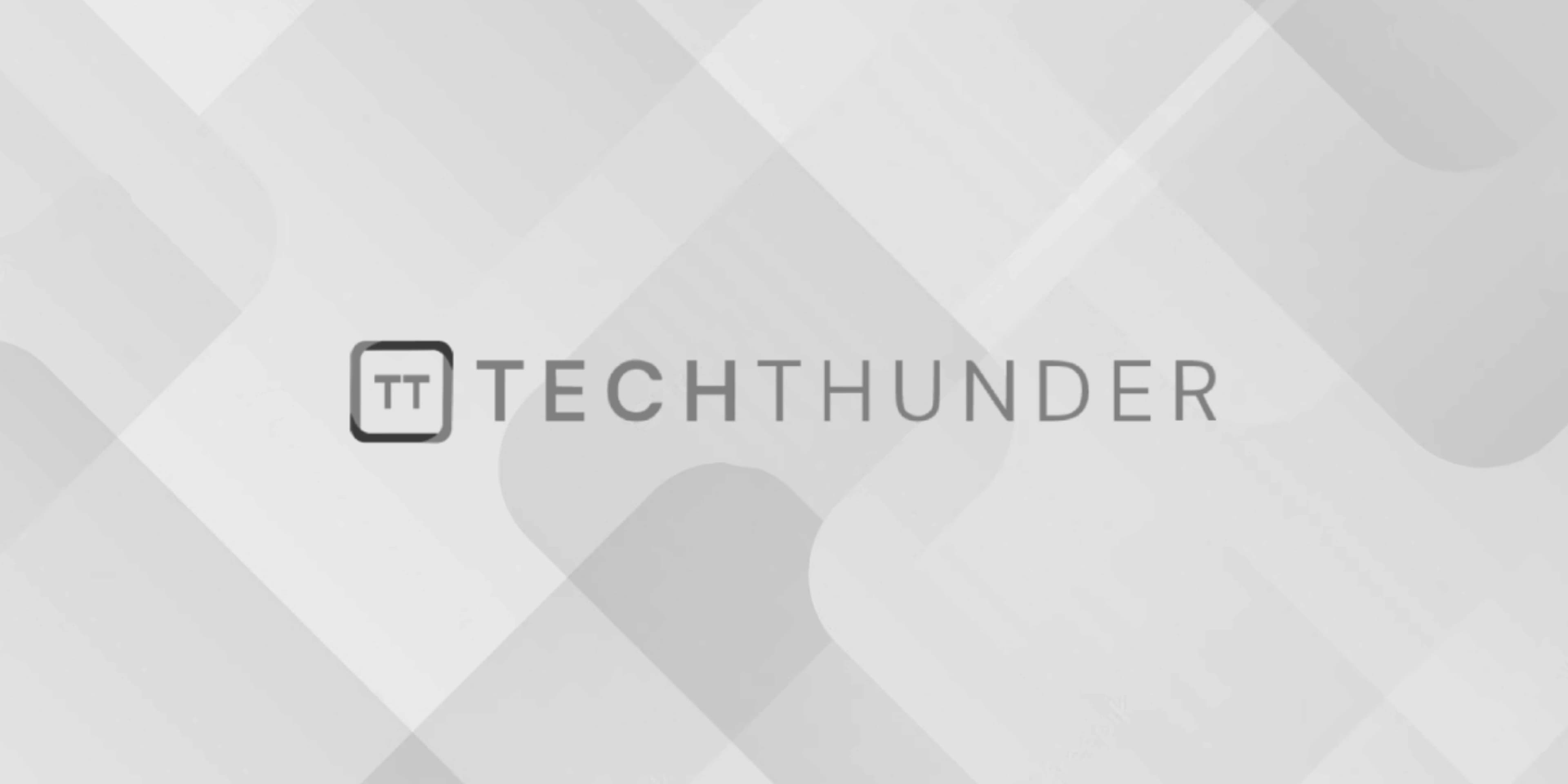
275 views
Adding of Two Numbers in C++
Adding two numbers in C++ is straightforward. You can use the +
operator to perform the addition. Here’s a simple C++ program to add two numbers:
C++
#include <iostream>
int main() {
// Declare variables to store the numbers
double num1, num2;
// Prompt the user to enter the first number
std::cout << "Enter the first number: ";
std::cin >> num1;
// Prompt the user to enter the second number
std::cout << "Enter the second number: ";
std::cin >> num2;
// Perform the addition
double sum = num1 + num2;
// Display the result
std::cout << "Sum: " << sum << std::endl;
return 0;
}
In this program:
- We declare two
double
variables,num1
andnum2
, to store the input numbers. - We use
std::cout
to prompt the user to enter both numbers. - We use
std::cin
to read the input numbers from the user. - We add the two numbers using the
+
operator and store the result in thesum
variable. - Finally, we use
std::cout
to display the sum to the user.
When you run this program, it will ask you to enter two numbers, and then it will calculate and display their sum.