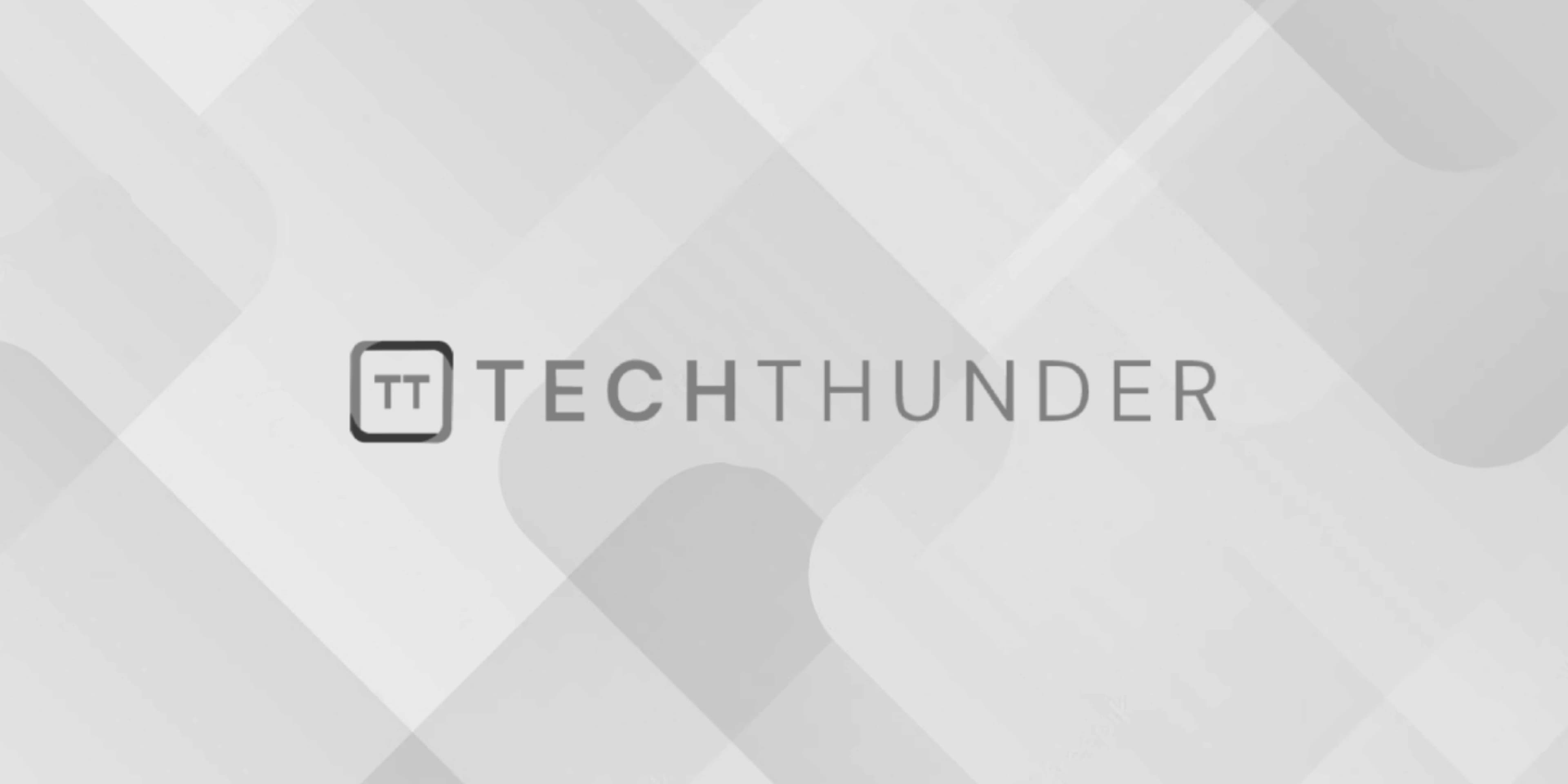
Bitmask in C++
The C++ bitmask is a technique used to manipulate individual bits within an integer or a group of integers. It involves setting, clearing, toggling, and checking specific bits to represent and work with certain attributes or flags efficiently. Bitmasks are commonly used to implement various algorithms, settings, and flags in a compact and memory-efficient way.
Here’s a basic overview of how bitmasks work in C++:
- Setting a Bit: To set a specific bit in an integer, you can use the bitwise OR (
|
) operator with the bitmask. The bitmask has the desired bit set to 1, while the other bits are set to 0.
int bitmask = 1 << position; // Shift 1 to the desired position
integer |= bitmask; // Set the bit
- Clearing a Bit: To clear a specific bit in an integer, you can use the bitwise AND (
&
) operator with a bitmask that has the desired bit set to 0 and all other bits set to 1.
int bitmask = ~(1 << position); // Invert the bitmask
integer &= bitmask; // Clear the bit
- Toggling a Bit: To toggle (flip) a specific bit in an integer, you can use the bitwise XOR (
^
) operator with a bitmask that has the desired bit set to 1 and all other bits set to 0.
int bitmask = 1 << position; // Shift 1 to the desired position
integer ^= bitmask; // Toggle the bit
- Checking a Bit: To check the value of a specific bit in an integer, you can use the bitwise AND (
&
) operator with a bitmask.
int bitmask = 1 << position; // Shift 1 to the desired position
bool isSet = (integer & bitmask) != 0; // Check the bit
Here’s a simple example using bitmasks to represent and manipulate flags:
#include <iostream>
const int FLAG_A = 1 << 0; // Bit 0 represents flag A
const int FLAG_B = 1 << 1; // Bit 1 represents flag B
const int FLAG_C = 1 << 2; // Bit 2 represents flag C
int main() {
int flags = 0; // Start with no flags set
flags |= FLAG_A; // Set flag A
flags |= FLAG_B; // Set flag B
if ((flags & FLAG_A) != 0) {
std::cout << "Flag A is set." << std::endl;
}
if ((flags & FLAG_B) != 0) {
std::cout << "Flag B is set." << std::endl;
}
if ((flags & FLAG_C) != 0) {
std::cout << "Flag C is set." << std::endl;
} else {
std::cout << "Flag C is not set." << std::endl;
}
flags ^= FLAG_A; // Toggle flag A
if ((flags & FLAG_A) == 0) {
std::cout << "Flag A is not set after toggling." << std::endl;
}
return 0;
}
Bitmasks are particularly useful when you need to store and manipulate multiple boolean flags compactly within an integer or a group of integers. They offer improved memory efficiency and faster operations compared to using separate boolean variables for each flag.