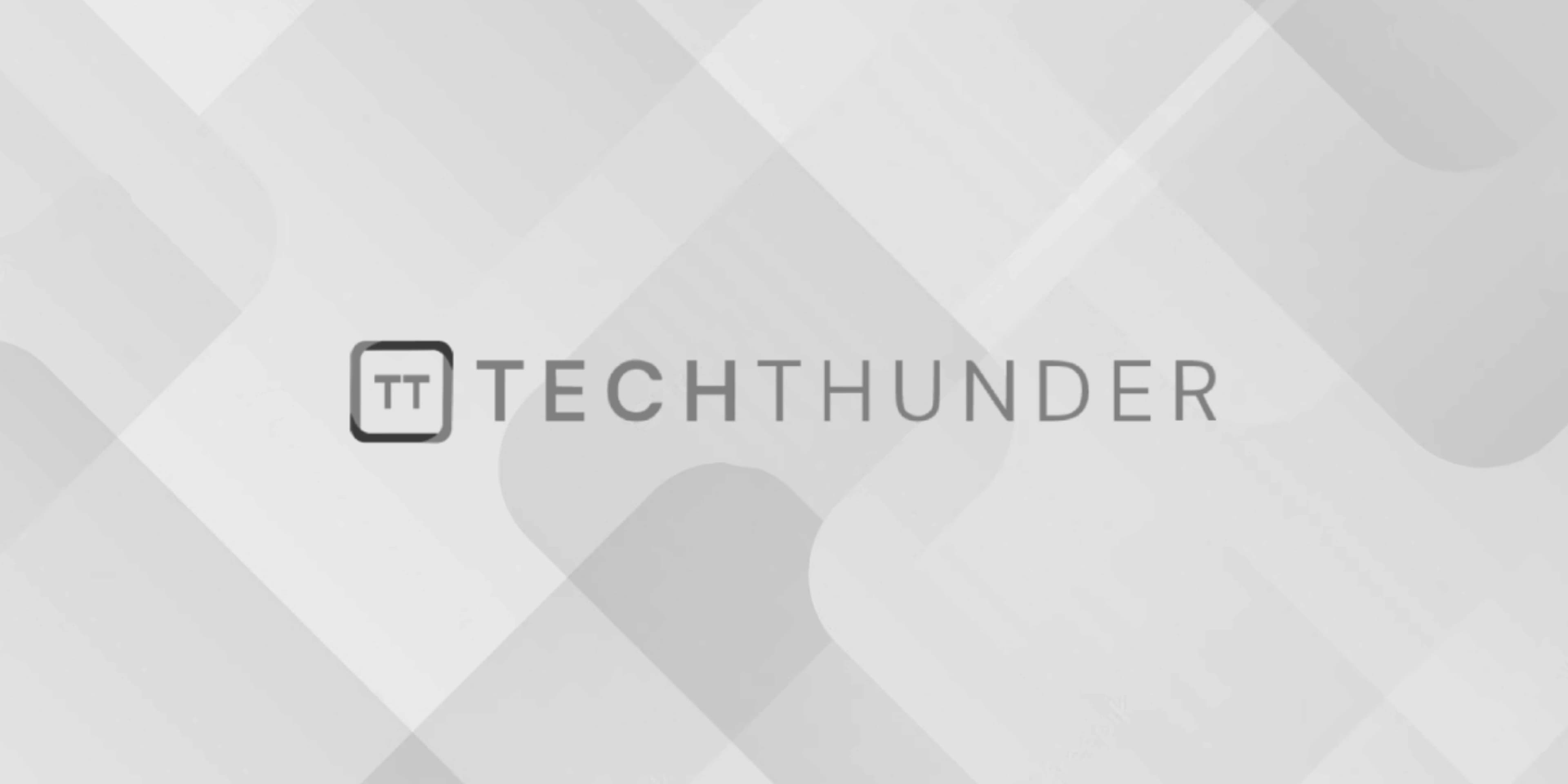
Structure vs Class in C++
The C++ has both structures (struct
) and classes (class
) are used to define user-defined data types that can hold data members (variables) and member functions (methods). They are used for encapsulation and organization of data within a program. While both structures and classes have similar features, there are some key differences in their default access control and intended use:
- Default Access Control:
- Structure (
struct
): The default access control for members of astruct
is public. This means that all data members are accessible from outside the structure without any restrictions. - Class (
class
): The default access control for members of aclass
is private. This means that by default, data members are not accessible from outside the class, and you need member functions (methods) to access or modify them.
- Intended Use:
- Structure (
struct
): Traditionally, structures were primarily used for grouping related data members together. They were considered more suitable for plain data structures without encapsulation or complex member functions. However, in modern C++, structures can have member functions and methods, and they can be used similarly to classes. - Class (
class
): Classes were originally introduced in C++ to provide object-oriented programming features, including data encapsulation and abstraction. They are more commonly used for defining objects that have both data members and methods to manipulate those members. Classes are suitable for creating well-encapsulated, reusable, and extensible code.
- Access Control Modifiers: Both structures and classes can use access control modifiers like
public
,private
, andprotected
to control the visibility and accessibility of their members. This allows you to specify which parts of your structure or class should be accessible to the outside world and which should be kept private for internal use.
Here’s an example illustrating the differences between a struct
and a class
:
// Example using a struct
struct PointStruct {
int x; // Default access: public
int y; // Default access: public
};
// Example using a class
class PointClass {
public: // Access specified explicitly
int x; // Access: public
int y; // Access: public
};
In both cases, you can create objects of PointStruct
and PointClass
and access their members, but with a struct
, the members are public by default, while with a class
, you need to explicitly specify the access control.
PointStruct s;
s.x = 10; // Accessing members directly is fine because they are public.
PointClass c;
c.x = 20; // This is fine because the members are public and access was specified as public.
In modern C++, the choice between using a struct
or a class
often depends on the design of your code and how you want to encapsulate and control access to your data members and member functions. Many C++ programmers use class
for most scenarios due to its additional access control and encapsulation features, while struct
may be used for simple data structures or when compatibility with C code is required. However, these are general guidelines, and the choice ultimately depends on the specific needs of your program.