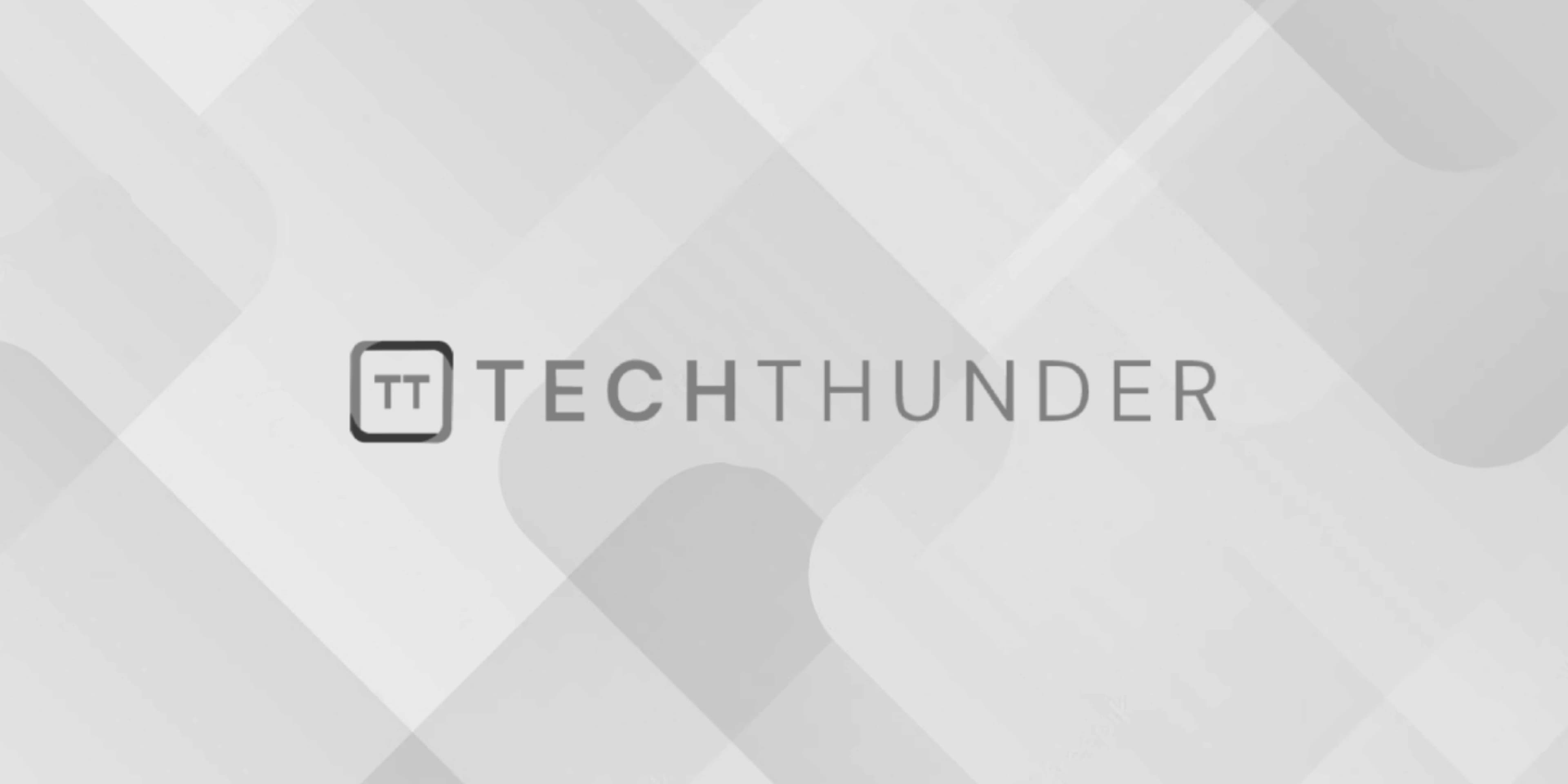
Convert Camel Case String in Snake Case in C++
To convert a Camel Case string to Snake Case in C++, you can write a function that iterates through the characters of the input string and adds underscores (_) before uppercase letters, while also converting all characters to lowercase. Here’s a sample C++ code to achieve this conversion:
#include <iostream>
#include <string>
std::string camelToSnakeCase(const std::string& input) {
std::string result;
bool lastCharWasLower = false;
for (char c : input) {
if (std::isupper(c)) {
if (lastCharWasLower) {
result.push_back('_');
}
result.push_back(std::tolower(c));
} else {
result.push_back(c);
}
lastCharWasLower = std::islower(c);
}
return result;
}
int main() {
std::string camelCaseString = "myCamelCaseString";
std::string snakeCaseString = camelToSnakeCase(camelCaseString);
std::cout << "Camel Case: " << camelCaseString << std::endl;
std::cout << "Snake Case: " << snakeCaseString << std::endl;
return 0;
}
This code defines a camelToSnakeCase
function that takes an input string in Camel Case and converts it to Snake Case. It iterates through the characters of the input string, adding underscores before uppercase letters (if the last character was not already uppercase) and converting all characters to lowercase.
When you run this code with the input string “myCamelCaseString,” it will output:
Camel Case: myCamelCaseString
Snake Case: my_camel_case_string
You can use the camelToSnakeCase
function to convert Camel Case strings to Snake Case in your C++ program.