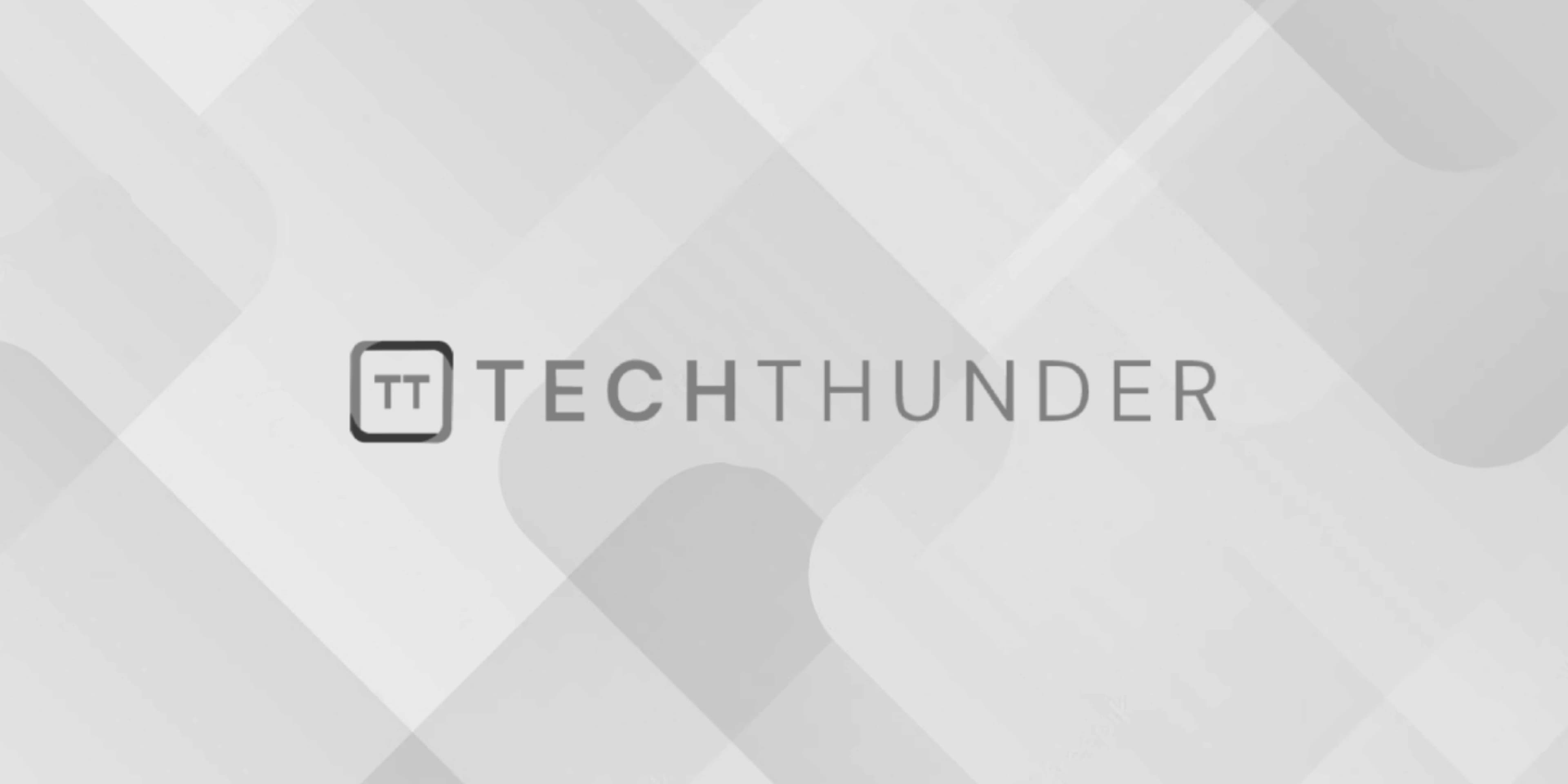
Actual Parameter and Formal Parameter in C++
The C++ actual parameters and formal parameters are terms used to distinguish between the arguments passed to a function (actual parameters) and the parameters declared in the function’s definition (formal parameters). These terms are essential in understanding how function arguments are passed and processed.
Here’s a breakdown of the concepts:
- Formal Parameters (or Formal Arguments):
- Formal parameters are the parameters declared in a function’s parameter list within its definition.
- They act as placeholders for the values that will be passed to the function when it is called.
- Formal parameters are also referred to as formal arguments or function parameters.
- These parameters have names and data types specified in the function’s signature.
void exampleFunction(int a, double b) {
// 'a' and 'b' are formal parameters
// They act as placeholders for the actual parameters passed when the function is called
}
- Actual Parameters (or Actual Arguments):
- Actual parameters are the values or expressions provided in a function call when invoking the function.
- They correspond to the formal parameters declared in the function’s definition.
- Actual parameters are also referred to as actual arguments or function arguments.
- These values or expressions are passed to the function to be used within the function’s body.
int x = 5;
double y = 3.14;
exampleFunction(x, y); // 'x' and 'y' are actual parameters
In the example above, int a
and double b
in the exampleFunction
function are formal parameters. When the function is called with x
and y
as arguments, x
corresponds to int a
(actual parameter for a
), and y
corresponds to double b
(actual parameter for b
).
The purpose of separating formal and actual parameters is to allow functions to work with data passed from the calling code dynamically. It allows you to reuse the same function with different input values without having to rewrite the function’s code. Understanding these concepts is fundamental when working with functions and function calls in C++.