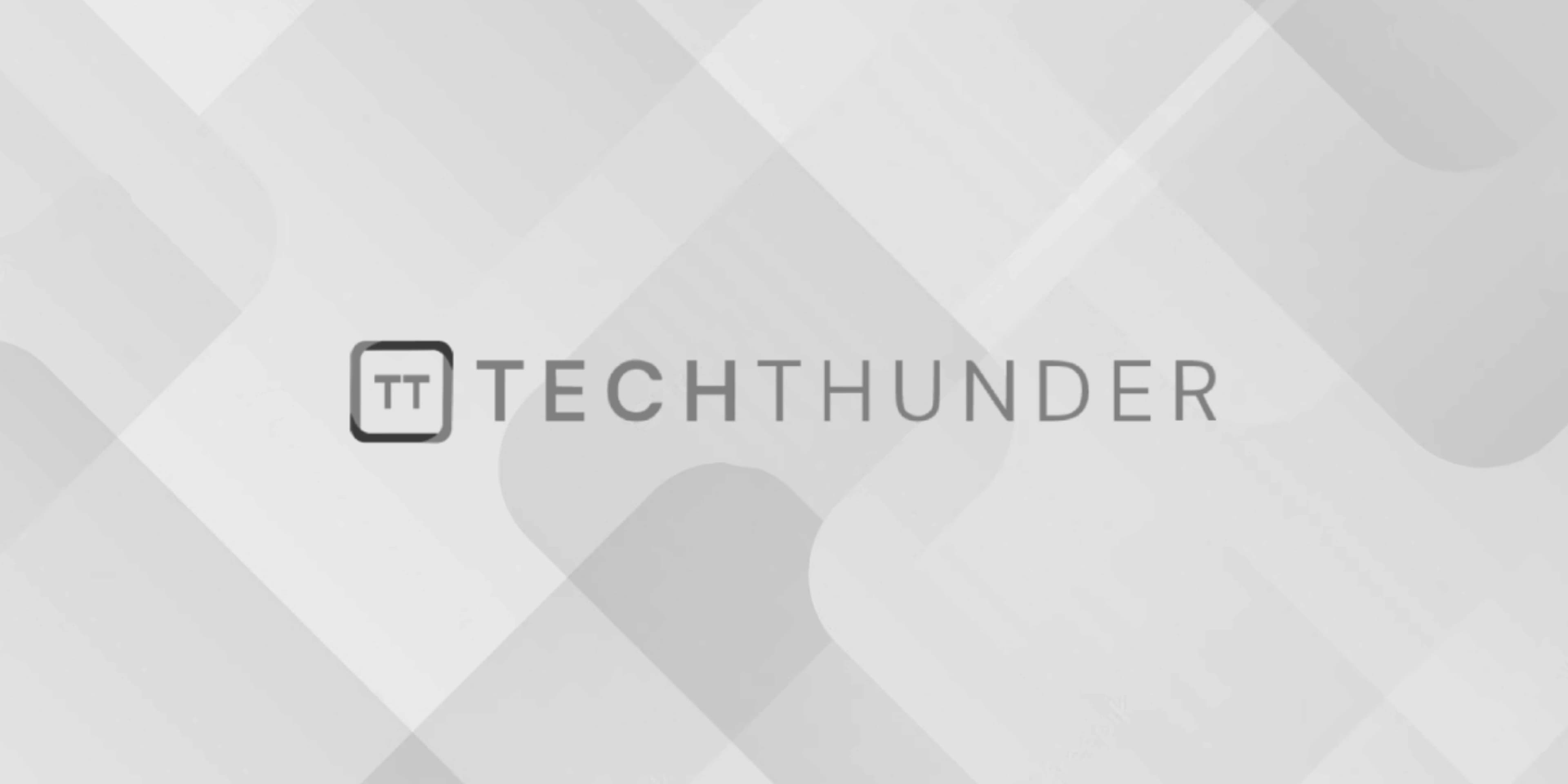
249 views
Smart pointers in C++
Smart pointers in C++ are a type of C++ object that acts as a wrapper around a raw (or regular) C++ pointer. They provide automatic memory management and help prevent common issues like memory leaks and dangling pointers. C++ has three types of smart pointers:
std::shared_ptr
: Shared pointers allow multiple pointers to share ownership of a dynamically allocated object. They use reference counting to keep track of the number of shared pointers that own the object, and the object is automatically deallocated when the last shared pointer that owns it is destroyed or reset.
C++
#include <iostream>
#include <memory>
int main() {
std::shared_ptr<int> sharedPtr = std::make_shared<int>(42);
std::shared_ptr<int> anotherPtr = sharedPtr;
std::cout << *sharedPtr << std::endl; // Output: 42
std::cout << *anotherPtr << std::endl; // Output: 42
return 0;
}
std::unique_ptr
: Unique pointers represent exclusive ownership of a dynamically allocated object. They cannot be copied but can be moved, ensuring that there is only one owner of the object. When the unique pointer goes out of scope, the object is automatically deallocated.
C++
#include <iostream>
#include <memory>
int main() {
std::unique_ptr<int> uniquePtr = std::make_unique<int>(42);
std::cout << *uniquePtr << std::endl; // Output: 42
return 0; // uniquePtr automatically deallocates the memory
}
std::weak_ptr
: Weak pointers are used in conjunction with shared pointers to break circular references. They do not increase the reference count of the object they point to. They can be used to check if the object they point to still exists or to create shared pointers temporarily.
C++
#include <iostream>
#include <memory>
int main() {
std::shared_ptr<int> sharedPtr = std::make_shared<int>(42);
std::weak_ptr<int> weakPtr = sharedPtr;
if (auto lockedSharedPtr = weakPtr.lock()) {
std::cout << *lockedSharedPtr << std::endl; // Output: 42
} else {
std::cout << "The object no longer exists." << std::endl;
}
return 0;
}
Smart pointers help simplify memory management in C++ by automatically handling the allocation and deallocation of objects, preventing memory leaks, and making code safer and more readable. It’s generally recommended to use smart pointers whenever possible to manage dynamically allocated objects.